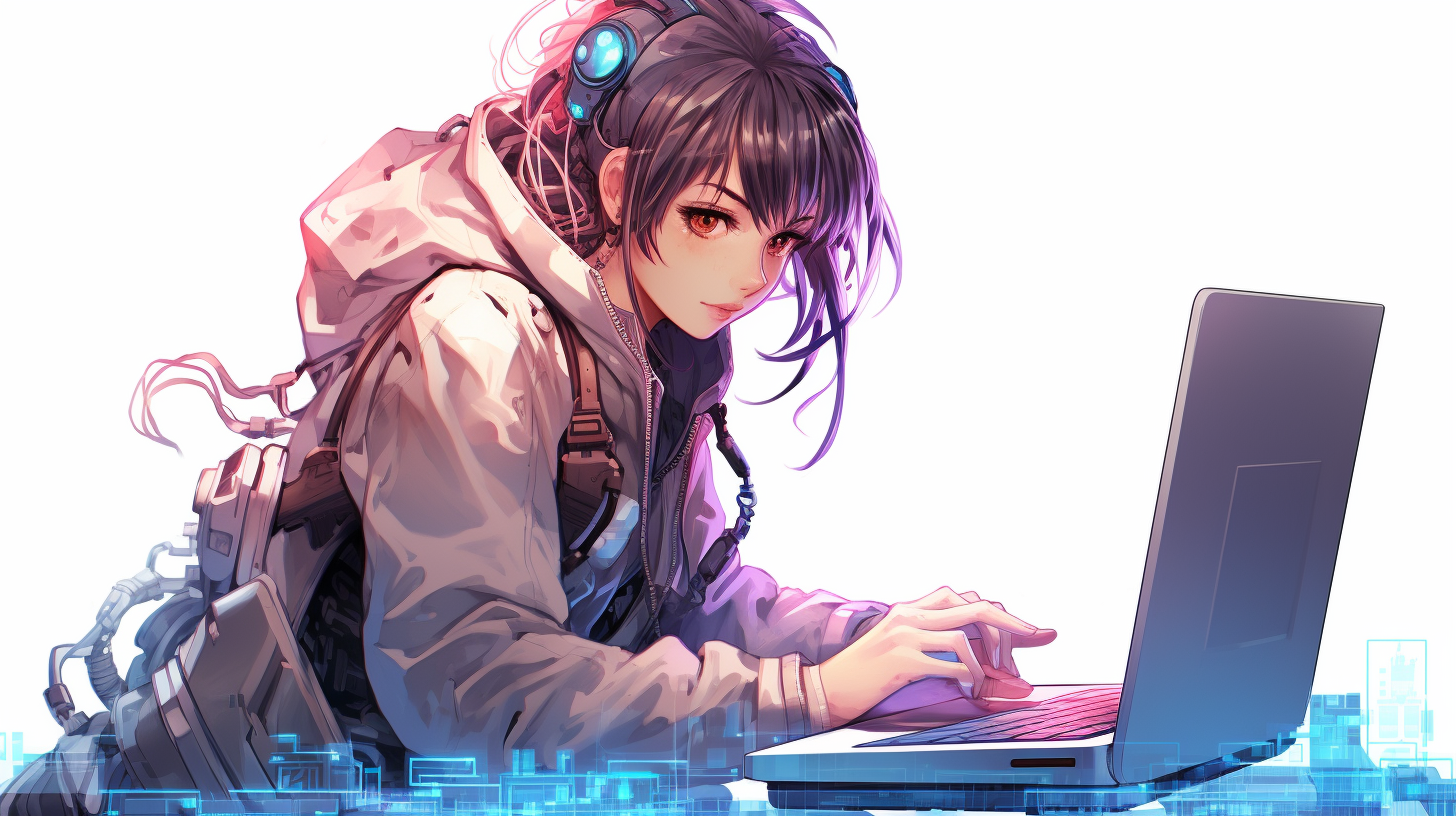
JavaScript Classes and OOP
Introduction to JavaScript Classes
JavaScript classes, introduced in ECMAScript 2015, are a way to create objects and handle inheritance in a more traditional object-oriented programming (OOP) style. Unlike functions, classes offer a more simpler syntax for creating constructor functions and handling prototype-based inheritance.
