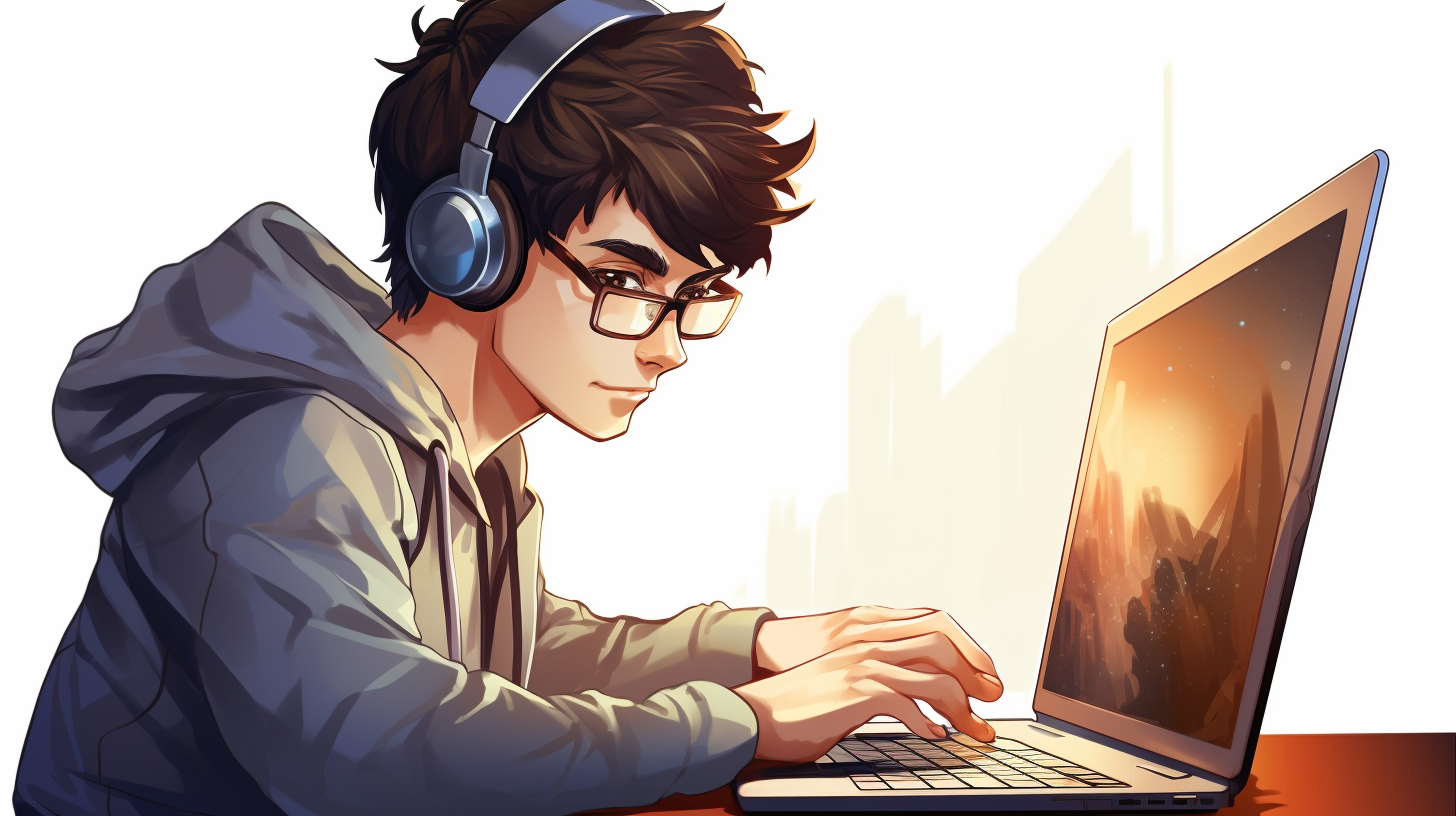
Bash in Scientific Computing
Bash, short for “Bourne Again SHell”, is a command-line language and shell that’s widely used in scientific computing. It provides developers with a powerful and flexible toolset for performing calculations, data analysis, and automation tasks. In this tutorial, we will explore how Bash can be utilized in scientific computing, focusing on its capabilities for calculations and data analysis.
Performing Calculations with Bash
Bash provides various arithmetic operators and functions that allow for performing calculations directly from the command line or within a Bash script. Here are a few examples:
- Addition
- Subtraction
- Multiplication
- Division
- Modulo (remainder of division)
- Exponentiation
Let’s see an example of using these operators in Bash:
#!/bin/bash x=5 y=10 # Addition result=$((x + y)) echo "Addition: $result" # Subtraction result=$((y - x)) echo "Subtraction: $result" # Multiplication result=$((x * y)) echo "Multiplication: $result" # Division result=$((y / x)) echo "Division: $result" # Modulo result=$((y % x)) echo "Modulo: $result" # Exponentiation result=$((x ** 2)) echo "Exponentiation: $result"
When executing the above script, the calculated results will be printed to the console:
Addition: 15 Subtraction: 5 Multiplication: 50 Division: 2 Modulo: 0 Exponentiation: 25
Bash also provides functions for more advanced mathematical operations, including square root, logarithm, and trigonometric functions. These functions can be used by invoking them with the appropriate arguments. For example:
#!/bin/bash x=4 y=2 # Square root result=$(bc -l <<< "sqrt($x)") echo "Square root: $result" # Logarithm result=$(bc -l <<< "l($x)") echo "Natural logarithm: $result" # Trigonometric function - Sine result=$(bc -l <<< "s($y)") echo "Sine: $result"
The bc -l
command is used here to provide a precise mathematical calculation environment. It reads the mathematical expressions passed to it and evaluates them accordingly.
Data Analysis with Bash
In addition to calculations, Bash can be used for data analysis tasks, such as processing and manipulating data files. Bash provides powerful string manipulation capabilities, file input/output operations, and command-line tools that are useful for these purposes.
Let’s consider an example where we have a CSV file containing data points, and we want to calculate the average value of a specific column:
#!/bin/bash # Read the CSV file filename="data.csv" # Column index for data column=2 # Process the data and calculate the average sum=0 count=0 while IFS=',' read -r line; do # Extract the value from the desired column value=$(echo "$line" | cut -d',' -f$column) # Accumulate the sum sum=$((sum + value)) # Increase the count count=$((count + 1)) done < "$filename" # Calculate the average average=$((sum / count)) echo "Average: $average"
In the above script, each line of the CSV file is read and processed. The desired column’s value is extracted using the cut
command, and the sum and count variables are updated accordingly. Finally, the average is calculated by dividing the sum by the count.
Bash also integrates well with other command-line tools commonly used in scientific computing, such as awk
and grep
. These tools provide additional capabilities for filtering, manipulating, and analyzing data. For example, we can use awk
to calculate the maximum value in a specific column:
#!/bin/bash # Read the CSV file filename="data.csv" # Column index for data column=3 # Calculate the maximum value using awk max_value=$(awk -F',' '{ if(max=="") max=$'$column'; else if($'$column'>max) max=$'$column'; } END { print max; }' "$filename") echo "Maximum value: $max_value"
In the above script, awk
processes the CSV file and checks each value in the desired column. It keeps track of the current maximum value encountered and updates it whenever a larger value is found. Finally, it prints the maximum value.
Bash is a versatile tool for scientific computing that allows for performing calculations and data analysis tasks efficiently. It supports arithmetic operations, mathematical functions, and provides powerful string manipulation capabilities. Combined with other command-line tools, Bash can become a valuable asset for scientific researchers and data analysts alike.