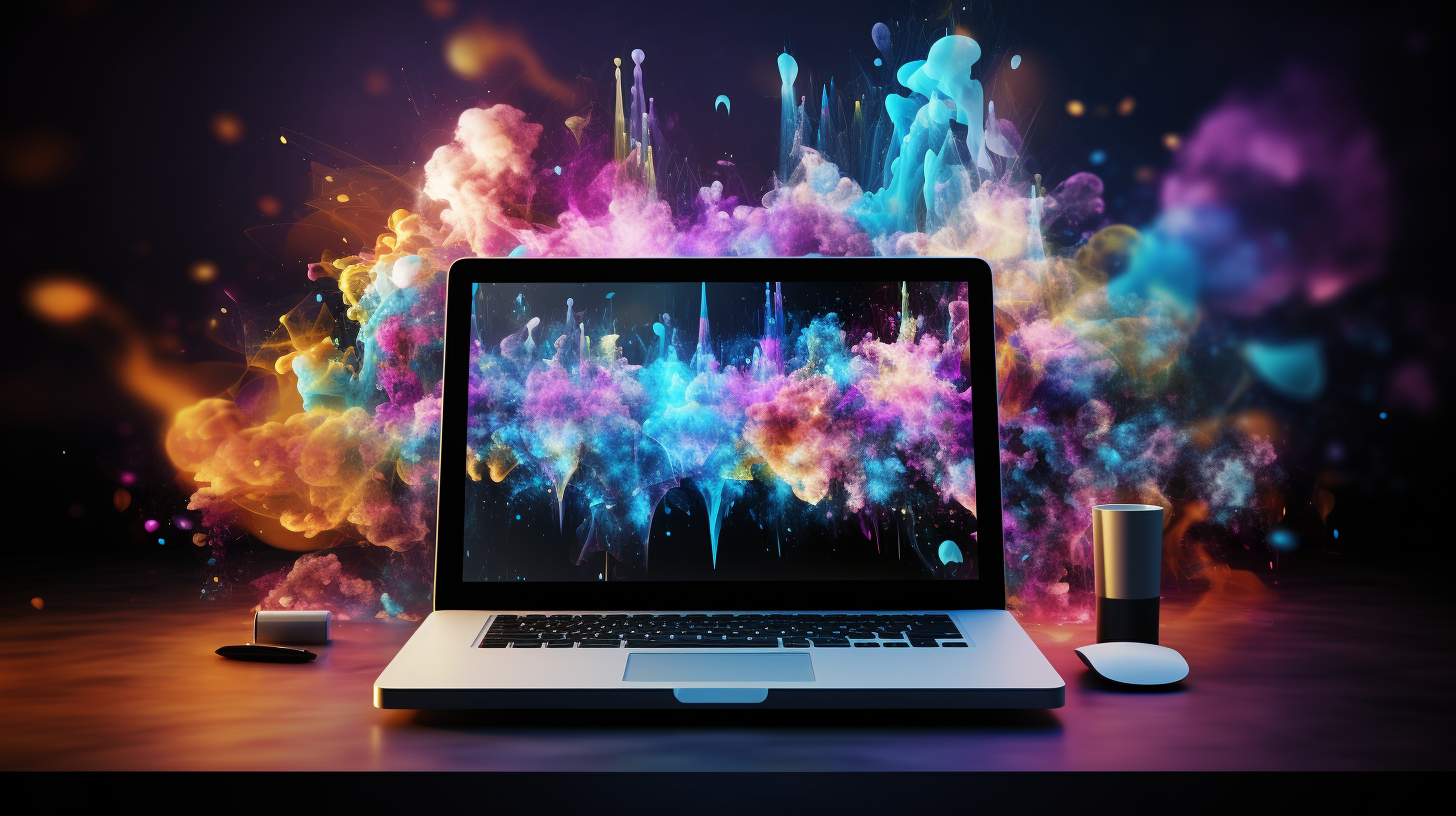
Unit Testing in Swift
Introduction to Unit Testing
Unit testing is a critical aspect of software development, and Swift is no exception. It’s a process where individual units or components of a software are tested to determine if they are fit for use. A unit is the smallest testable part of any software and usually has one or a few inputs and usually a single output. The primary goal of unit testing is to isolate each part of the program and show that the individual parts are correct in terms of requirements and functionality.
Unit tests are typically written and run by software developers to ensure that code meets its design and behaves as intended. In Swift, XCTest framework is commonly used for writing and running unit tests. It provides a set of APIs for creating tests and test cases, managing test execution, and reporting test results.
Unit testing helps in identifying problems early in the development cycle, making it easier to address issues before they become more complex.
Here’s an example of what a simple unit test might look like in Swift:
import XCTest @testable import YourApp class YourAppTests: XCTestCase { func testExample() { let value = true XCTAssertTrue(value, "Value should be true") } }
This example shows a test case YourAppTests
with a single test testExample
that checks if a value is true. If the value isn’t true, the test will fail, showing a message “Value should be true”.
