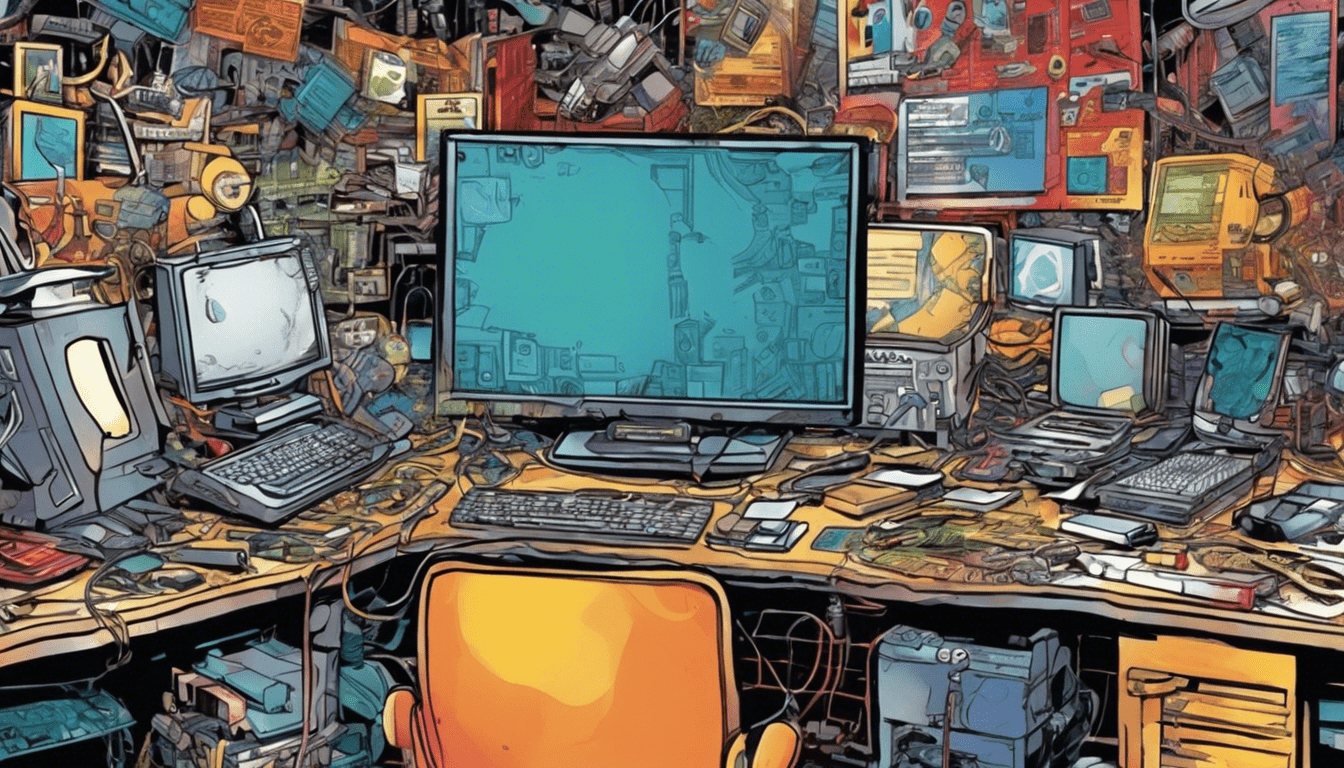
Java and API Design: Best Practices
API design is a critical aspect of software development, especially in the contemporary era where services are increasingly interconnected through APIs. Java, being one of the most popular programming languages, has a plethora of libraries and frameworks that help in designing and implementing APIs. In this article, we will focus on some best practices to keep in mind while designing APIs in Java.
1. Keep it Simple
Simplicity is key when it comes to API design. The API should be easy to understand and use for the developers who will be consuming it. This means naming your endpoints in a clear and concise manner, keeping the number of endpoints to a minimum, and using straightforward request and response models.
2. Use HTTP methods correctly
In RESTful APIs, HTTP methods have specific meanings. GET is used for retrieving data, POST for creating new resources, PUT for updating existing resources, and DELETE for removing resources. It’s important to stick to these conventions so that your API is intuitive for developers to use.
@RequestMapping(method = RequestMethod.GET) public ResponseEntity> getAllUsers() { // implementation } @RequestMapping(method = RequestMethod.POST) public ResponseEntity createUser(@RequestBody User user) { // implementation }
3. Use Proper Status Codes
HTTP status codes are an essential part of API design. They provide immediate feedback to the client about the result of their request. For example, 200 OK for a successful request, 400 Bad Request for an invalid request, 404 Not Found when a resource doesn’t exist, and 500 Internal Server Error when something goes wrong on the server. Using the correct status code is essential for good API design.
4. Version Your API
As your API evolves, you’ll need to make changes that may not be backward compatible. To handle this, it is best practice to version your API from the start. This can be done in the URL path or in the request header.
@RequestMapping(value = "/api/v1/users", method = RequestMethod.GET) public ResponseEntity> getAllUsers() { // implementation }
5. Provide Documentation
Good documentation is critical for any API. It should clearly explain how to use the API, including endpoints, request/response models, status codes, and error messages. Interactive documentation using tools like Swagger can make it even easier for developers to understand and test your API.
6. Security
Security should always be a top priority when designing an API. This means implementing proper authentication and authorization mechanisms to protect sensitive data and functionality. It also means validating and sanitizing all input to prevent common attacks such as SQL injection and cross-site scripting (XSS).
7. Think Performance
An API is only as good as its performance. This means optimizing for low latency and fast response times. Caching can be used to reduce load on the server and improve performance for frequently accessed data.
8. Handle Errors Gracefully
When something goes wrong, your API should provide helpful error messages that allow the client to understand what happened and how to fix it. Include error codes that can be used to look up more information and possibly even suggest a course of action to resolve the issue.
9. Be Consistent
Consistency makes your API easier to use and understand. This means being consistent in naming conventions, endpoint paths, request/response formats, status codes, and error handling.
To wrap it up, designing a great API requires careful thought and attention to detail. By following these best practices, you can create an API that is user-friendly, performant, secure, and reliable. With the right approach, your API will be a pleasure for developers to use and will help them build better services for their users.