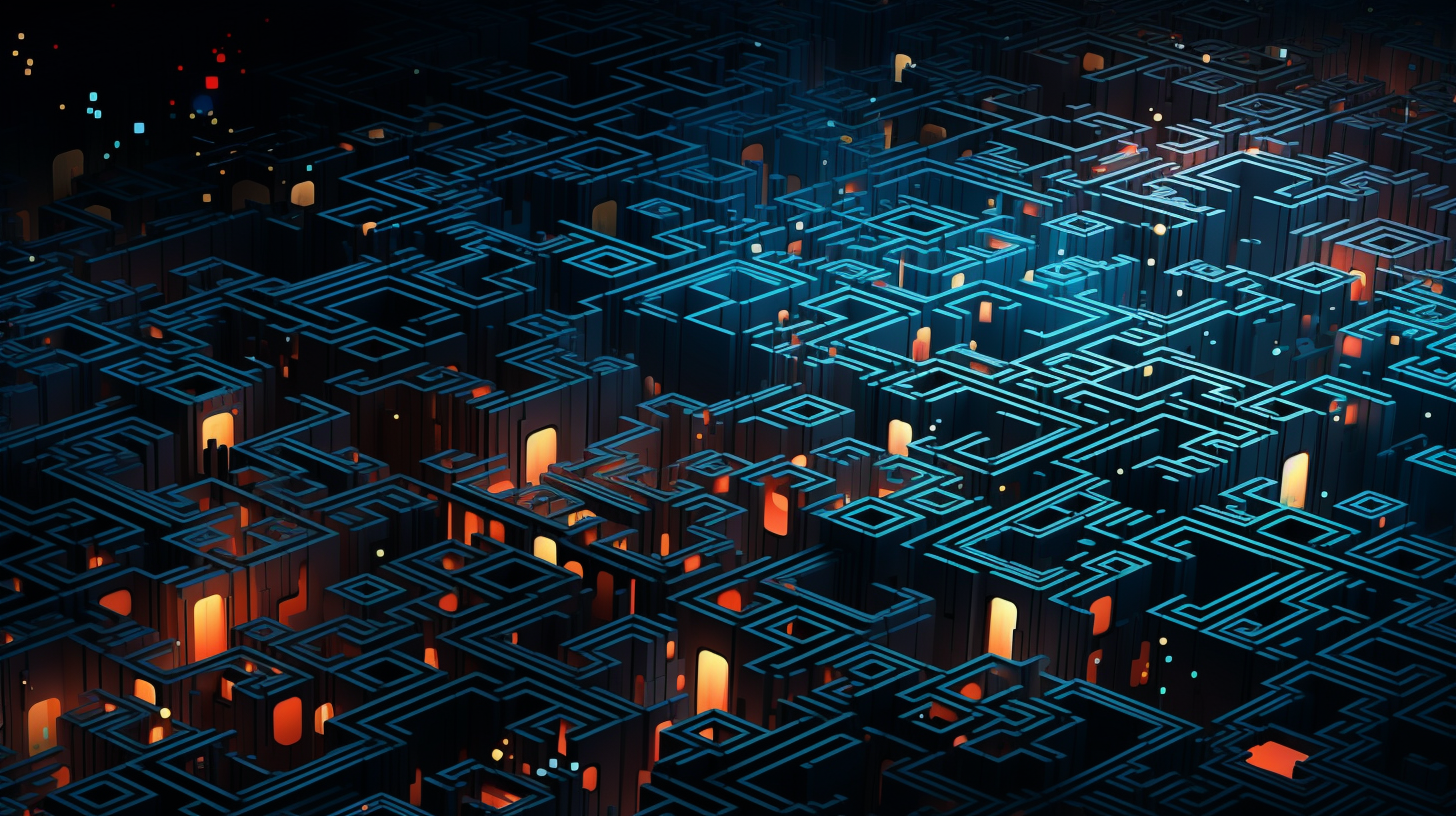
Understanding Variables in JavaScript
Introduction to Variables
In JavaScript, variables are containers for storing data values. They are one of the fundamental building blocks of the language, allowing developers to hold data, manipulate it, and pass it around different parts of the program. Understanding variables is important for anyone looking to master JavaScript.
A variable in JavaScript can be declared using one of three keywords: var, let, or const. Each of these keywords has a different implication on the scope, reassignment, and hoisting behavior of the variable it declares.