At its core, a JavaScript class is a type of function. However, classes are declared using the class keyword, followed by the class name and a pair of curly braces that encompass the class body. The class body typically contains a constructor method and any number of other methods that define the behaviors of the class.
class MyClass { constructor(name) { this.name = name; } sayHello() { console.log(`Hello, my name is ${this.name}`); } } const myInstance = new MyClass('Alice'); myInstance.sayHello(); // Output: "Hello, my name is Alice"
The constructor method is a special function that gets called when a new instance of the class is created with the new keyword. It is typically used to initialize the object’s properties.
Classes also support inheritance through the extends keyword, which will allow you to create subclasses that inherit properties and methods from a parent class.
class Animal { constructor(name) { this.name = name; } speak() { console.log(`${this.name} makes a noise.`); } } class Dog extends Animal { constructor(name) { super(name); // Call the parent class constructor with the name parameter } speak() { console.log(`${this.name} barks.`); } } const pet = new Dog('Rex'); pet.speak(); // Output: "Rex barks."
In this example, the Dog class extends the Animal class. The super
call within the Dog
constructor invokes the parent class’s constructor, passing along any arguments. The Dog
class also overrides the speak
method to provide its own implementation.
JavaScript classes provide a clearer and more concise syntax for creating objects and handling inheritance compared to traditional function-based approaches. They are an essential part of modern JavaScript development and are widely used in both front-end and back-end development.
Object-Oriented Programming Concepts
Object-oriented programming (OOP) is a programming paradigm that uses objects and their interactions to design and program applications. OOP is based on several core concepts that make it powerful and flexible. These concepts include encapsulation, abstraction, inheritance, and polymorphism. Understanding these concepts is important for working with JavaScript classes effectively.
Encapsulation refers to the bundling of data and the methods that operate on that data within a single unit, or class. Encapsulation helps to protect the integrity of the data and to manage complexity by restricting access to certain components of an object.
class Person { constructor(name, age) { this.name = name; this.age = age; } getDetails() { console.log(`Name: ${this.name}, Age: ${this.age}`); } } const person1 = new Person('John', 30); person1.getDetails(); // Output: "Name: John, Age: 30"
Abstraction is the concept of hiding the complex reality while exposing only the essentials. It helps in reducing programming complexity and effort. In JavaScript, abstraction can be achieved using classes to hide complex code and provide a simple interface.
class Calculator { constructor() { this.value = 0; } add(num) { this.value += num; } subtract(num) { this.value -= num; } getValue() { return this.value; } } const calc = new Calculator(); calc.add(10); calc.subtract(5); console.log(calc.getValue()); // Output: 5
Inheritance is a mechanism that allows one class to inherit properties and methods from another class. Inheritance promotes code reusability and establishes a relationship between classes.
class Vehicle { constructor(wheels) { this.wheels = wheels; } describe() { console.log(`This vehicle has ${this.wheels} wheels.`); } } class Car extends Vehicle { constructor(wheels, brand) { super(wheels); this.brand = brand; } describe() { super.describe(); console.log(`It is a ${this.brand}.`); } } const car = new Car(4, 'Toyota'); car.describe(); // Output: "This vehicle has 4 wheels." "It is a Toyota."
Polymorphism means “many forms” and it allows objects to be treated as instances of their parent class rather than their actual class. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object.
class Shape { draw() { console.log('Drawing a shape'); } } class Circle extends Shape { draw() { console.log('Drawing a circle'); } } const shapes = [new Shape(), new Circle()]; shapes.forEach(shape => shape.draw()); // Output: "Drawing a shape" // "Drawing a circle"
Understanding OOP concepts such as encapsulation, abstraction, inheritance, and polymorphism is important for working with JavaScript classes. These concepts not only help in creating more organized and manageable code but also enable the development of powerful and scalable applications.
