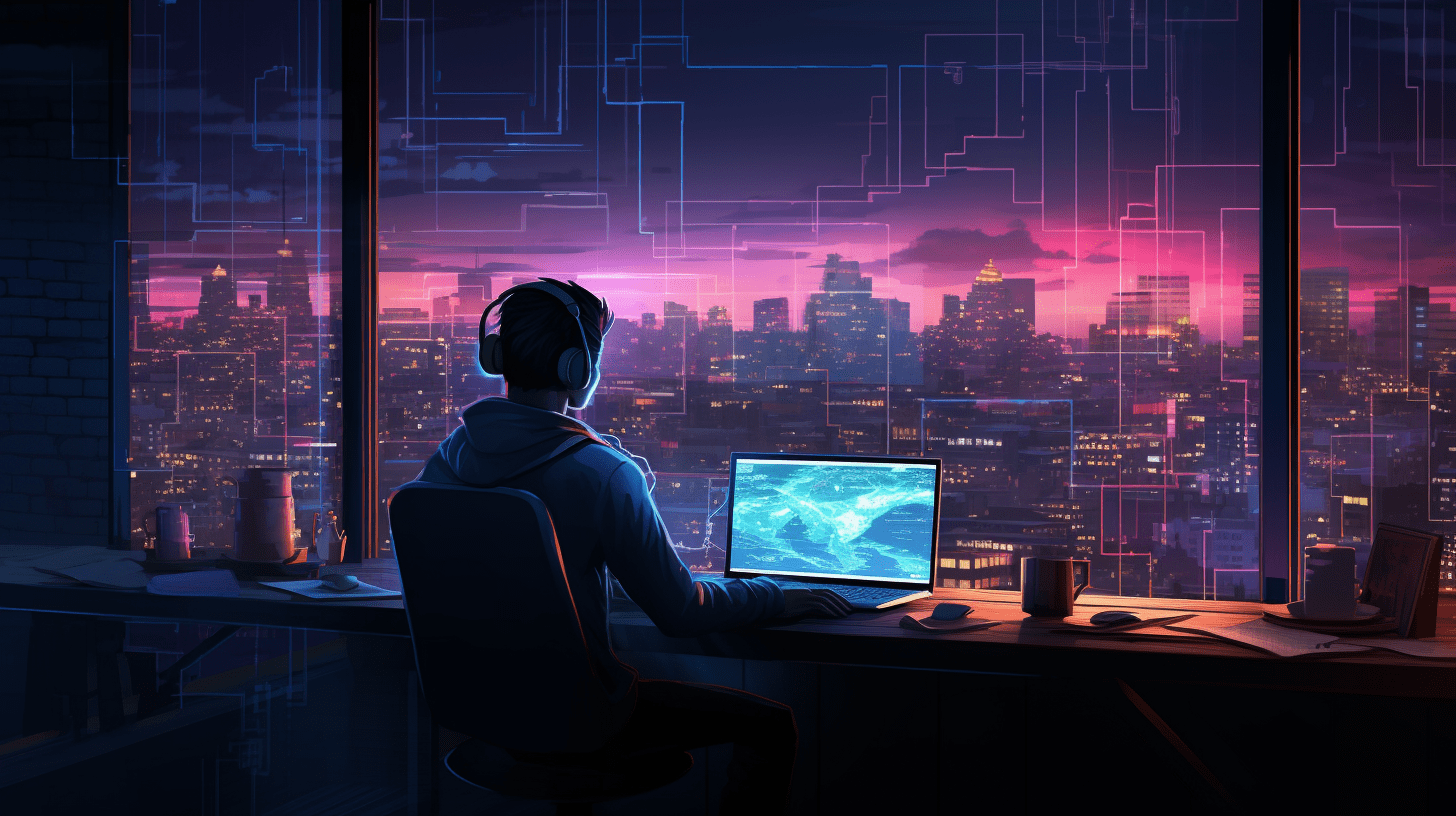
Building Your First JavaScript Game
Setting up the Development Environment
Before diving into building your first JavaScript game, it is essential to set up a proper development environment. This will ensure that you have all the tools needed to code effectively and efficiently. Here’s how to get started:
Choose a code editor: There are many code editors out there, but some popular ones for JavaScript development include Visual Studio Code, Sublime Text, and Atom. Choose one that you’re comfortable with and that offers features like syntax highlighting, code completion, and debugging tools.
// Example of a simple JavaScript code snippet you can write in a code editor console.log("Hello, world!");
Install Node.js: Node.js is a runtime environment that allows you to run JavaScript on the server. It is useful for building server-side components of your game, like handling multiplayer capabilities or storing high scores. Download it from the official Node.js website.
Note: After installation, you can check if Node.js is installed correctly by opening your command line and typing node -v
. You should see the version number displayed.
