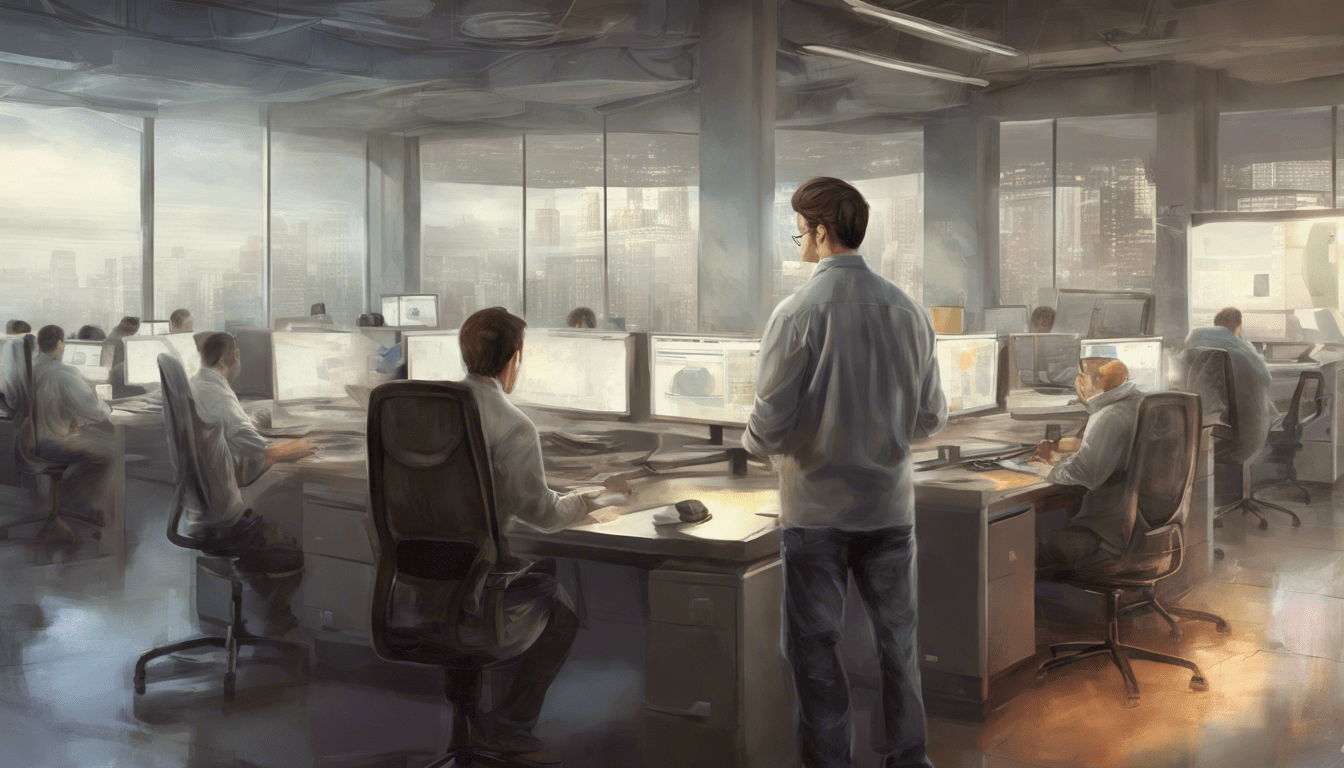
Using Python Modules and Libraries
Python is a versatile and powerful programming language this is widely used in various fields such as web development, data analysis, machine learning, and more. One of the reasons for its popularity is the vast collection of modules and libraries available that can be easily integrated into your projects. In this article, we will discuss what modules and libraries are, and how to use them in Python.
What are Modules and Libraries?
Modules in Python are simply files containing Python code that define functions, classes, and variables. These modules can be imported into other Python files to use the functionality they provide. A library, on the other hand, is a collection of modules that are related and can be used together to perform specific tasks.
Importing Modules
To use a module in your Python code, you need to import it using the import
statement. The basic syntax for importing a module is:
import module_name
For example, if you want to use the math
module which provides mathematical functions, you would write:
import math
Once you have imported the module, you can use its functions by using the following syntax:
module_name.function_name()
For example, to use the sqrt
function from the math
module to calculate the square root of a number, you would write:
import math result = math.sqrt(16) print(result) # This will print 4.0
Importing Specific Functions
If you only need to use a specific function from a module, you can import just that function using the from
keyword:
from module_name import function_name
For example, to import only the sqrt
function from the math
module:
from math import sqrt result = sqrt(16) print(result) # This will print 4.0
Importing Multiple Functions
You can also import multiple functions from a module in a single line by separating them with a comma:
from module_name import function1, function2
Alias for Modules
If you find that the name of a module is too long or conflicts with another name in your code, you can create an alias for it using the as
keyword:
import module_name as alias_name
For example, the pandas
library is commonly imported with an alias:
import pandas as pd
Now you can use the alias pd
instead of pandas
in your code:
import pandas as pd df = pd.DataFrame(data)
Finding More Modules and Libraries
The Python standard library comes with a wide range of modules that provide functionality for common tasks. However, there are many more third-party libraries available that can be installed using package managers like pip
. To find new libraries, you can search the Python Package Index (PyPI) which is a repository of software for the Python programming language.
In conclusion, using modules and libraries in Python is an essential skill for any Python developer. They allow you to leverage existing code to perform common tasks without having to reinvent the wheel. With a bit of practice, you’ll be able to easily integrate these powerful tools into your projects.