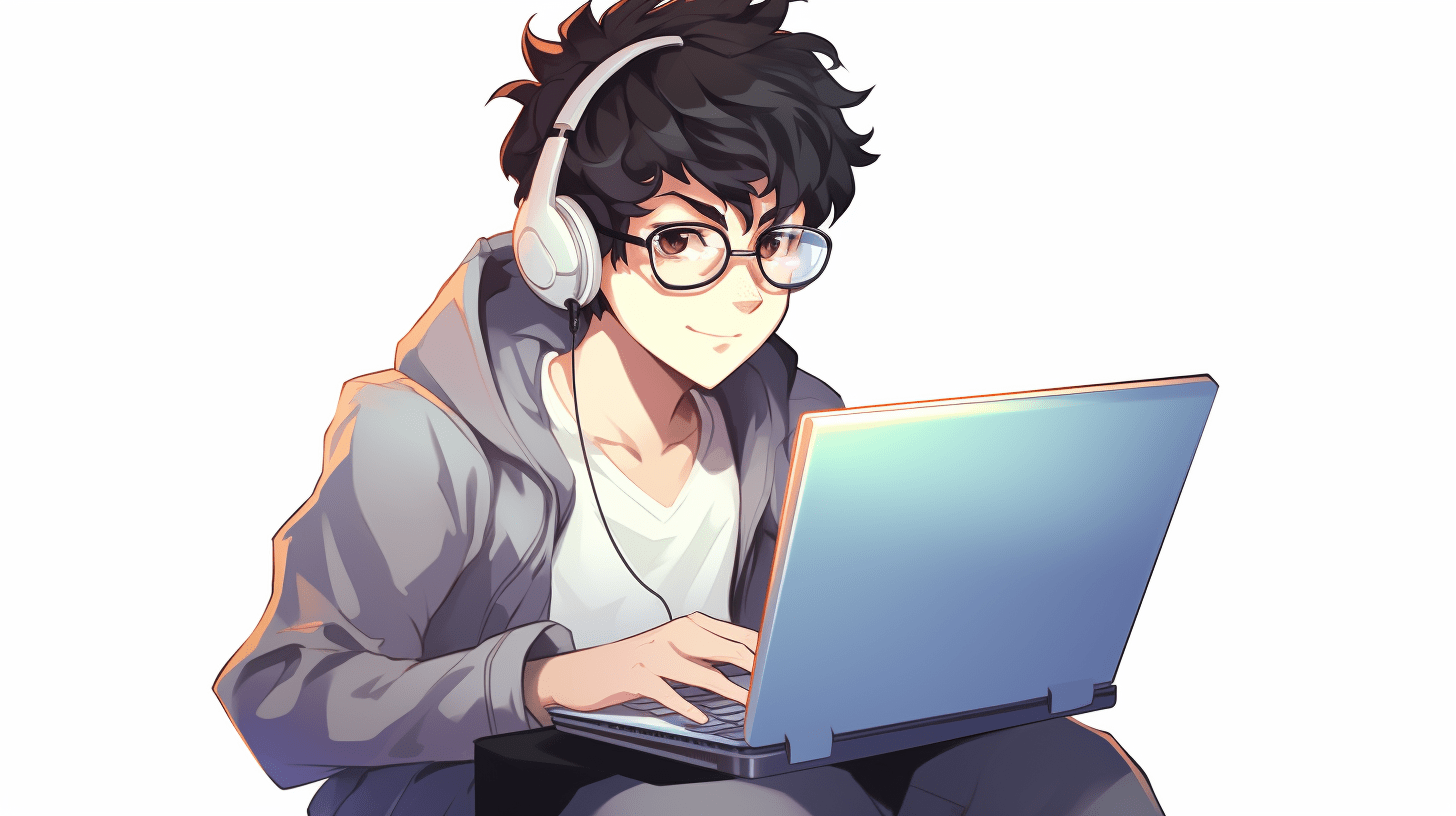
JDBC: Connecting Java with Databases
Introduction to JDBC
JDBC, short for Java Database Connectivity, is an API (Application Programming Interface) that enables Java programs to interact with various databases. It’s a part of the Java Standard Edition platform and provides methods to query and update data in a database. JDBC also allows for the seamless integration of various database operations into Java applications.
JDBC acts as a bridge between Java applications and databases by allowing developers to send SQL statements to the database and process the results. That’s achieved through the use of JDBC drivers, which are specific to each database vendor and must be installed and configured properly to establish a connection.
The core components of JDBC include:
- This class manages a list of database drivers and establishes a connection between the database and the appropriate driver.
- Represents a connection with a database and provides methods to manage transactions and create statements.
- Used for executing SQL queries and updating records in the database.
- Represents the result set of a query, providing access to the data retrieved from the database.
- An exception that provides information on database access errors or other errors related to JDBC.
To use JDBC, you need to follow these basic steps:
- Load the JDBC driver.
- Establish a connection to the database.
- Create a statement object to send SQL commands.
- Execute SQL queries or updates.
- Process the results obtained from the database.
- Close the connections and clean up resources.