Set up a local server: To test your game locally, you’ll need a local server. An easy way to do that is by using the http-server
package from npm (Node Package Manager). First, install npm by typing the following in your command line:
npm install -g npm
After installing npm, install http-server
:
npm install -g http-server
Once installed, navigate to your project directory in the command line and start the server with:
http-server
Your game will now be accessible at http://localhost:8080 in your web browser.
Get familiar with browser developer tools: Contemporary web browsers come with developer tools that are invaluable for debugging your game. Learn how to use the JavaScript console, debugger, and network inspector. With these tools, you can step through your code, inspect variables, and view any network requests made by your game.
With these initial steps taken care of, you’ll have a solid foundation for starting to build your game. As you progress, you may need additional tools and libraries, but these basics will take you a long way.
Creating the Game Logic
Creating the Game Logic
The heart of any game is its logic. It’s what defines the rules, tracks the score, and determines the win or lose conditions. In JavaScript games, this logic is implemented through functions and event listeners that respond to player actions. Let’s start by defining the basic structure of our game logic.
Firstly, we need to initialize our game variables. These would typically include the player’s score, the game state, and any other necessary settings. For example:
let score = 0; let gameState = "start";
Next, we have to create functions that run the game. One such function could be startGame, which sets up the game and prepares it for play. It might reset the game variables and display the initial game screen:
function startGame() { score = 0; gameState = "playing"; // Additional setup code here }
Another important function is endGame, which is called when the game is over. This function would handle things like stopping the game loop, displaying the final score, and offering the player a chance to restart:
function endGame() { gameState = "ended"; // Code to handle end of game, such as displaying score }
In addition to these, we need functions that respond to player interactions. These are often set up as event listeners. For example, in a simple clicking game where the objective is to click on objects as they appear on screen, we might have:
document.addEventListener("click", function(event) { if (gameState === "playing") { score++; // Code to handle object being clicked } });
Apart from handling clicks, you will also need to define functions for different types of input like key presses or mouse movements depending on your game’s mechanics.
The final component of your game’s logic is the game loop. That’s what keeps the game running, frequently checking for changes and updating the screen accordingly. A very simple game loop could look something like this:
function gameLoop() { if (gameState === "playing") { // Code to update game objects for each frame // Check for win/lose conditions } requestAnimationFrame(gameLoop); } // Start the game loop requestAnimationFrame(gameLoop);
With these main components in place – initialization, start/end functions, input handling, and a game loop – you have a framework for your game logic. It’s important to test each part thoroughly and make sure they work together seamlessly.
Remember that good game logic not only makes the game functional but also enjoyable to play. Keep refining it until you’re satisfied with how the game feels. Once the logic is in place, you can move on to designing a great user interface for players to interact with.
Designing the User Interface
The user interface (UI) is what players will interact with while playing your game. It is critical that the UI is intuitive, responsive, and visually appealing. This section will guide you through designing a UI that enhances your game’s playability and user experience.
Layout and Visual Elements
Begin by sketching out a layout for your game’s UI. Identify where on the screen the game’s various elements will go, such as the scoreboard, player controls, and game area. Keep in mind the following when designing your layout:
- Ensure that important information is easily visible.
- Avoid clutter by spacing out elements appropriately.
- Use contrasting colors to differentiate interactive elements from non-interactive ones.
After you have a layout, create the visual assets for your UI. This might include buttons, icons, and backgrounds. For our game, we could have something as simple as this:
Interactivity
Interactive elements need to be clearly marked and responsive to player input. Think adding hover and active states to buttons using CSS so players know when they’re clickable.
#startButton:hover { background-color: #ccc; } #startButton:active { background-color: #aaa; }
Feedback
Players should receive immediate feedback for their actions. This could be through sound effects, animations, or updating score displays. Here’s how you might increase the score and give visual feedback when a player clicks an object in the game area:
document.querySelector('#gameArea').addEventListener('click', function() { if (gameState === "playing") { score++; document.querySelector('#score').textContent = score; // Visual feedback this.classList.add('clicked'); setTimeout(() => this.classList.remove('clicked'), 100); } });
Note: The ‘clicked’ class should define a noticeable visual change in your CSS.
Accessibility
Accessibility is often overlooked in game development, which can exclude players with disabilities from enjoying your game. Ensure that all players can access your game by:
- Providing alternate control schemes for those who cannot use a mouse or keyboard.
- Using high-contrast colors for those with visual impairments.
- Offering captions or visual indicators for any sound-based gameplay elements.
Testing
Once you have designed your UI, test it thoroughly. Observe how players interact with each element and make adjustments as necessary. The success of your game heavily relies on how well players can understand and interact with your UI.
You might not get the UI right on the first try, but iterative design and testing will go a long way in improving user experience.
With your UI designed, you’re one step closer to having a complete game. Next, you’ll need to focus on testing and deploying your game so others can enjoy it.
