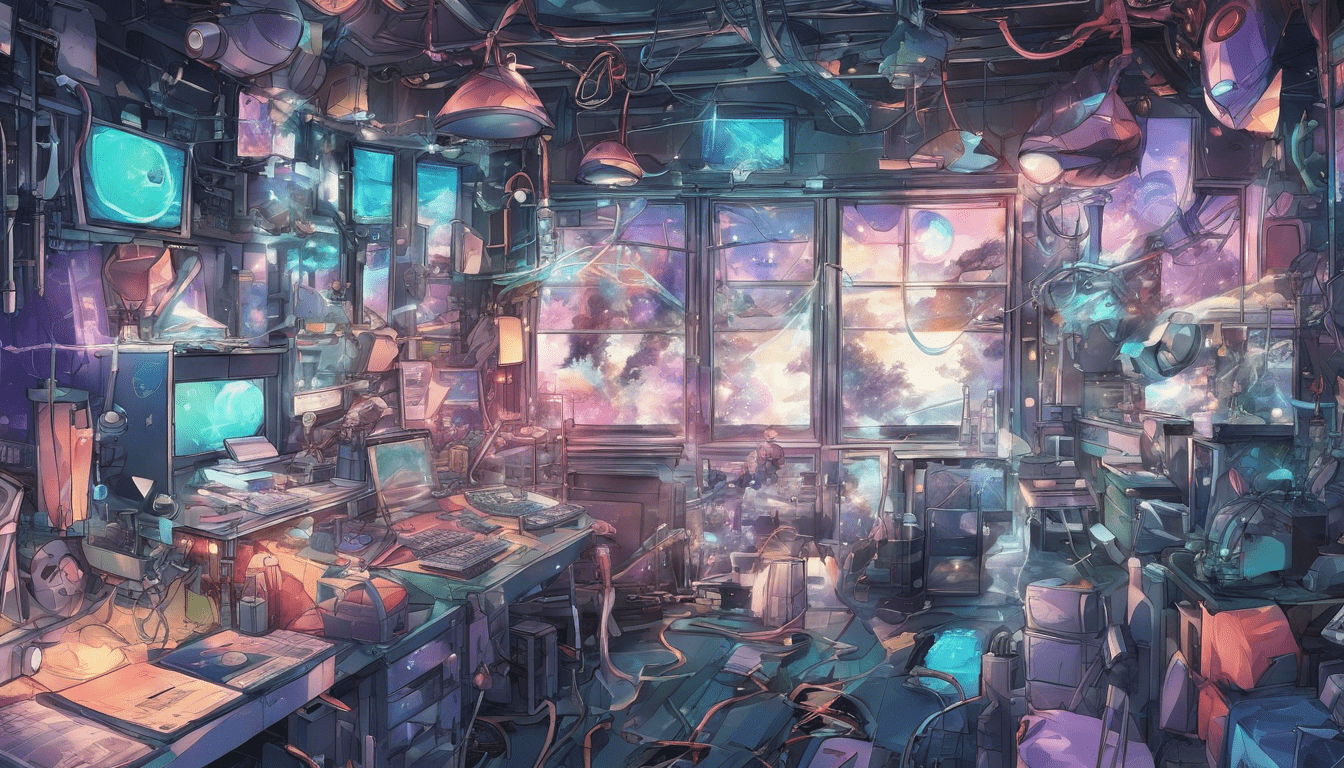
JavaScript for Fitness and Health Apps
JavaScript is a powerful programming language that can be used to create interactive and dynamic web applications including fitness and health apps. In this article, we will explore how JavaScript can be used to track various health metrics such as steps taken, calories burned, heart rate, and more.
One of the most basic ways to use JavaScript for fitness and health tracking is by accessing the device’s sensors, such as the accelerometer and gyroscope, to track physical activity. For example, to track the number of steps taken by a user, we can use the following code:
if (window.DeviceMotionEvent) { window.addEventListener('devicemotion', function(event) { var acceleration = event.acceleration; // Calculate steps taken based on acceleration data }); }
This code checks if the device has motion sensors and then adds an event listener for the ‘devicemotion’ event. When this event is triggered, we can access the acceleration data and use it to calculate the number of steps taken.
Another common feature of fitness and health apps is tracking the number of calories burned. This can be achieved by using JavaScript to calculate the user’s basal metabolic rate (BMR) and then adjust it based on their physical activity level. The following code shows an example calculation of BMR:
function calculateBMR(weight, height, age, gender) { if (gender === 'male') { return (10 * weight) + (6.25 * height) - (5 * age) + 5; } else { return (10 * weight) + (6.25 * height) - (5 * age) - 161; } }
This function takes in the user’s weight, height, age, and gender as parameters and returns their BMR based on the Harris-Benedict equation. Once we have the BMR, we can adjust it based on the user’s activity level to calculate the total calories burned.
Heart rate tracking is another important aspect of fitness and health apps. With JavaScript, we can use the Web Bluetooth API to connect to a heart rate monitor and retrieve the user’s heart rate data. Below is an example of how to do this:
navigator.bluetooth.requestDevice({ filters: [{ services: ['heart_rate'] }] }) .then(device => device.gatt.connect()) .then(server => server.getPrimaryService('heart_rate')) .then(service => service.getCharacteristic('heart_rate_measurement')) .then(characteristic => characteristic.startNotifications()) .then(characteristic => { characteristic.addEventListener('characteristicvaluechanged', function(event) { var value = event.target.value; // Parse heart rate data from value }); }) .catch(error => console.error(error));
This code requests the user’s permission to connect to a Bluetooth device that offers the ‘heart_rate’ service. Once connected, we get the primary service for heart rate and then get the characteristic for heart rate measurement. We then start notifications for that characteristic and add an event listener for when the characteristic value changes. When this event is triggered, we can parse the heart rate data from the value provided.