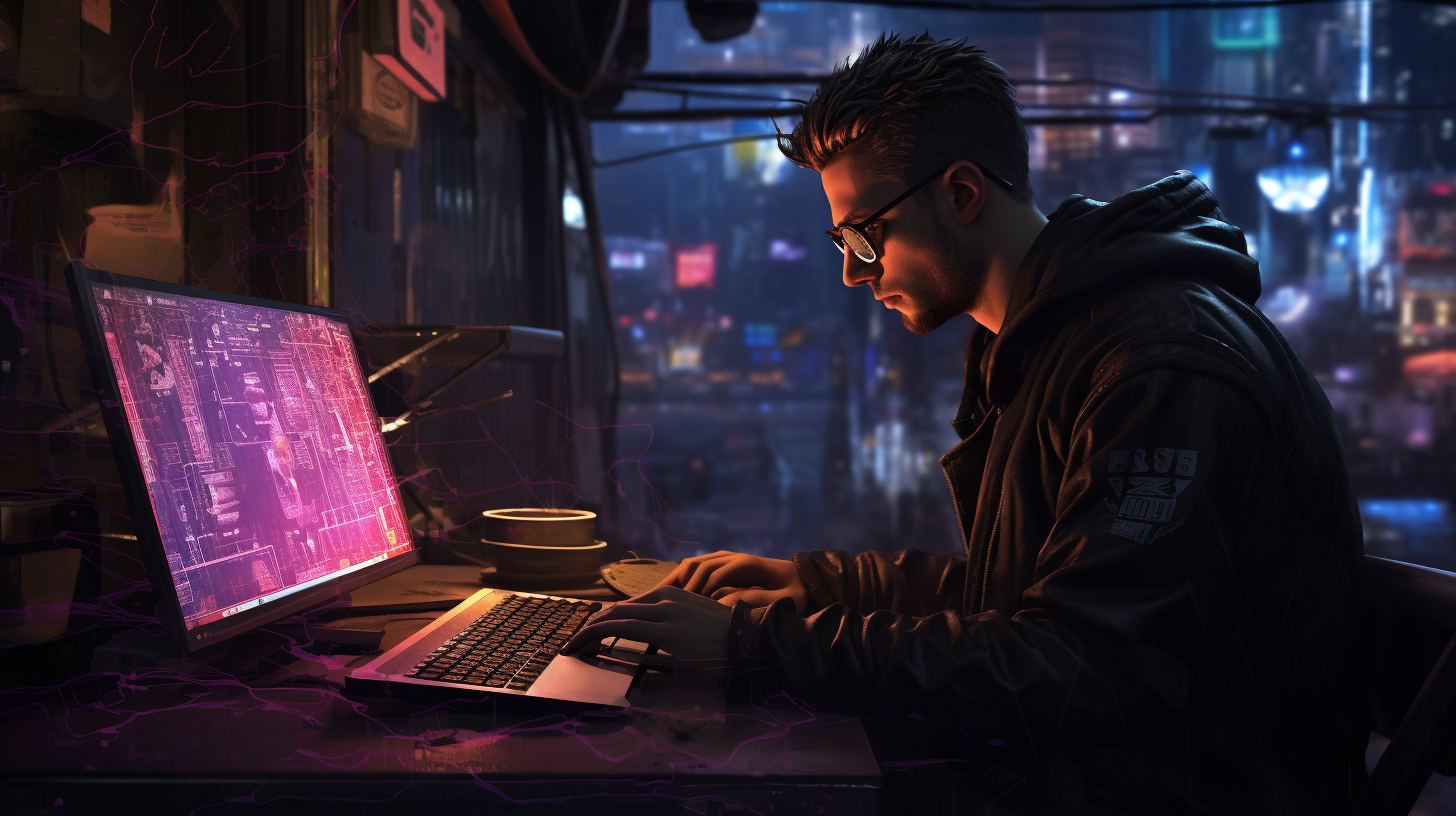
PHP and CRON Jobs: Scheduling Tasks
Understanding CRON Jobs
CRON jobs are a powerful feature of Unix-based operating systems, including Linux and macOS, that allow users to schedule tasks to run automatically at specified intervals. The name “CRON” comes from the Greek word “chronos,” meaning time, which is fitting since these jobs are all about automating tasks based on time.
The CRON daemon is a background process that runs on the system and checks for scheduled tasks, or “jobs,” to execute. These tasks are defined in a crontab file, which is a simple text file that contains a list of commands and the times at which they should be run.