A simple example of how to load a JDBC driver and establish a connection is shown below:
try { // Load the JDBC driver Class.forName("com.mysql.cj.jdbc.Driver"); // Establish a connection Connection conn = DriverManager.getConnection( "jdbc:mysql://localhost:3306/mydatabase", "username", "password" ); // Do something with the connection here // Close the connection conn.close(); } catch (ClassNotFoundException e) { System.out.println("JDBC Driver not found"); } catch (SQLException e) { System.out.println("Error connecting to the database: " + e.getMessage()); }
It’s important to note that different databases require different JDBC drivers. For example, to connect to an Oracle database, you would need to use the Oracle JDBC driver. The URL format used in DriverManager.getConnection
also varies based on the database vendor.
JDBC provides a flexible and standardized way for Java applications to interact with databases, making it an essential tool for any Java developer working with data persistence.
Establishing Database Connections in Java
Establishing a database connection in Java using JDBC involves several key steps. First, you need to ensure that the JDBC driver for your chosen database is available on the classpath. Next, you will use the DriverManager class to obtain a Connection object, which represents a physical connection to the database.
To establish a connection, you will typically need the following information:
- The JDBC driver class name
- The database URL
- A username and password
Here is a step-by-step guide to establishing a database connection:
-
Loading the JDBC Driver: You must first load the JDBC driver by calling the
Class.forName()
method. This step is necessary to register the driver with the DriverManager. Make sure that the JDBC driver JAR file is included in your project’s build path.Class.forName("com.mysql.cj.jdbc.Driver");
-
Establishing a Connection: Use the
DriverManager.getConnection()
method to establish a connection to the database. You’ll need to pass the database URL, user credentials, and any other necessary connection properties.Connection conn = DriverManager.getConnection( "jdbc:mysql://localhost:3306/mydatabase", "username", "password" );
-
Handling Exceptions: Both loading the driver and establishing a connection can throw exceptions, so it is important to handle these properly. The most common exceptions are
ClassNotFoundException
, if the JDBC driver is not found, andSQLException
, if there are issues connecting to the database.try { // Load the driver and establish a connection } catch (ClassNotFoundException e) { System.out.println("JDBC Driver not found: " + e.getMessage()); } catch (SQLException e) { System.out.println("Error connecting to the database: " + e.getMessage()); }
-
Closing the Connection: It is important to close the database connection once your operations are complete to free up resources. This should be done in a finally block or using try-with-resources to ensure that it executes regardless of whether an exception occurs.
finally { if (conn != null) { try { conn.close(); } catch (SQLException e) { // Handle potential exception on close } } }
By following these steps, you can establish a reliable connection to your database and begin executing SQL queries and updates through your Java application.
Note: The database URL format can vary based on the database vendor. For instance, connecting to PostgreSQL would require a different URL format and possibly different credentials.
Always ensure that your database credentials are kept secure and are not hard-coded into your application’s source code. Think using environment variables or configuration files to manage sensitive information.
Executing SQL Queries and Managing Results
Once a database connection is established, the next step is to execute SQL queries and manage the results. The Statement
, PreparedStatement
, and CallableStatement
interfaces in JDBC provide mechanisms to execute SQL commands and retrieve data from a database.
To execute a SQL query, you need to create a Statement
object using the Connection
object’s createStatement()
method. Then, you can use the executeQuery()
method to execute SELECT statements or executeUpdate()
for INSERT, UPDATE, DELETE, or DDL statements. The results of a SELECT query are returned in a ResultSet
object.
Here’s an example of executing a SELECT query:
try (Statement stmt = conn.createStatement(); ResultSet rs = stmt.executeQuery("SELECT * FROM employees")) { while (rs.next()) { int id = rs.getInt("id"); String name = rs.getString("name"); // Process each row of the result set } } catch (SQLException e) { System.out.println("Error executing query: " + e.getMessage()); }
For INSERT, UPDATE, and DELETE operations, you can use the executeUpdate()
method which returns an integer representing the number of rows affected by the SQL statement:
try (Statement stmt = conn.createStatement()) { int rowsAffected = stmt.executeUpdate("UPDATE employees SET salary = 50000 WHERE id = 1"); System.out.println("Rows affected: " + rowsAffected); } catch (SQLException e) { System.out.println("Error executing update: " + e.getMessage()); }
If you are executing a query with parameters, it’s safer and more efficient to use a PreparedStatement
. It helps prevent SQL injection attacks and can improve performance by reusing the same SQL statement with different parameters:
String sql = "SELECT * FROM employees WHERE department = ?"; try (PreparedStatement pstmt = conn.prepareStatement(sql)) { pstmt.setString(1, "Sales"); try (ResultSet rs = pstmt.executeQuery()) { while (rs.next()) { // Process result set } } } catch (SQLException e) { System.out.println("Error executing prepared statement: " + e.getMessage()); }
Finally, it is important to always close the ResultSet
, Statement
, and Connection
objects to free up database resources. This can be done in a finally
block or, as shown above, using try-with-resources which ensures these resources are closed automatically.
In summary, managing SQL queries and results in JDBC involves creating statements, executing queries or updates, processing the results, and properly closing resources. By following these practices, you can ensure efficient and secure interaction with your database.