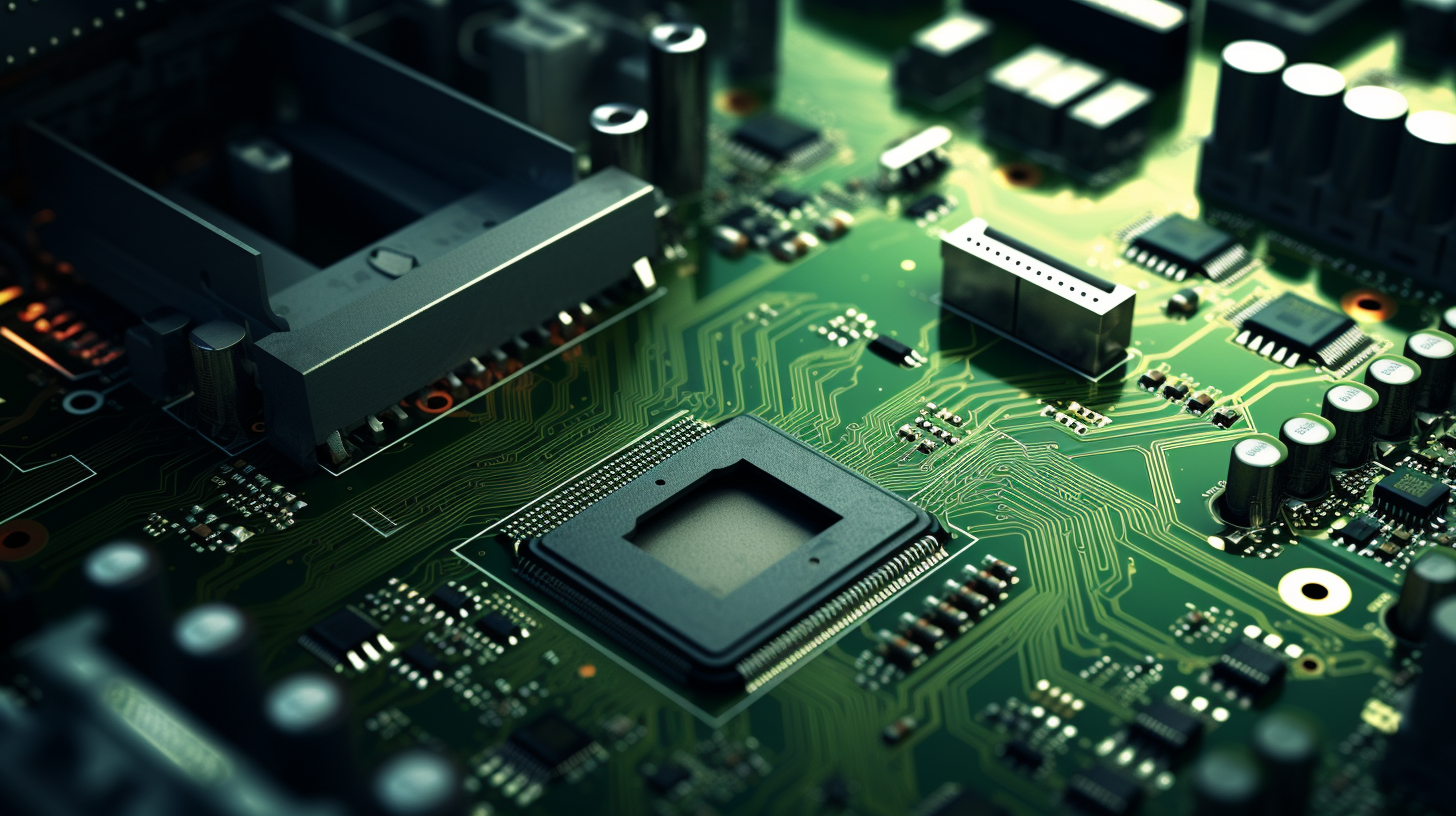
SQL Locking Mechanisms for Concurrency Control
Locking mechanisms in SQL play an important role in maintaining data integrity and consistency in multi-user environments. When multiple transactions are executed concurrently, SQL must manage access to shared resources to prevent anomalies such as dirty reads, non-repeatable reads, and phantom reads. Locks ensure that when one transaction is accessing a piece of data, other transactions cannot modify it until the first one has been completed.
At its core, a lock is a mechanism that restricts access to a resource, allowing only one transaction to modify it at a time. This is essential in databases where simultaneous operations may lead to inconsistent states. SQL databases typically implement two main types of locks: shared locks and exclusive locks.
- These locks allow multiple transactions to read a resource but prevent any transaction from modifying it. For example, if Transaction A holds a shared lock on a row, Transaction B can also acquire a shared lock on the same row but cannot modify it until Transaction A releases its lock.
- An exclusive lock allows a transaction to both read and modify a resource. When a transaction holds an exclusive lock on a resource, no other transaction can acquire any type of lock on that resource until the exclusive lock is released.
SQL databases use a combination of these locks to enforce isolation levels. The isolation level determines how transaction integrity is visible to other transactions and can range from fully isolated transactions to those that allow various levels of concurrency. Understanding the lock types and their implications on transaction behavior is essential for optimizing performance and ensuring data consistency.