In Swift, unit tests can be run directly from Xcode, which makes it easy to integrate testing into your development workflow. This immediate feedback loop helps developers to write more reliable code and maintain high-quality standards throughout the development process.
- Unit tests should be isolated. They shouldn’t rely on external systems or states.
- Unit tests serve as documentation for your code. They can provide examples of how to use the code being tested.
- Unit tests protect against regression. They help ensure that changes to the code do not break existing functionality.
Understanding the basics of unit testing in Swift sets the foundation for writing effective tests that help build robust applications. As we progress through this article, we’ll dive deeper into writing unit tests in Swift and explore best practices to maximize their effectiveness.
Writing Unit Tests in Swift
Writing unit tests in Swift is a straightforward process, thanks to the XCTest framework. To get started, you first need to create a test case class. This class will inherit from XCTestCase
and contain all your test methods. A test method is a function that starts with the word “test” and contains the logic to perform a specific test.
Here’s a step-by-step guide to writing a unit test in Swift:
- That is necessary to access all the functionalities provided by the testing framework.
- Use this attribute before importing your app module to allow the test cases access to your app’s internal entities.
- This class should inherit from
XCTestCase
. - Each method should start with the word “test” and contain assertions to validate the code being tested.
Here is an example of a test case that checks for the successful initialization of a custom Person
struct:
import XCTest @testable import YourApp class PersonTests: XCTestCase { func testPersonInitialization() { let person = Person(name: "Mitch Carter", age: 30) XCTAssertNotNil(person, "Person should not be nil") XCTAssertEqual(person.name, "Mitch Carter", "Name should be 'Mitch Carter'") XCTAssertEqual(person.age, 30, "Age should be 30") } }
In this example, we have three assertions:
- Ensures that the
person
object is not nil after initialization. - Checks that the
name
andage
properties are set correctly.
Assertions are the core of unit testing in Swift. They validate that certain conditions are met during test execution. If an assertion fails, the test method will stop executing, and the failure will be reported. This helps in quickly identifying issues with the code.
You can run your tests directly in Xcode by using the product > Test menu option or by pressing Command + U. Xcode provides a test navigator where you can see all your tests and their status, whether they passed or failed.
Writing unit tests might seem like extra work, but it’s an investment that pays off in the long run. It ensures that your codebase remains healthy and easy to maintain. In the next section, we’ll look at some best practices for unit testing in Swift to help you make the most out of your tests.
Best Practices for Unit Testing in Swift
When it comes to unit testing in Swift, following best practices can significantly improve the quality and reliability of your tests. Here are some key practices to keep in mind:
- Test One Thing at a Time: Each test case should focus on a single aspect of your code. This practice makes it easier to identify the source of any failures and ensures that tests are not dependent on each other.
- Keep Tests Short and Fast: Unit tests should be quick to run. Swift developers often run tests frequently, so keeping them short helps maintain a rapid development cycle.
- Use Descriptive Test Method Names: The name of your test method should clearly describe what the test is checking. This can act as documentation and make it easier for others (or yourself in the future) to understand the purpose of the test.
- Arrange-Act-Assert Pattern: Structure your tests with setup (Arrange), execution (Act), and verification (Assert) phases. This pattern helps keep your tests organized and clear.
Here’s an example that demonstrates these best practices:
import XCTest @testable import YourApp class CalculatorTests: XCTestCase { func testAddition_TwoPlusTwo_EqualsFour() { // Arrange let calculator = Calculator() // Act let result = calculator.add(a: 2, b: 2) // Assert XCTAssertEqual(result, 4, "Addition result should be 4") } }
In this example, the test method name testAddition_TwoPlusTwo_EqualsFour
clearly states what the test is supposed to verify. The test itself is short, and the Arrange-Act-Assert pattern is followed.
- Avoid Testing Implementation Details: Focus on testing the behavior and output of your code, not the specific implementation. This allows you to refactor the code later without needing to change your tests.
- Mock Dependencies: When testing a unit that depends on other components, use mocks or stubs for those dependencies. This ensures that your tests are truly isolated and not affected by external factors.
Let’s look at an example of mocking a dependency:
import XCTest @testable import YourApp class UserServiceTests: XCTestCase { var userService: UserService! var mockNetworkManager: MockNetworkManager! override func setUp() { super.setUp() mockNetworkManager = MockNetworkManager() userService = UserService(networkManager: mockNetworkManager) } func testFetchUser_Success_ReturnsUser() { // Arrange mockNetworkManager.mockResponse = User(name: "Alice", age: 28) // Act userService.fetchUser { user, error in // Assert XCTAssertNotNil(user) XCTAssertEqual(user?.name, "Alice") XCTAssertNil(error) } } } class MockNetworkManager: NetworkManaging { var mockResponse: User? func fetch(completion: @escaping (User?, Error?) -> Void) { completion(mockResponse, nil) } }
In this example, MockNetworkManager
is used to simulate network behavior without making actual network calls. This isolation helps in testing only the UserService
logic.
By adhering to these best practices, you can ensure that your unit tests in Swift are effective and maintainable. Remember, well-written tests can save time in the long run by catching issues early and simplifying the debugging process.