Creating and Using Classes in JavaScript
Now that we’ve covered the basics of JavaScript classes and core OOP concepts, let’s delve into creating and using classes in JavaScript. The process is simpler but powerful, allowing developers to structure their code in a way that’s both readable and reusable.
To define a class in JavaScript, you use the class keyword followed by the class name. Inside the class body, you can define a constructor, which is a special method for creating and initializing objects. Additionally, you can add methods that represent the behavior of the class.
class Person { constructor(firstName, lastName) { this.firstName = firstName; this.lastName = lastName; } getFullName() { return `${this.firstName} ${this.lastName}`; } greet() { console.log(`Hello, my name is ${this.getFullName()}`); } }
To create an instance of a class, you use the new keyword followed by the class name and any necessary arguments for the constructor.
const person = new Person('Jane', 'Doe'); person.greet(); // Output: "Hello, my name is Jane Doe"
It is important to note that methods within a class do not require the function keyword and are separated by commas. Also, all methods are added to the class’s prototype, making them available to all instances of the class.
JavaScript classes also support getter and setter methods which allow for controlled access to the properties of an object. Here’s an example:
class User { constructor(username) { this._username = username; // the underscore is a common convention to indicate a property should not be accessed directly } get username() { return this._username; } set username(newUsername) { // You can add validation or any other logic before setting the property this._username = newUsername; } } const user = new User('jsmith'); console.log(user.username); // Output: "jsmith" user.username = 'jdoe'; console.log(user.username); // Output: "jdoe"
In addition to getters and setters, you can also define static methods that are called on the class itself rather than on instances of the class. These are often utility functions relevant to all instances of a class.
class Calculator { static add(x, y) { return x + y; } static subtract(x, y) { return x - y; } } console.log(Calculator.add(5, 3)); // Output: 8 console.log(Calculator.subtract(5, 3)); // Output: 2
Creating and using classes in JavaScript is a powerful way to organize your code and encapsulate functionality. It allows for easy instantiation of objects while also providing a clear structure for inheritance. As we continue, we’ll explore more advanced features such as inheritance and polymorphism which further enhance the capabilities of JavaScript classes.
Inheritance and Polymorphism in JavaScript Classes
Inheritance and polymorphism are two foundational concepts in object-oriented programming that JavaScript supports through its class syntax. Inheritance allows a class to inherit properties and methods from another class, promoting code reusability and hierarchy. Polymorphism, on the other hand, enables objects to be treated as instances of their parent class rather than their actual class, allowing for flexible and dynamic code.
In JavaScript, inheritance is achieved using the extends keyword. When a class extends another class, it inherits all the properties and methods of the parent class. The child class can also override methods or define new ones. Here’s an example:
class Animal { constructor(name) { this.name = name; } speak() { console.log(`${this.name} makes a noise.`); } } class Dog extends Animal { constructor(name, breed) { super(name); // Call the parent class constructor with the name parameter this.breed = breed; } speak() { // Override the speak method console.log(`${this.name} barks loudly.`); } } const pet = new Dog('Max', 'Labrador'); pet.speak(); // Output: "Max barks loudly."
Notice how the Dog class uses super to call the constructor of the Animal class. That is necessary to ensure that the Animal class is properly initialized before adding or overriding any properties or methods.
Polymorphism in JavaScript allows us to work with objects without needing to know their exact type. That’s particularly useful when dealing with a collection of objects that share a common superclass. For example:
class Cat extends Animal { speak() { console.log(`${this.name} meows.`); } } const animals = [new Animal('Generic Animal'), new Dog('Buddy', 'Golden Retriever'), new Cat('Whiskers')]; animals.forEach(animal => animal.speak()); // Output: "Generic Animal makes a noise." // "Buddy barks loudly." // "Whiskers meows."
In the above example, we created an array of different animal objects, all of which share the Animal superclass. When we call the speak method on each object, JavaScript automatically uses the correct method based on the object’s actual class. This demonstrates polymorphism, where the same operation can behave differently on different classes.
Inheritance and polymorphism are powerful features of JavaScript classes that allow developers to create complex hierarchies and interact with objects in a generic way. Mastering these concepts can lead to more flexible and maintainable code.