- Declares a variable, optionally initializing it to a value.
- Declares a block-scoped local variable, optionally initializing it to a value.
- Declares a block-scoped, read-only named constant.
Once a variable is declared, you can assign a value to it using the assignment operator (=
). For example:
var myName; // declaration myName = 'Nick Johnson'; // assignment let age; age = 30; const pi = 3.14; // declaration and assignment
It is important to note that JavaScript is a dynamically typed language, which means you do not need to specify the type of the variable when declaring it. The type of the variable will be determined by the value it holds.
Variables are one of the most basic, yet powerful concepts in programming. They allow developers to write flexible and dynamic code that can handle different types of data.
Declaring Variables
When declaring variables in JavaScript, it’s important to understand the implications of each keyword used. The first keyword, var, has been around since the inception of JavaScript. It declares a variable with function scope, meaning it’s accessible within the function it is defined, or globally if declared outside of a function. However, one of the downsides of var
is that it is susceptible to hoisting, which can lead to unexpected behavior in your code.
var greeting = 'Hello, world!'; console.log(greeting); // Outputs: Hello, world!
The second keyword, let, was introduced in ES6 (ECMAScript 2015) to address some of the issues with var
. It declares a block-scoped local variable, meaning it is only accessible within the block it is defined, such as a loop or an if statement. This makes let
a better choice for controlling the variable’s scope and avoiding hoisting issues.
let age = 25; if (true) { let age = 30; console.log(age); // Outputs: 30 } console.log(age); // Outputs: 25
The third keyword, const, also introduced in ES6, is used to declare variables that are not meant to be re-assigned. A const
variable must be initialized at the time of declaration, and it is also block-scoped. Attempting to re-assign a const
variable will result in a runtime error, ensuring the integrity of the data it holds.
const pi = 3.14159; console.log(pi); // Outputs: 3.14159 // This will throw an error // pi = 3.14;
It’s important to note that while const
prevents re-assignment of the variable, it does not make the value it holds immutable. For instance, if the const
variable holds an object, the properties of that object can still be changed.
const person = { name: 'Alice' }; person.name = 'Bob'; // That is allowed console.log(person.name); // Outputs: Bob // This will throw an error // person = { name: 'Charlie' };
Variable Scope
Understanding the scope of a variable especially important in JavaScript as it determines where that variable can be accessed and modified within your code. There are two main types of scope in JavaScript: global scope and local scope.
Global Scope: A variable is in the global scope if it’s declared outside of any function or block. This means it can be accessed and modified from any part of the code, including inside functions and blocks.
var globalVar = "I'm a global variable"; function logGlobalVar() { console.log(globalVar); // Outputs: I'm a global variable } logGlobalVar();
Local Scope: A variable is in the local scope if it is declared within a function or a block. It can only be accessed and modified within that function or block. If you try to access it from outside, you’ll get an error indicating that the variable is not defined.
function logLocalVar() { var localVar = "I'm a local variable"; console.log(localVar); // Outputs: I'm a local variable } logLocalVar(); console.log(localVar); // Uncaught ReferenceError: localVar is not defined
With ES6, JavaScript introduced let
and const
which provide block scoping. This means that a variable declared with let
or const
inside a block (e.g., if
, for
, while
), is only accessible within that block and not outside of it.
if (true) { let blockScopedVar = "I'm block scoped"; console.log(blockScopedVar); // Outputs: I'm block scoped } console.log(blockScopedVar); // Uncaught ReferenceError: blockScopedVar is not defined
It’s also important to understand function scoping, which is specific to variables declared with var
. A variable declared with var
inside a function is scoped to that function and can’t be accessed outside of it.
function myFunction() { var functionScopedVar = "I'm function scoped"; console.log(functionScopedVar); // Outputs: I'm function scoped } myFunction(); console.log(functionScopedVar); // Uncaught ReferenceError: functionScopedVar is not defined
However, if you declare a variable with var
inside a block (and not a function), it does not get block scope; it is still accessible outside of that block, which can lead to unexpected behavior and is one of the reasons why let
and const
are now preferred over var
.
