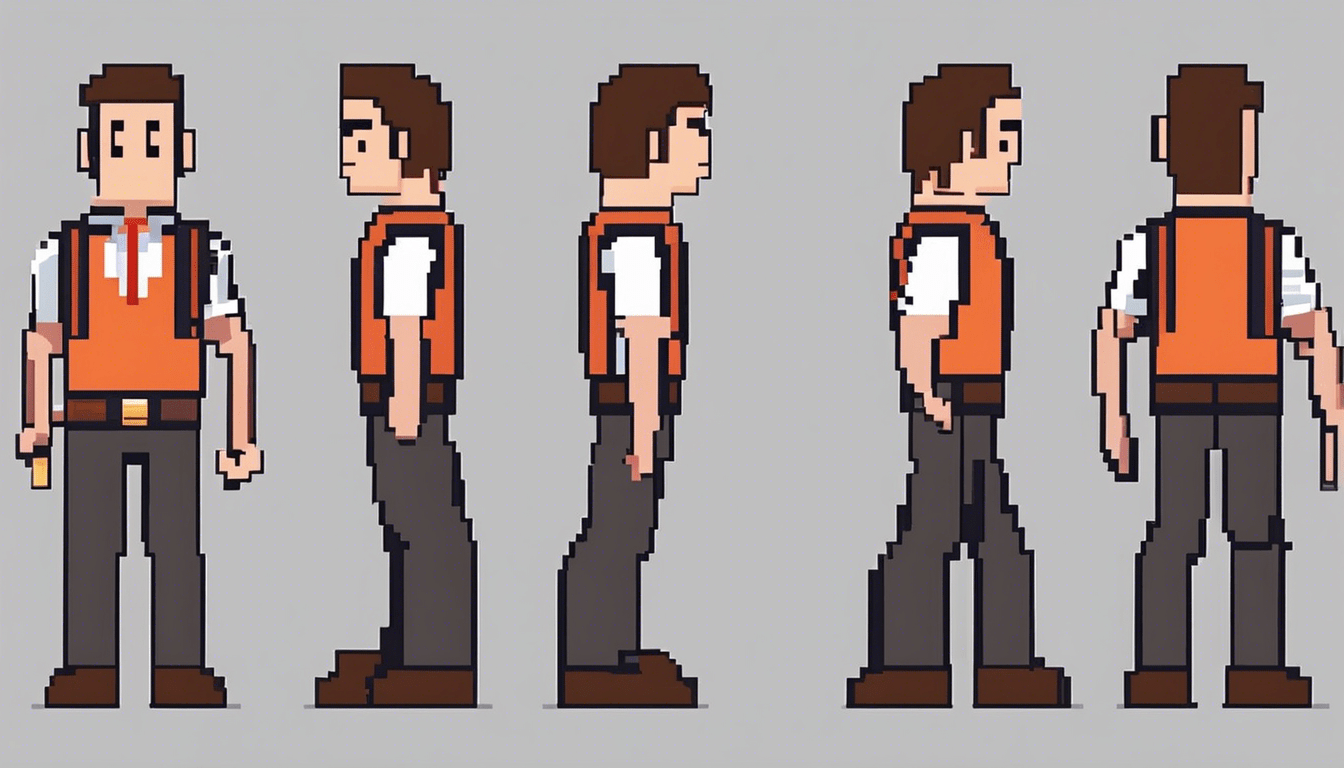
Basic Python Operators: Arithmetic and Logical
When you begin learning Python or any coding language, understanding the basic operators is important. Operators are symbols in Python that carry out arithmetic or logical computation. They function like the plus (+) or minus (-) signs in math. This article will take you through the arithmetic and logical operators in Python, one by one.
First, let’s start with Arithmetic operators in Python. These are used with numerical values to perform common mathematical operations:
The arithmetic operators include: addition (+), subtraction (-), multiplication (*), division (/), floor division (//), remainder (%), and exponential (**).
Addition (+) is used to add two numbers:
# Addition x = 5 y = 10 print(x + y) # Output: 15
Subtraction (-) is used to subtract one value from another:
# Subtraction x = 10 y = 5 print(x - y) # Output: 5
Multiplication (*) is used to multiply two values:
# Multiplication x = 5 y = 10 print(x * y) # Output: 50
Division (/) is used to divide one value by another:
# Division x = 10 y = 5 print(x / y) # Output: 2
Floor Division (//) is used to divide one value by another, rounding down to the nearest whole number:
# Floor Division x = 10 y = 3 print(x // y) # Output: 3
Remainder (%) is used to divide one value by another, and then returns the remainder:
# Modulo x = 10 y = 3 print(x % y) # Output: 1
Exponential (**) is used to raise the first number to the power of the second:
# Exponential x = 2 y = 3 print(x ** y) # Output: 8
Now that we’ve covered the basic arithmetic operators, let’s move on to the Logical operators. In Python, logical operators are used to combine conditional statements. They include ‘and’, ‘or’ and ‘not’ operators.
And operator returns True if both conditions are true. However, if one condition is false, it will return False.
# And Operator x = 5 print(x > 3 and x
Or operator returns True if at least one of the conditions is true. It will only return False if all conditions are false.
# Or Operator x = 5 print(x > 3 or x > 10) # Returns True because at least one condition, 5 is greater than 3, is true