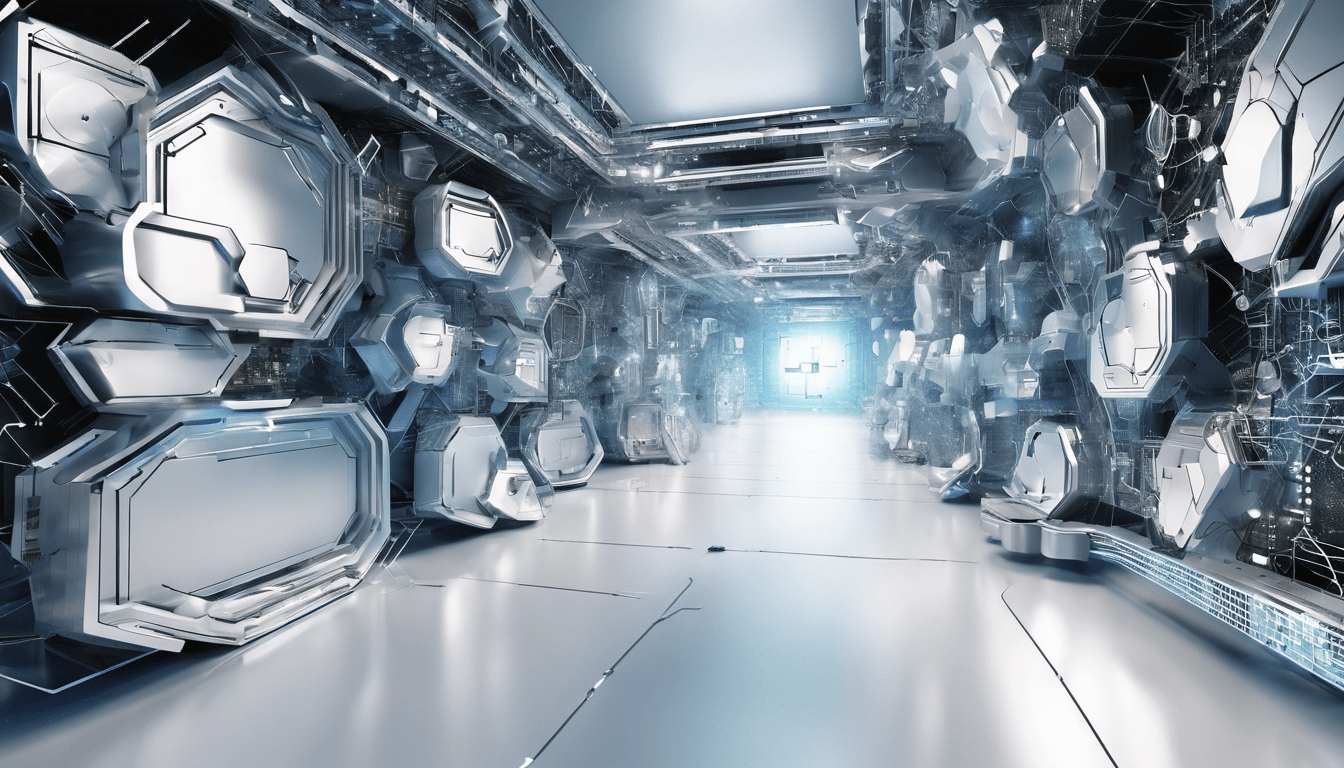
Using JavaScript with HTML5 Canvas
HTML5 Canvas is a powerful element that allows developers to draw graphics on a web page. With the help of JavaScript, you can create interactive and dynamic visuals that can enhance the user experience of your website. In this article, we’ll explore how to use JavaScript with HTML5 Canvas to create simple shapes and animations.
First, let’s start with the basics. To use canvas, you need to include it in your HTML markup:
This creates a canvas element with an ID of “myCanvas”, which we can then target with JavaScript. The width and height attributes define the size of the canvas.
Next, we need to access the canvas element with JavaScript and create a drawing context. The drawing context is what allows us to draw on the canvas:
var canvas = document.getElementById("myCanvas"); var ctx = canvas.getContext("2d");
Now that we have our drawing context, we can start creating graphics. Let’s start by drawing a simple rectangle:
ctx.fillStyle = "blue"; ctx.fillRect(10, 10, 150, 75);
The fillStyle
property sets the color of the shape, and the fillRect
method draws the rectangle. The parameters for fillRect
are the x and y coordinates of the top left corner of the rectangle, followed by its width and height.
In addition to rectangles, we can draw other shapes like circles:
ctx.beginPath(); ctx.arc(50, 50, 25, 0, Math.PI * 2); ctx.fillStyle = "red"; ctx.fill();
The beginPath
method starts a new path for drawing, and the arc
method creates a circle with the specified x and y coordinates, radius, start angle, and end angle (in radians). We then set the fill color to red and call the fill
method to draw the circle.
We can also create animations by continuously updating the canvas using the requestAnimationFrame
method:
var x = 0; function animate() { ctx.clearRect(0, 0, canvas.width, canvas.height); ctx.fillRect(x, 10, 50, 50); x += 1; requestAnimationFrame(animate); } animate();
In this example, we create a function called animate
that clears the canvas with clearRect
, draws a rectangle at an increasing x coordinate using fillRect
, increments the x coordinate by 1, and then calls itself recursively with requestAnimationFrame
to create a smooth animation.
The HTML5 Canvas and JavaScript open up endless possibilities for creating interactive and dynamic graphics on your web pages. With a little practice and creativity, you can use these tools to imropve your web projects and engage your users.
Remember to experiment with different shapes and colors, and don’t be afraid to try out new techniques. Happy coding!