A typical crontab entry consists of six fields:
- The minute of the hour when the command should run (0-59)
- The hour of the day when the command should run (0-23)
- The day of the month when the command should run (1-31)
- The month of the year when the command should run (1-12)
- The day of the week when the command should run (0-7, where both 0 and 7 represent Sunday)
- The command to execute
Each field can contain a single value, a range of values, a list of values, or a special character that represents a recurring value. An asterisk (*) represents all possible values for a field, meaning the command will run every time that field cycles. For example, an asterisk in the minute field means the command will run every minute.
Here’s an example of a crontab entry that runs a PHP script every day at midnight:
0 0 * * * /usr/bin/php /path/to/your/script.php
CRON jobs are particularly useful for automating repetitive tasks such as data backups, sending emails, or cleaning up log files. By using CRON jobs, developers can ensure that these tasks are performed consistently and without manual intervention.
It is important to note that the crontab file should be edited with caution, as incorrect entries can lead to unexpected behavior or system issues. It is recommended to use the crontab -e
command to edit the crontab file, which opens the file in the default text editor and checks for syntax errors upon saving.
Remember: Always double-check your crontab entries to ensure they’re correct and won’t cause any unintended consequences on your system.
Setting Up CRON Jobs in PHP
Setting up CRON jobs in PHP requires a few steps. First, you need to write the PHP script that you want to execute at a certain time. Once your script is ready, you can then schedule the CRON job using the crontab.
To begin, open your terminal and type crontab -e
. This will open your crontab file in your default text editor. If you’re using this for the first time, you might be prompted to select a text editor before the file opens.
Next, add a new line to the bottom of the crontab file with the schedule and the command to run your PHP script. The format should match the typical crontab entry with six fields as mentioned previously. Here is an example of how to schedule a PHP script to run every day at 3am:
0 3 * * * /usr/bin/php /path/to/your/script.php
Make sure to replace /usr/bin/php
with the path to the PHP CLI executable on your system, and /path/to/your/script.php
with the path to your PHP script.
After adding your scheduled task, save and close the crontab file. The CRON daemon will automatically pick up the changes to the crontab file and begin executing your script at the scheduled time.
It is important to ensure that your PHP script is executable and that it has the appropriate permissions. You can make your script executable by running the following command:
chmod +x /path/to/your/script.php
Finally, it is a good practice to log the output of your CRON jobs so you can monitor their execution. You can redirect the output to a log file by modifying your crontab entry like so:
0 3 * * * /usr/bin/php /path/to/your/script.php >> /path/to/your/logfile.log 2>&1
In this example, >>
appends the output to the logfile, and 2>&1
ensures that both standard output and standard error are redirected to the log file.
With these steps, you have successfully set up a CRON job to run a PHP script at a scheduled time. This allows you to automate tasks and ensure they run consistently without the need for manual intervention.
Common CRON Job Tasks
CRON jobs can be used for a variety of common tasks that need to be run on a schedule. Here are some examples of tasks that can be automated with CRON:
- Regularly backing up your database especially important for data integrity and recovery. You can schedule a CRON job to run a PHP script that dumps your database to a file and stores it in a secure location.
// Database backup script $database = 'my_database'; $user = 'my_user'; $password = 'my_password'; $backupFile = 'backup-' . date('Y-m-d') . '.sql'; $command = "mysqldump --user={$user} --password={$password} {$database} > {$backupFile}"; exec($command);
// Email reminder script mail('[email protected]', 'Reminder', 'Don't forget your appointment tomorrow!');
// Report generation script // Code to generate report $report = generateReport(); mail('[email protected]', 'Weekly Report', $report);
// Log cleanup script $logFile = '/path/to/your/logfile.log'; if (file_exists($logFile) && filesize($logFile) > 1000000) { // Archive and truncate log file $archiveFile = $logFile . '.' . date('Y-m-d'); rename($logFile, $archiveFile); file_put_contents($logFile, ''); }
// Content update script // Code to fetch new content and update the website updateContent();
These are just a few examples of the many tasks that can be automated with CRON jobs. By identifying repetitive or time-based tasks within your applications, you can use CRON to ensure they are handled efficiently and without manual intervention.
Troubleshooting CRON Job Issues
When working with CRON jobs, it’s not uncommon to encounter issues that prevent your scheduled tasks from running as expected. Troubleshooting these issues can be a bit tricky, but there are several common areas to check that can help you identify and resolve problems quickly.
