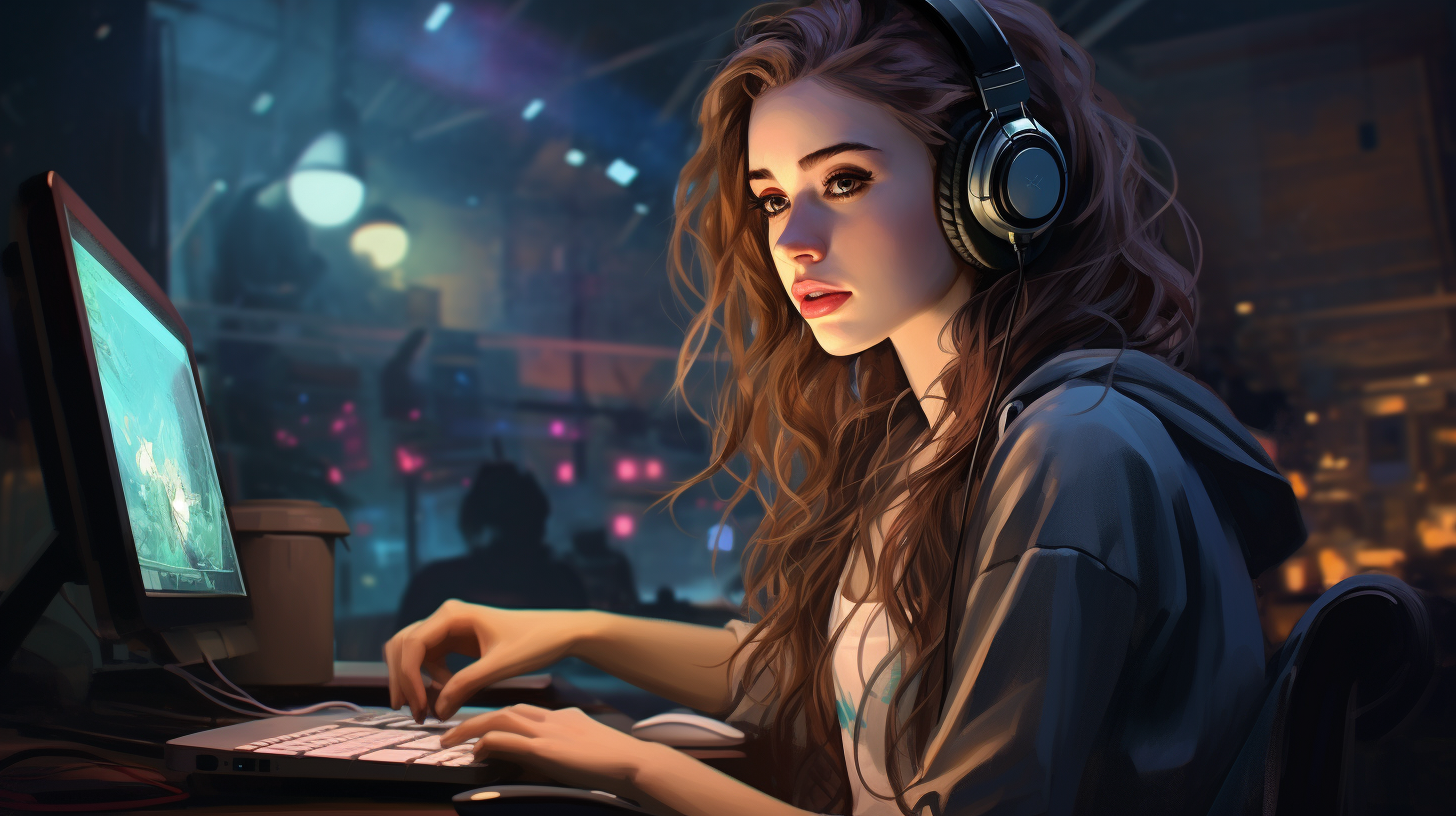
SQL for Data Formatting and Presentation
In the context of SQL, mastering functions for data transformation is akin to wielding a powerful toolset that allows you to manipulate and reshape your data to meet specific needs. These functions enable you to format, convert, and otherwise transform your data to extract meaningful insights. Let’s delve into some of the key functions that can elevate your SQL queries.