Here’s an example demonstrating how to acquire a shared lock in SQL:
SELECT * FROM employees WITH (NOLOCK);
This query reads data from the employees table without acquiring shared locks, thus allowing other transactions to continue working with the table while this query executes. However, it could lead to reading uncommitted changes, which is why it’s often approached with caution.
Conversely, to acquire an exclusive lock, you can use the following SQL statement:
BEGIN TRANSACTION; UPDATE employees SET salary = salary * 1.10 WHERE department = 'Sales'; -- Exclusive lock is held until the transaction is committed or rolled back COMMIT;
In this case, the update statement acquires an exclusive lock on the rows being modified, ensuring that no other transactions can read or write to those rows until the transaction is completed.
Types of Locks: Shared, Exclusive, and More
Beyond shared and exclusive locks, SQL also implements other types of locks to address specific concurrency control scenarios. These include update locks, intent locks, and schema locks, each serving a unique purpose in the broader locking framework.
Update Locks: An update lock is a hybrid that serves both as a signal that a transaction intends to modify a resource and as a way to prevent deadlocks. When a transaction acquires an update lock, it indicates that it intends to acquire an exclusive lock later. That’s useful in situations where multiple transactions might want to update the same resource, as it allows them to signal their intention without immediately blocking each other. For example:
BEGIN TRANSACTION; SELECT * FROM employees WITH (UPDLOCK) WHERE department = 'Marketing'; -- Perform some calculations or checks UPDATE employees SET salary = salary * 1.05 WHERE department = 'Marketing'; COMMIT;
Here, the UPDLOCK
hint is used to acquire an update lock on the selected rows. This prevents other transactions from acquiring an exclusive lock on these rows until the current transaction is either committed or rolled back.
Intent Locks: Intent locks are used to indicate that a transaction intends to acquire locks on a finer granularity (like rows) down the line. They come in two flavors: intent shared (IS) and intent exclusive (IX). Intent locks are essential when dealing with table-level locking, as they allow the database engine to efficiently manage the locking hierarchy. For example:
BEGIN TRANSACTION; SELECT * FROM departments WITH (TABLOCKX); -- The transaction intends to acquire exclusive locks on rows in the departments table UPDATE departments SET budget = budget * 1.20 WHERE department_id = 1; COMMIT;
In this case, the table-level exclusive lock ensures that no other transactions can read or write to the departments
table while the budget update occurs.
Schema Locks: Schema locks are used when structural changes are made to the database schema, such as creating or dropping tables. They prevent other transactions from accessing the schema until the changes are complete, ensuring that no other transactions can interfere with ongoing schema modifications. An example of a schema lock would be:
BEGIN TRANSACTION; CREATE TABLE new_employees (id INT, name VARCHAR(100)); -- Schema lock held until the transaction is committed COMMIT;
Lock Granularity: Row-Level vs. Table-Level
Lock granularity refers to the level of detail at which locks are applied in a database, and it significantly impacts the performance and concurrency of transactions. The two primary types of lock granularity are row-level and table-level locks, each with its advantages and disadvantages. Understanding these concepts can help optimize database performance and ensure efficient transaction handling.
Row-level locking allows for a finer granularity of control, where locks are applied to individual rows within a table. This means that multiple transactions can work on different rows of the same table simultaneously without interfering with each other. Such a mechanism is particularly beneficial in high-concurrency environments, as it maximizes the number of transactions that can be processed in parallel.
For instance, think a scenario where two transactions are attempting to update different rows in the same table. With row-level locking, both updates can proceed without waiting for the other to finish. Here’s how this can be implemented:
BEGIN TRANSACTION; UPDATE employees SET salary = salary * 1.10 WHERE employee_id = 1; COMMIT; BEGIN TRANSACTION; UPDATE employees SET salary = salary * 1.15 WHERE employee_id = 2; COMMIT;
In this example, Transaction 1 updates the salary of the employee with ID 1 while Transaction 2 updates the salary of the employee with ID 2, allowing both operations to occur simultaneously without conflict.
On the other hand, table-level locking applies a lock to the entire table, preventing any other transactions from accessing the table until the lock is released. While this can simplify lock management and reduce the overhead associated with acquiring and releasing locks, it can lead to increased contention and reduced throughput in environments with high transaction volumes.
Consider this example of a table-level lock:
BEGIN TRANSACTION; UPDATE employees SET salary = salary * 1.20; -- This operation locks the entire employees table COMMIT;
In this case, while the salary update is being processed, no other transactions can read from or write to the employees table, potentially causing delays and reduced performance as other transactions queue up behind the lock.
Choosing between row-level and table-level locking depends on the specific needs and expected workload of the application. Row-level locking is generally preferable in high-concurrency scenarios, whereas table-level locking might be more suitable for operations that require comprehensive changes to a table’s data or structure.
Deadlocks: Detection and Resolution Strategies
Deadlocks are a critical concern in SQL concurrency control, especially in environments where multiple transactions are vying for the same resources. A deadlock occurs when two or more transactions are waiting indefinitely for each other to release locks, creating a cycle of dependency that prevents any of them from proceeding. This situation can severely impact database performance and responsiveness, making it essential to detect and resolve deadlocks efficiently.
