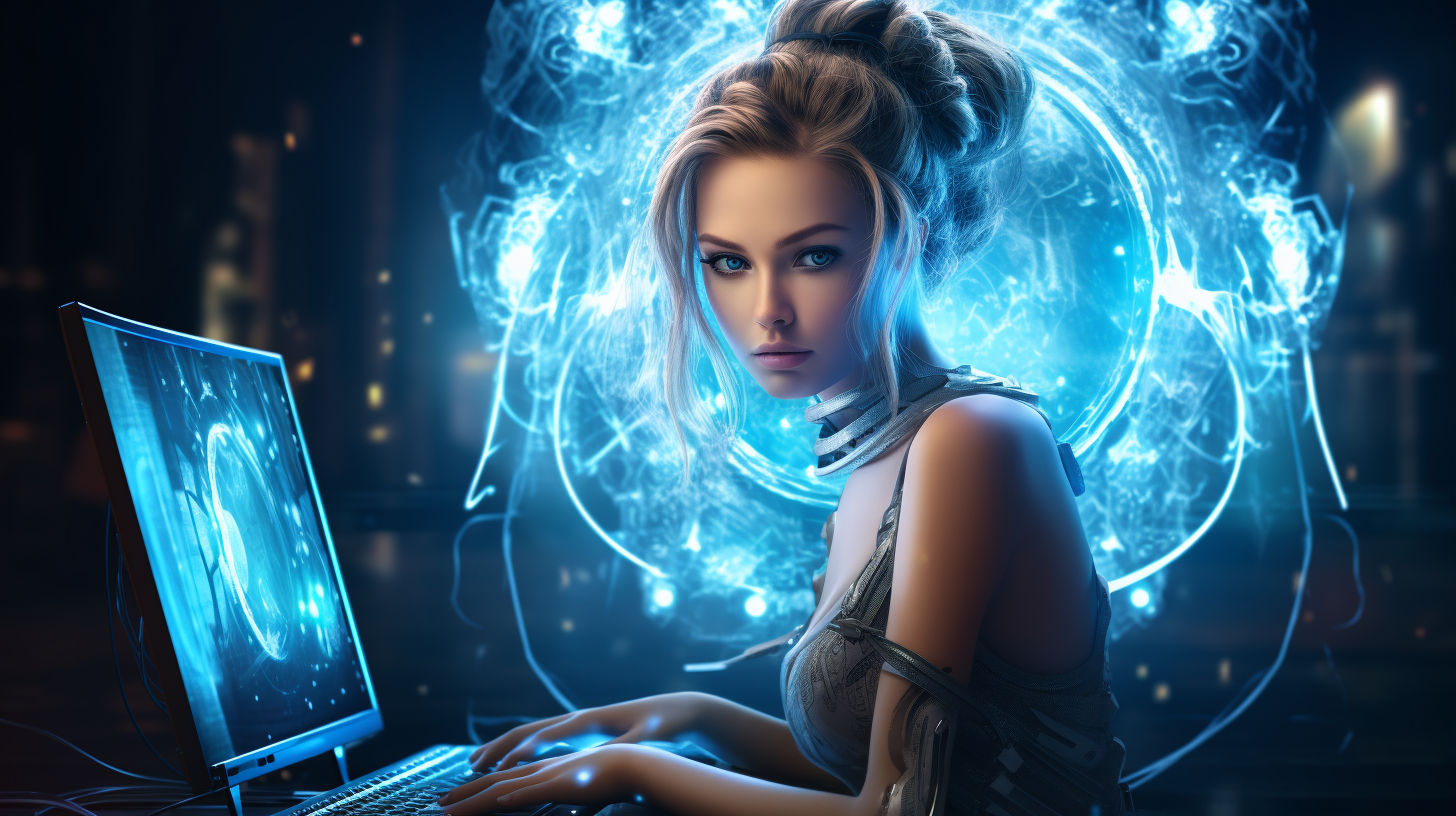
Java and Gradle: Build Automation
Introduction to Java and Gradle
Java is a widely-used programming language that’s known for its portability, performance, and robustness. It is a language this is used to build a variety of applications, from web applications to mobile apps and large-scale enterprise systems. Java is an object-oriented language this is designed to be easy to write, compile, test, and debug.
Gradle, on the other hand, is an open-source build automation tool that’s designed to be flexible enough to build almost any type of software. It uses a Groovy-based domain-specific language (DSL) for declaring project configurations, which makes it more expressive and concise than XML-based build scripts used by Maven. Gradle integrates seamlessly with the Java ecosystem, including support for compiling Java code, running Java tests, and packaging Java applications.