1. String Functions
String functions are essential for manipulating textual data. They allow you to modify, extract, and format strings in various ways. Here are a few commonly used string functions:
- Joins two or more strings together.
- Extracts a portion of a string.
- Convert strings to upper or lower case, respectively.
For instance, to concatenate a first name and last name into a full name, you could use:
SELECT CONCAT(first_name, ' ', last_name) AS full_name FROM users;
2. Date Functions
Date functions are invaluable when dealing with temporal data. They allow you to perform operations such as extracting parts of a date, calculating intervals, or formatting dates. Common date functions include:
- Returns the current date.
- Calculates the difference between two dates.
- Formats a date according to a specified format.
To find the number of days between two dates, you might use:
SELECT DATEDIFF(end_date, start_date) AS days_difference FROM events;
3. Numeric Functions
When dealing with numerical data, SQL provides a variety of numeric functions that allow for calculations, rounding, and mathematical operations. Some of the most used numeric functions are:
- Rounds a number to the specified number of decimal places.
- Calculates the average value of a numeric column.
- Sums up values in a numeric column.
To calculate the average salary from a salary column, you would use:
SELECT AVG(salary) AS average_salary FROM employees;
4. Conditional Functions
Conditional functions allow for logical evaluations and conditional expressions, allowing you to perform different actions based on specific conditions. The most notable among these is:
- Provides conditional logic in SQL queries.
A typical use case for a CASE statement could be assigning performance ratings based on sales figures:
SELECT employee_id, CASE WHEN sales > 100000 THEN 'Excellent' WHEN sales > 50000 THEN 'Good' ELSE 'Needs Improvement' END AS performance_rating FROM sales_data;
Using Aggregate and Window Functions for Insightful Reporting
Aggregate and window functions serve as cornerstones in SQL for generating insightful reports, transforming raw data into meaningful information that informs decision-making. These functions allow you to summarize data, perform calculations across sets of rows, and provide analytical insights without the need for complex joins or subqueries. Let’s explore each type in detail.
Aggregate Functions
Aggregate functions operate on sets of rows and return a single value, making them ideal for summarizing data. Common aggregate functions include:
- Returns the total number of rows that match a specified criterion.
- Calculates the total sum of a numeric column.
- Computes the average value of a numeric column.
- Finds the minimum value in a set.
- Returns the maximum value in a set.
For example, to determine the total number of orders placed by customers, you could execute the following query:
SELECT COUNT(*) AS total_orders FROM orders;
To find the average sales amount, you might use:
SELECT AVG(sales_amount) AS average_sales FROM sales;
Window Functions
Unlike aggregate functions, window functions perform calculations across a specified range of rows without collapsing the result set into a single output. This allows for detailed analysis while retaining individual row context. Common window functions include:
- Assigns a unique sequential integer to rows within a partition of a result set.
- Similar to ROW_NUMBER, but rows with equal values receive the same rank.
- Like RANK, but does not leave gaps between ranks.
- Can be used as a window function to compute running totals.
For instance, to assign a rank to each employee based on their sales performance, you could write:
SELECT employee_id, sales_amount, RANK() OVER (ORDER BY sales_amount DESC) AS sales_rank FROM sales_data;
To calculate a running total of sales, the query would look like this:
SELECT order_date, sales_amount, SUM(sales_amount) OVER (ORDER BY order_date) AS running_total FROM sales_orders;
Crafting Dynamic Queries for Flexible Data Presentation
Dynamic queries are a robust approach to SQL that allows for the creation of adaptable and flexible SQL statements at runtime. They are particularly useful when the exact structure of the SQL command is not known ahead of time, or when you need to incorporate variable conditions into your query. This capability is a game-changer for developers who seek to create applications that respond to user inputs or other runtime factors.
To craft dynamic queries, you typically use SQL string construction techniques to build your SQL statements. This can be achieved through the use of programming languages that interface with your SQL database, such as Python, Java, or PHP, even though SQL itself does not natively support dynamic execution in the same way.
