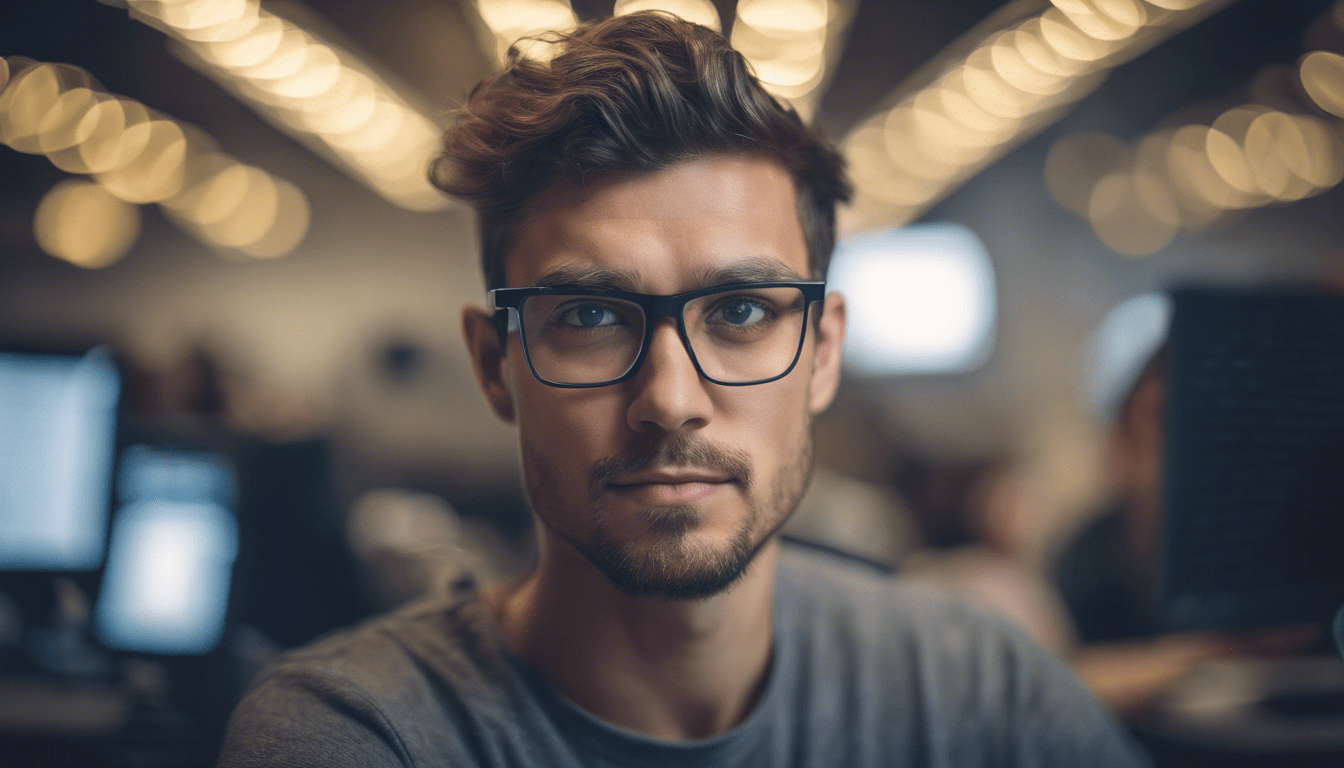
Python and Cryptography: Secure Coding
Python is a versatile and widely-used programming language that is known for its simplicity and ease of use. It isn’t only popular among beginners but also widely used in various domains, including cryptography. Cryptography involves the secure transmission and storage of data, and Python provides powerful tools to implement secure coding practices. In this article, we will dive into the key concepts of Python and Cryptography: Secure Coding and provide a step-by-step guide on how to implement these concepts.
Detailed Explanation of Concepts
Before we begin coding, let’s explore some key concepts related to Python and Cryptography: Secure Coding.
Encryption:
Encryption is the process of converting plain text into cipher text to protect data from unauthorized access. Python provides several libraries, such as cryptography
, that make it easy to implement encryption algorithms.
from cryptography.fernet import Fernet # Generate a secret key key = Fernet.generate_key() # Create a cipher suite with the secret key cipher_suite = Fernet(key) # Encrypt the plain text encrypted_text = cipher_suite.encrypt(b"Hello World") print(encrypted_text)
Decryption:
Decryption is the reverse process of encryption, where cipher text is converted back into plain text. Python libraries like cryptography
provide methods to decrypt encrypted data.
from cryptography.fernet import Fernet # Decrypt the encrypted text decrypted_text = cipher_suite.decrypt(encrypted_text) print(decrypted_text)
Hashing:
Hashing is a one-way function used to convert data into a fixed-size string. It is commonly used to verify the integrity of data by comparing hash values. Python’s hashlib
library provides methods to perform hash functions.
import hashlib # Create a hash object hash_object = hashlib.sha256() # Hash the data hash_object.update(b"Hello World") # Get the hash value hash_value = hash_object.hexdigest() print(hash_value)
Step-by-Step Guide
Now that we understand the key concepts, let’s move on to the step-by-step guide on implementing Python and Cryptography: Secure Coding.
- Install the
cryptography
library by runningpip install cryptography
. This library provides various cryptographic recipes and algorithms. - Create a secret key using the
Fernet.generate_key()
method from thecryptography.fernet
module. - Create a cipher suite with the secret key using
Fernet(key)
. - Encrypt the plain text using the cipher suite’s
encrypt()
method. - Print the encrypted text.
- Decrypt the encrypted text using the cipher suite’s
decrypt()
method. - Print the decrypted text.
Common Pitfalls and Troubleshooting Tips
While working with Python and Cryptography: Secure Coding, beginners might encounter some common issues. Let’s address them with practical advice and troubleshooting tips.
Import Errors:
Make sure you have installed the required libraries, such as cryptography
, using pip install
. Also, double-check the import statements at the beginning of your code.
Encoding Issues:
When working with strings, make sure to encode and decode them correctly. Use your_string.encode()
to convert strings to bytes and your_bytes.decode()
to convert bytes back to strings.
Key Management:
Securely manage your secret keys. Avoid hardcoding them in your code and think storing them in environment variables or separate configuration files.
Further Learning Resources
To further enhance your understanding of Python and Cryptography: Secure Coding, ponder exploring the following resources:
- “Cryptography and Network Security” by William Stallings and “Python Cryptography” by Cryptography.io.
- “Applied Cryptography in Python” on Coursera and “Cryptography I” on Udemy.
- The official documentation for the
cryptography
library: https://cryptography.io/
In today’s digital age, data security is of utmost importance. Understanding the concepts of Python and Cryptography: Secure Coding is important for building secure applications and protecting sensitive information. By implementing encryption, decryption, and hashing techniques in Python, you can ensure the confidentiality, integrity, and authenticity of your data. Remember to continuously learn and practice secure coding practices to stay ahead in the field of cryptography.