When working with Java, Gradle offers a significant advantage because it automates the build process. This means that repetitive tasks such as compilation, packaging, and testing are handled by Gradle, which can significantly speed up the development process. For example, to compile a simple Java project using Gradle, you would use the following build script:
apply plugin: 'java' repositories { mavenCentral() } dependencies { testCompile 'junit:junit:4.12' }
This script tells Gradle to apply the Java plugin, which adds tasks for compiling Java code, running tests, and creating a JAR file. The repositories block tells Gradle where to find the dependencies that your project needs. In this case, it is using Maven Central, which is a popular repository for Java libraries. The dependencies block declares a single dependency for JUnit, which is a common testing framework for Java.
Gradle also offers incremental builds, which means that it only recompiles parts of the code that have changed since the last build, which can save a lot of time. Additionally, Gradle’s build cache can reuse outputs from previous builds, making subsequent builds even faster.
In summary, using Java in conjunction with Gradle can streamline the build process, making it faster and more efficient. By automating repetitive tasks and leveraging features like incremental builds and build caching, developers can spend more time writing code and less time managing the build process.
Benefits of Build Automation with Gradle
One of the primary benefits of build automation with Gradle is increased productivity. By automating the build process, developers can eliminate manual tasks that are both time-consuming and prone to errors. Gradle’s incremental build feature is a perfect example of how automation can save time. Instead of recompiling the entire codebase, Gradle intelligently determines which parts of the code have changed and only recompiles those sections. This can significantly reduce the build time, especially for large projects.
Another benefit of using Gradle for build automation is its flexibility and configurability. Gradle scripts are written in Groovy, a powerful and expressive language that allows developers to write clean and maintainable build scripts. This makes it easy to customize the build process to fit the specific needs of a project. For instance, if you need to create different types of JAR files for various deployment scenarios, you can easily configure Gradle to handle this with a few lines of code:
task customJar(type: Jar) { from sourceSets.main.output archiveClassifier = 'custom' }
Gradle’s dependency management is also a significant advantage. With its declarative dependency syntax, developers can easily manage and update library versions without manually downloading and managing JAR files. This ensures that projects are always using the latest and most secure versions of dependencies. Here’s an example of how to declare dependencies in Gradle:
dependencies { implementation 'org.apache.commons:commons-lang3:3.10' testImplementation 'junit:junit:4.12' }
Moreover, Gradle integrates well with Continuous Integration (CI) and Continuous Deployment (CD) tools, which are essential for state-of-the-art software development practices. With Gradle, you can easily set up automated builds and tests that run on every code check-in, ensuring that your codebase is always in a deployable state.
Lastly, Gradle’s plugin ecosystem extends its capabilities even further. There are plugins for code quality checks, static analysis, code coverage, and more. This means that developers can integrate additional tools into their build process with minimal effort.
To wrap it up, build automation with Gradle offers a multitude of benefits that streamline the development process. From increased productivity and flexibility to better dependency management and integration with CI/CD tools, Gradle helps developers focus on what they do best: writing great code.
How to Implement Java Build Automation with Gradle
Implementing Java build automation with Gradle involves a few key steps, which are important to streamline your development process. The first step is to set up your Gradle project by creating a build.gradle file in your project’s root directory. This file will contain all the necessary configurations for your project.
apply plugin: 'java' repositories { mavenCentral() } dependencies { implementation 'com.google.guava:guava:29.0-jre' testImplementation 'junit:junit:4.12' }
As shown in the code example above, you start by applying the Java plugin, which adds tasks like compileJava and test to your project. Next, you specify where Gradle should look for the dependencies your project requires. In most cases, mavenCentral() will suffice. Finally, you define your project’s dependencies within the dependencies block.
Once your build.gradle file is set up, you can run various commands to automate your build process. For instance, the command gradle build will compile your code, run tests, and package your application into a JAR file. If you only want to compile your code without running tests or creating a JAR, you can use gradle assemble.
To further optimize the build process, you can enable the Gradle Daemon, which is a long-lived background process that makes subsequent builds much faster. You can do this by adding the following line to your gradle.properties file:
org.gradle.daemon=true
If you want to create custom tasks, Gradle enables you to do so with ease. For example, if you need a task to clean up build directories, you could add the following to your build.gradle file:
task clean(type: Delete) { delete rootProject.buildDir }
In conclusion, implementing Java build automation with Gradle is straightforward and provides a robust and flexible way to manage your build process. With a properly configured build.gradle file and an understanding of Gradle’s command-line interface, you can automate tasks such as compilation, testing, and packaging, and ultimately save time and reduce potential errors in your development workflow.