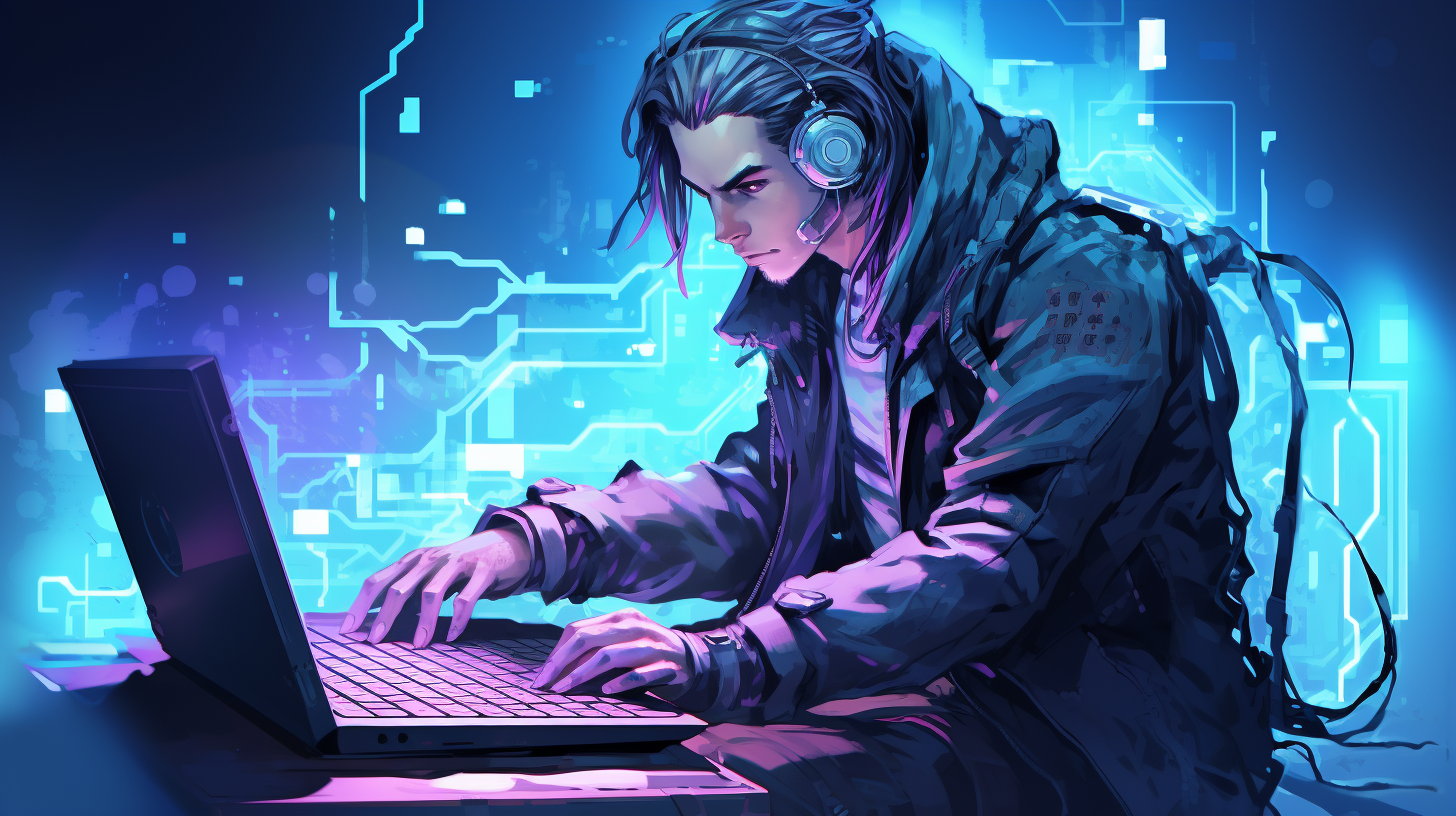
Managing NULL Values in SQL Queries
When working with SQL databases, you’ll often encounter NULL values. A NULL value in SQL represents missing or unknown data. It is important to understand how to manage NULL values in SQL queries to avoid unexpected results or errors. In this tutorial, we will discuss various techniques to handle NULL values in SQL.
Understanding NULL Values
NULL is not the same as zero or an empty string. NULL represents a lack of value. When comparing a NULL value with another value using comparison operators like =
, <
, >
, the result is always UNKNOWN. That is why we must use the IS NULL
or IS NOT NULL
operator to check for NULL values.
SELECT * FROM table_name WHERE column_name IS NULL;
SELECT * FROM table_name WHERE column_name IS NOT NULL;
Using COALESCE to Manage NULLs
The COALESCE
function in SQL can be used to return the first non-NULL value in a list. That is particularly useful when you want to replace NULL with a default value.
SELECT COALESCE(column_name, 'default_value') FROM table_name;
Using ISNULL to Replace NULL
Similar to COALESCE
, the ISNULL
function can be used to replace NULL with a specific value. However, ISNULL
is specific to SQL Server and only allows for one replacement value.
SELECT ISNULL(column_name, 'default_value') FROM table_name;
Using CASE Statement to Manage NULLs
The CASE
statement can also be used to handle NULL values. This allows for more complex logic to determine the replacement value.
SELECT CASE WHEN column_name IS NULL THEN 'default_value' ELSE column_name END FROM table_name;
Aggregating NULL Values
When using aggregation functions like SUM
, AVG
, MAX
, MIN
, SQL automatically excludes NULL values from the calculation. If you want to include NULL values as zero in the calculation, you must use COALESCE
or ISNULL
.
SELECT SUM(COALESCE(column_name, 0)) FROM table_name;
Handling NULLs in JOIN Conditions
When joining tables, if a column contains NULL values, the JOIN will not be able to match those NULLs with any value, possibly leading to missed data. To include rows with NULL values, use an OUTER JOIN
.
SELECT * FROM table1 LEFT OUTER JOIN table2 ON table1.column_name = table2.column_name;
Managing NULL values in SQL queries very important for achieving the desired results and avoiding errors. By understanding and properly using SQL functions like COALESCE
and ISNULL
, as well as operators like IS NULL
and IS NOT NULL
, one can handle NULL values effectively. Always remember to take NULL values into account while applying JOIN operations and aggregations, to ensure correct and comprehensive data handling.