To understand deadlock detection, think a simple scenario involving two transactions:
BEGIN TRANSACTION; UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; -- Transaction A holds a lock on account_id = 1 WAITFOR DELAY '00:00:05'; -- Simulate a delay UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; COMMIT;
BEGIN TRANSACTION; UPDATE accounts SET balance = balance + 100 WHERE account_id = 2; -- Transaction B holds a lock on account_id = 2 WAITFOR DELAY '00:00:05'; -- Simulate a delay UPDATE accounts SET balance = balance - 100 WHERE account_id = 1; COMMIT;
In this example, Transaction A is waiting for Transaction B to release its lock on account_id = 2, while Transaction B is waiting for Transaction A to release its lock on account_id = 1. This circular wait creates a deadlock situation.
SQL Server, like many database systems, employs various deadlock detection mechanisms. These mechanisms typically involve monitoring the lock manager to identify cycles in the wait-for graph, where transactions are waiting on each other. When a deadlock is detected, the database system must resolve it by terminating one of the transactions, allowing the other to proceed.
The transaction that’s chosen to be terminated is often referred to as the “deadlock victim.” It’s typically the one that has the least amount of work done or the one that can be rolled back with minimal impact on overall operation. Here’s how you might handle a deadlock in SQL Server:
BEGIN TRY BEGIN TRANSACTION; -- Execute your transaction logic here COMMIT; END TRY BEGIN CATCH IF (ERROR_NUMBER() = 1205) -- Deadlock error number BEGIN PRINT 'Deadlock detected. Transaction has been rolled back.'; ROLLBACK; END ELSE BEGIN -- Handle other errors PRINT 'An error occurred: ' + ERROR_MESSAGE(); ROLLBACK; END END CATCH;
In this example, a `TRY…CATCH` block is used to handle potential deadlock errors. If a deadlock is detected, the transaction is rolled back, and the application can retry the operation or log the incident for further analysis.
Beyond detection, the resolution of deadlocks can also be influenced by application design. Implementing best practices such as acquiring locks in a consistent order across transactions can significantly reduce the likelihood of deadlocks. Additionally, using lower isolation levels where appropriate can help minimize locking contention. For example:
SET TRANSACTION ISOLATION LEVEL READ COMMITTED; BEGIN TRANSACTION; -- Perform operations that require minimal locking COMMIT;
Moreover, applications can implement timeout strategies for transactions. If a transaction waits too long for a lock, it can be aborted, thereby preventing prolonged deadlocks. For instance:
SET LOCK_TIMEOUT 5000; -- Timeout in milliseconds BEGIN TRANSACTION; -- Attempt to perform operations COMMIT;
Optimistic vs. Pessimistic Concurrency Control
In the context of concurrency control, two predominant strategies emerge: optimistic concurrency control and pessimistic concurrency control. Each of these approaches has its unique methodologies and use cases, determined largely by the nature of application workloads and the level of contention for shared resources.
Optimistic concurrency control operates on the assumption that conflicts between transactions are rare. Thus, it allows transactions to execute without acquiring locks initially. Instead, it verifies whether a conflict has occurred at the time of committing the transaction. This mechanism is particularly beneficial in environments where read operations significantly outnumber write operations. In such situations, the overhead of locking resources is minimized, resulting in improved performance.
Here’s an example of how optimistic concurrency control can be implemented in SQL:
BEGIN TRANSACTION; -- Read the current state of the item SELECT * FROM products WHERE product_id = 1; -- Assuming we make some changes in application logic -- Update this product price optimistically UPDATE products SET price = price * 1.10 WHERE product_id = 1 AND version = @current_version; IF @@ROWCOUNT = 0 BEGIN PRINT 'Conflict detected: The product has been modified by another transaction.'; ROLLBACK; END ELSE BEGIN COMMIT; END
In this scenario, a versioning mechanism is employed. The transaction reads the current version of the record and attempts to update it only if the version remains unchanged. If another transaction has modified this record, the `@@ROWCOUNT` will indicate that no rows were updated, triggering a rollback and allowing the application to handle the conflict gracefully.
On the other hand, pessimistic concurrency control assumes that conflicts are likely and employs locks to prevent them proactively. This method can be particularly effective in high-contention scenarios where multiple transactions are attempting to modify the same data concurrently. By acquiring locks on resources at the beginning of a transaction, pessimistic control mitigates the risk of conflicts but may introduce significant overhead due to blocking.
Here’s an example of implementing pessimistic concurrency control:
BEGIN TRANSACTION; -- Acquire an exclusive lock on this product row UPDATE products WITH (ROWLOCK) SET price = price * 1.10 WHERE product_id = 1; -- This will block other transactions from accessing the row COMMIT;
In this case, an exclusive lock is placed on the row for the duration of the transaction, preventing any other transaction from accessing or modifying that row until the current transaction is completed. While this guarantees data integrity, it can introduce bottlenecks in high-concurrency environments, as transactions may end up waiting for each other to complete.