Testing and Deploying the Game
Testing your JavaScript game is a important step before deployment. It’s where you’ll ensure there are no bugs, the game logic works as intended, and it is generally enjoyable to play. Here are some things to ponder when testing your game:
- Play through your game multiple times, testing all possible actions a player can take. Make sure the game progresses and ends as expected.
- Test your game on different devices and browsers to check for any lag or performance issues. That is important to provide a smooth gaming experience for all players.
- Ensure that all UI elements are intuitive and function correctly. This includes buttons, controls, and any interactive components.
- Look for and fix any glitches or bugs in the game. Use browser developer tools like the console and debugger to pinpoint issues.
It’s also a good idea to have others test your game. They can provide invaluable feedback and spot issues you may have missed.
Once testing is complete, it is time to deploy your game. There are several options for deployment, which vary based on your specific needs and goals. You might deploy to a personal website, use a cloud service like Amazon Web Services or Microsoft Azure, or submit it to online game portals.
To prepare for deployment, first, you might want to optimize your code by:
- Minifying your JavaScript files
- Optimizing images and assets
- Ensuring your code is error-free and well-documented
The deployment process will typically include:
- Uploading your game files to a web server
- Setting up any necessary configurations, such as security settings or database connections
- Testing the live version intensively to ensure everything runs as smoothly as it did during development
Don’t forget to think the legal aspects such as copyright, licensing of assets, and terms of service for your game.
In conclusion, testing and deploying your JavaScript game might seem daunting at first, but with careful planning and thorough testing, you can ensure a successful launch.
Remember that deployment isn’t the end of development; you will likely need to provide updates, fix bugs, and maybe even add new features based on player feedback.
Here’s an example of a simple deployment script that you could execute from the command line:
// Assuming you have an FTP client installed // Replace the placeholders with your actual server information ftp -u ftp://:@ ./public_html// *
A Simple JavaScript Game
In this final chapter, we’ll create a simple yet engaging game to demonstrate basic game development principles. Our game will be a classic “Catch the Falling Objects” game where the player moves a basket to catch objects falling from the top of the screen.
Game Concept
The game’s objective is straightforward: catch as many falling objects as possible within a set time limit. Missing an object results in a loss of points. The game ends when the timer runs out.
Implementation
We’ll use basic HTML for the game’s structure and JavaScript for its functionality. No advanced libraries are required, making this a great starting point for beginners.
<!DOCTYPE html> <html> <head> <title>Simple JavaScript Game</title> <style> #gameCanvas { border: 1px solid black; background-color: #f0f0f0; } </style> </head> <body> <canvas id="gameCanvas" width="800" height="600"></canvas> <script> const canvas = document.getElementById('gameCanvas'); const ctx = canvas.getContext('2d'); let basket = { x: canvas.width / 2, y: canvas.height - 50, width: 100, height: 20 }; let fallingObjects = []; let score = 0; const gravity = 2; let objectCreationInterval = 1000; let lastCreationTime = Date.now(); function drawBasket() { ctx.fillStyle = '#654321'; ctx.fillRect(basket.x, basket.y, basket.width, basket.height); } function addFallingObject() { fallingObjects.push({ x: Math.random() * canvas.width, y: 0, size: 20 }); } function checkCollision(obj) { if (obj.x > basket.x && obj.x < basket.x + basket.width && obj.y + obj.size > basket.y && obj.y < basket.y + basket.height) { return true; } return false; } function drawFallingObjects() { fallingObjects = fallingObjects.filter(obj => { let collision = checkCollision(obj); if (!collision) { ctx.fillStyle = 'red'; ctx.beginPath(); ctx.arc(obj.x, obj.y, obj.size, 0, Math.PI * 2); ctx.fill(); obj.y += gravity; return obj.y <= canvas.height; } else { score++; return false; } }); } function updateGame() { let currentTime = Date.now(); if (currentTime - lastCreationTime > objectCreationInterval) { addFallingObject(); lastCreationTime = currentTime; } ctx.clearRect(0, 0, canvas.width, canvas.height); drawBasket(); drawFallingObjects(); ctx.font = '20px Arial'; ctx.fillStyle = 'black'; ctx.fillText('Score: ' + score, 10, 30); requestAnimationFrame(updateGame); } updateGame(); document.addEventListener('mousemove', (event) => { basket.x = event.clientX - canvas.getBoundingClientRect().left - basket.width / 2; }); </script> </body> </html>
In this code:
- We define a simple HTML canvas to act as our game area.
- The JavaScript part handles the game logic.
drawBasket()
function renders the player’s basket.addFallingObject()
creates new objects at random positions.drawFallingObjects()
renders these objects and applies gravity.updateGame()
is the main game loop, redrawing the canvas for each frame.- Mouse movement is tracked to move the basket.
This code provides a fundamental structure for a simple JavaScript game. You can expand upon it by adding scores, levels, or additional gameplay features. This simple game demonstrates how JavaScript can be used to create interactive and fun web games. With these basics, you can experiment further and develop more complex games, applying your creativity and programming skills.