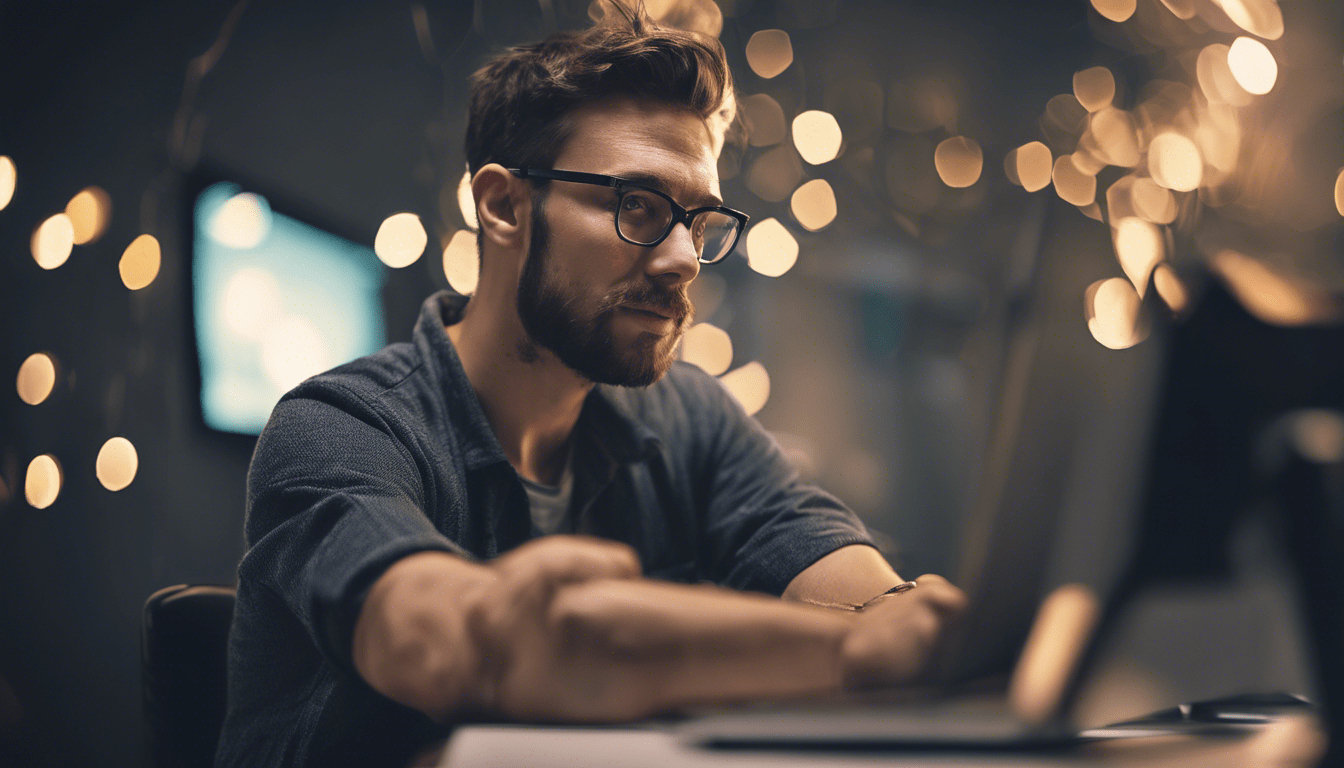
JavaScript and Blockchain Basics
JavaScript and blockchain are two technologies that are quickly becoming essential for contemporary web developers to understand. JavaScript is a powerful programming language used to create interactive websites and web applications, while blockchain is a distributed ledger technology that allows for secure and transparent transactions. Together, they can be used to create decentralized applications (DApps) on the Ethereum network.
Blockchain technology is most commonly associated with cryptocurrencies like Bitcoin and Ethereum, but it has many other potential uses as well. At its core, a blockchain is simply a chain of blocks, each containing a list of transactions. These blocks are linked together using cryptography, making the blockchain incredibly secure and difficult to tamper with.
One of the key features of blockchain technology is the use of smart contracts. Smart contracts are self-executing contracts with the terms of the agreement written directly into code. They are stored on the blockchain and can be automatically executed when certain conditions are met. This makes them perfect for automating complex processes and reducing the need for intermediaries.
To get started with JavaScript and blockchain development, you’ll first need to install some tools. The most important of these is Node.js, which is a JavaScript runtime that enables you to run JavaScript code outside of a web browser. You’ll also need a text editor like Visual Studio Code or Sublime Text, and a blockchain development environment like Truffle or Hardhat.
Once you have these tools installed, you can start writing your first smart contract. Here’s a simple example of a smart contract written in Solidity, the programming language used for Ethereum smart contracts:
pragma solidity ^0.5.0; contract HelloWorld { string public message; constructor() public { message = "Hello, world!"; } function setMessage(string memory _message) public { message = _message; } }
This contract simply stores a message and allows anyone to change it by calling the setMessage
function. To interact with this contract from a JavaScript application, you’ll need to use a library like web3.js or ethers.js. Here’s an example of how you might do that:
const Web3 = require('web3'); const web3 = new Web3('http://localhost:8545'); const contractAddress = '0x...'; // replace with your contract address const abi = [ ... ]; // replace with your contract ABI const helloWorldContract = new web3.eth.Contract(abi, contractAddress); helloWorldContract.methods.message().call() .then((message) => { console.log(message); }); helloWorldContract.methods.setMessage('Hello, Ethereum!').send({ from: '0x...' }) // replace with your account address .then((receipt) => { console.log(receipt); });
Of course, this is just a very basic example of what you can do with JavaScript and blockchain technology. As you learn more, you’ll discover how to create more complex DApps that can interact with multiple smart contracts, handle user authentication, and integrate with other blockchain networks.
Blockchain development is still a relatively new field, so there’s plenty of room for innovation and creativity. If you’re a JavaScript developer looking to expand your skills, there’s never been a better time to get involved in blockchain development.