for (var i = 0; i < 3; i++) { console.log(i); // Outputs: 0, 1, 2 } console.log(i); // Outputs: 3, since `i` is function-scoped and not block-scoped
Data Types in Variables
In JavaScript, each variable holds a value of a specific data type. The language is loosely typed, which means that you don’t have to declare the type of the variable when you declare it, and the variable’s type can change based on the value it is assigned. There are several basic data types in JavaScript, including:
- Represents textual data. It’s how you work with text in JavaScript. Example:
var name = "John";
- Represents both integer and floating-point numbers. Example:
var age = 25;
orvar price = 99.99;
- Represents a logical entity and can have two values: true or false. Example:
var isStudent = true;
- A variable that has been declared but not assigned a value is of type undefined. Example:
var a;
- Represents the intentional absence of any object value. It’s one of JavaScript’s primitive values. Example:
var empty = null;
- Represents instances of objects, which can be a collection of properties. Example:
var person = {firstName: "Jane", lastName: "Doe"};
- A unique and immutable primitive introduced in ES6 for unique property keys. Example:
var sym = Symbol('description');
Additionally, ES6 introduced two more useful data types:
- Represents numbers larger than 2^53 – 1, which is the largest number JavaScript can reliably represent with the Number type. Example:
var bigNumber = 1234567890123456789012345678901234567890n;
- Although technically a type of object, arrays are a special kind of object suited to storing ordered collections. Example:
var colors = ['Red', 'Green', 'Blue'];
Here are some examples of how these data types can be used in JavaScript:
// String var greeting = "Hello, World!"; // Number var score = 100; // Boolean var isApproved = false; // Undefined var notAssigned; // Null var noValue = null; // Object var user = { username: "johndoe", password: "123456" }; // Symbol var mySymbol = Symbol('mySymbol'); // BigInt var largeNumber = 9007199254740992n; // Array var fruits = ["Apple", "Banana", "Cherry"];
Understanding the different data types is important because it affects how you perform operations on your variables. For instance, you can’t perform mathematical operations on strings (unless you are concatenating them), and you can’t treat numbers like text without converting them first.
JavaScript also provides various methods and properties to work with these data types, such as .length
for strings and arrays, or .toFixed()
for numbers.
Manipulating Variables
Manipulating variables in JavaScript involves various operations such as assignment, arithmetic, comparison, and logical operations. These operations allow you to change the value of variables, perform calculations, and make decisions in your code based on variable values.
To manipulate variables, you can use the assignment operator (=
) to change the value of a variable after it has been declared. For example:
let count = 10; count = 15; // reassigning the value of count to 15
In addition to assignment, you can perform arithmetic operations on variables that hold number values. These operations include addition (+
), subtraction (-
), multiplication (*
), division (/
), and modulus (%
).
let a = 5; let b = 2; let sum = a + b; // 7 let difference = a - b; // 3 let product = a * b; // 10 let quotient = a / b; // 2.5 let remainder = a % b; // 1
You can also use the increment (++
) and decrement (--
) operators to increase or decrease a variable’s value by one.
let score = 0; score++; // score is now 1 score--; // score is now 0
Comparison operators, such as greater than (>
), less than (<
), equal to (==
), and not equal to (!=
), can be used to compare two variables and return a Boolean value (true
or false
).
let x = 10; let y = 5; console.log(x > y); // true console.log(x < y); // false console.log(x == y); // false console.log(x != y); // true
Logical operators such as AND (&&
), OR (||
), and NOT (!
) can be used to combine or invert Boolean values.
let isAdult = true; let hasPermission = false; console.log(isAdult && hasPermission); // false console.log(isAdult || hasPermission); // true console.log(!isAdult); // false
JavaScript also allows for string concatenation using the addition operator (+
). This operation combines two strings into one.
let firstName = "John"; let lastName = "Doe"; let fullName = firstName + " " + lastName; // "Neil Hamilton"
Lastly, variables holding objects or arrays can be manipulated by accessing and modifying their properties or elements.
let user = { name: "Alice", age: 28 }; user.age = 29; // updating the age property let colors = ["Red", "Green", "Blue"]; colors[1] = "Yellow"; // changing the second element from Green to Yellow
One important aspect that the article doesn’t touch on is the concept of variable hoisting in JavaScript. That is the behavior where variable declarations are moved to the top of their containing scope during the compilation phase, which can lead to unexpected results if not properly understood. For instance, a variable declared with var can be used before its actual declaration in the code, because it’s hoisted to the top of its scope. This can cause confusion and bugs, so it’s something that developers need to be aware of.