Here’s a simplified example of how you might construct a dynamic SQL query. Suppose you want to retrieve user details based on a dynamic input parameter. The SQL string can be built based on user input:
SET @user_input = 'admin'; SET @sql_query = CONCAT('SELECT * FROM users WHERE role = ''', @user_input, ''';'); PREPARE stmt FROM @sql_query; EXECUTE stmt; DEALLOCATE PREPARE stmt;
In this example, the SQL command is constructed dynamically, allowing for different roles to be queried without rewriting the query’s structure. The use of PREPARE, EXECUTE, and DEALLOCATE commands showcases how SQL can be manipulated to perform dynamic operations safely.
One critical aspect to consider when crafting dynamic queries is the potential for SQL injection attacks. Always ensure that user input is sanitized or parameterized correctly to prevent execution of unintended commands. Using prepared statements, as shown above, is a robust method to mitigate this risk.
Moreover, for applications that need to adjust the query based on multiple conditions, you could use a series of conditional constructs. Here’s an example where the query dynamically adjusts based on whether a username filter is applied:
SET @username = 'john_doe'; SET @sql_query = 'SELECT * FROM users WHERE 1=1'; IF @username IS NOT NULL THEN SET @sql_query = CONCAT(@sql_query, ' AND username = ''', @username, ''''); END IF; PREPARE stmt FROM @sql_query; EXECUTE stmt; DEALLOCATE PREPARE stmt;
This approach allows you to keep your SQL queries clean and maintainable, even as you scale up to more complex requirements. The flexibility of dynamic queries is essential when data presentation needs to adapt based on user actions or inputs.
Best Practices for Enhancing Readability and Usability in SQL Outputs
When it comes to enhancing the readability and usability of SQL outputs, clarity is paramount. A well-structured output not only makes it easier for users to interpret the results but also facilitates better decision-making. Here are some best practices to incorporate into your SQL queries to ensure they’re both user-friendly and effective.
1. Use Descriptive Aliases
Aliases can significantly improve the understanding of your output by providing context to the data. Instead of returning columns with generic names, opt for descriptive aliases that convey the meaning of the data. For instance:
SELECT CONCAT(first_name, ' ', last_name) AS full_name, email AS user_email FROM users;
This practice helps users grasp what each column represents without requiring them to cross-reference with the underlying database schema.
2. Format Numeric Outputs
When displaying numeric data, especially financial figures, it is critical to format the output for easier comprehension. Use functions to format numbers, such as rounding or converting them to currency. Here’s an example:
SELECT ROUND(salary, 2) AS formatted_salary, FORMAT(salary, 2) AS salary_currency FROM employees;
In this case, rounding the salary to two decimal places and formatting it as currency enhances clarity, making it easier for users to interpret the financial information.
3. Order and Group Data Logically
Logical ordering of data can help users quickly find the information they need. Use the ORDER BY
clause to sort results based on relevant columns, and apply GROUP BY
to summarize data when appropriate. For example:
SELECT department_id, COUNT(*) AS employee_count FROM employees GROUP BY department_id ORDER BY employee_count DESC;
In this query, grouping employees by department and ordering the results by employee count allows for immediate insights into organizational structure.
4. Limit Result Sets
Displaying an overwhelming amount of data can obscure key insights. Use the LIMIT
clause to restrict the number of rows returned, particularly in reports or user interfaces. For example:
SELECT * FROM orders ORDER BY order_date DESC LIMIT 10;
Here, limiting results to the most recent ten orders provides users with a focused view without extraneous information.
5. Leverage Comments
Incorporating comments within your SQL code can elucidate complex queries and enhance maintainability. This practice is especially useful when future users (or even you) revisit the code. For example:
SELECT username, last_login FROM users WHERE active = 1;
Comments help clarify the intent behind various parts of your SQL statements, providing context that might not be immediately obvious.
6. Use Consistent Formatting
Adopt a consistent style for your SQL queries, including indentation, line breaks, and capitalization of SQL keywords. This uniformity makes your queries more readable and easier to understand. For instance:
SELECT id, name, created_at FROM users WHERE status = 'active' ORDER BY created_at DESC;
By maintaining a consistent formatting style, you create a visual structure that helps users quickly parse through the SQL logic.