- Ensure that the syntax in your crontab is correct. A missing or misplaced character can cause the CRON job to fail. Double-check the time and date fields, as well as the command itself.
- Your PHP script must have the appropriate execute permissions. Use
ls -l /path/to/your/script.php
to check the permissions andchmod +x /path/to/your/script.php
to make it executable if needed. - CRON jobs can generate output that’s sent to the system’s mail by default. Check the mail for the user under which the CRON job is running, or review the log file if you’ve set up output redirection in the crontab.
- Run your PHP script manually from the command line to ensure it executes without errors. This can help you identify any issues within the script itself.
- CRON jobs run in a different environment than the user’s interactive shell. Environment variables that are available in the shell may not be present for a CRON job. You may need to explicitly set necessary environment variables in the script or the crontab entry.
Here’s an example of a PHP script that checks for common CRON job issues:
// Check for CRON job issues $scriptPath = '/path/to/your/script.php'; $permissions = substr(sprintf('%o', fileperms($scriptPath)), -4); if ($permissions !== '0755') { echo "Script does not have execute permissions.n"; echo "Run 'chmod +x {$scriptPath}' to fix.n"; } // Test script execution $output = []; $returnVar = 0; exec("/usr/bin/php {$scriptPath}", $output, $returnVar); if ($returnVar !== 0) { echo "Script did not execute successfully.n"; echo "Check the script for errors.n"; } // Check for CRON environment variables $envVars = ['PATH', 'HOME', 'SHELL']; foreach ($envVars as $envVar) { if (!getenv($envVar)) { echo "Environment variable '{$envVar}' is not set for CRON.n"; echo "Set this variable in the crontab or script.n"; } }
If you’ve checked all the common issues and your CRON job still isn’t working, it may be necessary to dig deeper into the system logs or consult with a system administrator for further assistance.
By methodically checking each potential issue, you can quickly identify and resolve problems with your CRON jobs, ensuring that your scheduled tasks run smoothly and reliably.
Best Practices for Scheduling Tasks
When it comes to scheduling tasks with CRON jobs, following best practices can help ensure that your automated tasks run efficiently and without errors. Here are some key best practices to consider:
- Always use absolute paths for both the PHP executable and the script you’re running. This removes any ambiguity about where the files are located and ensures that CRON can find them.
0 3 * * * /usr/bin/php /absolute/path/to/your/script.php
- Ensure that your tasks can handle overlapping runs. If a task takes longer than expected, the next scheduled run may start before the previous one finishes. Design your scripts to handle this scenario gracefully.
// Check if script is already running $lockFile = '/tmp/script.lock'; if (file_exists($lockFile)) { echo "Script is already running.n"; exit; } touch($lockFile); // Your task logic here // Remove lock file after completion unlink($lockFile);
- Always log the output of your CRON jobs. This will help you troubleshoot any issues and keep track of what the job is doing. Use output redirection in the crontab to send output to a log file.
0 3 * * * /usr/bin/php /path/to/your/script.php >> /path/to/your/logfile.log 2>&1
- Don’t schedule jobs more frequently than necessary. If a task only needs to run once a day, don’t schedule it to run every hour. This reduces the load on your server and prevents unnecessary executions.
- Your scripts should include proper error handling to catch and log exceptions or issues that occur during execution. This practice helps in identifying problems early and fixing them.
try { // Your task logic here } catch (Exception $e) { // Log exception file_put_contents('/path/to/your/error.log', $e->getMessage(), FILE_APPEND); }
- Add comments to your crontab entries to explain what each job does. That is especially helpful when you have multiple jobs scheduled and need to keep track of their purposes.
# Daily database backup at 3am 0 3 * * * /usr/bin/php /path/to/your/backup_script.php >> /path/to/your/backup.log 2>&1
- Regularly monitor your CRON jobs to ensure they’re running as expected. Setting up monitoring tools or scripts can alert you to any failures or performance issues.
By following these best practices, you can create a robust and reliable system for scheduling tasks with CRON jobs in PHP. Remember, the key to successful task automation is careful planning, thorough testing, and ongoing monitoring.
One important aspect to ponder when setting up CRON jobs is the impact on server resources. It is essential to monitor server resource usage, such as CPU and memory, to ensure that the scheduled tasks do not lead to performance degradation, especially when running resource-intensive scripts. Additionally, it is a good practice to implement error notification mechanisms, like email alerts, which can inform the administrator immediately if a CRON job fails to execute or encounters issues. This proactive approach helps maintain system stability and allows for quick resolution of any problems that may arise.