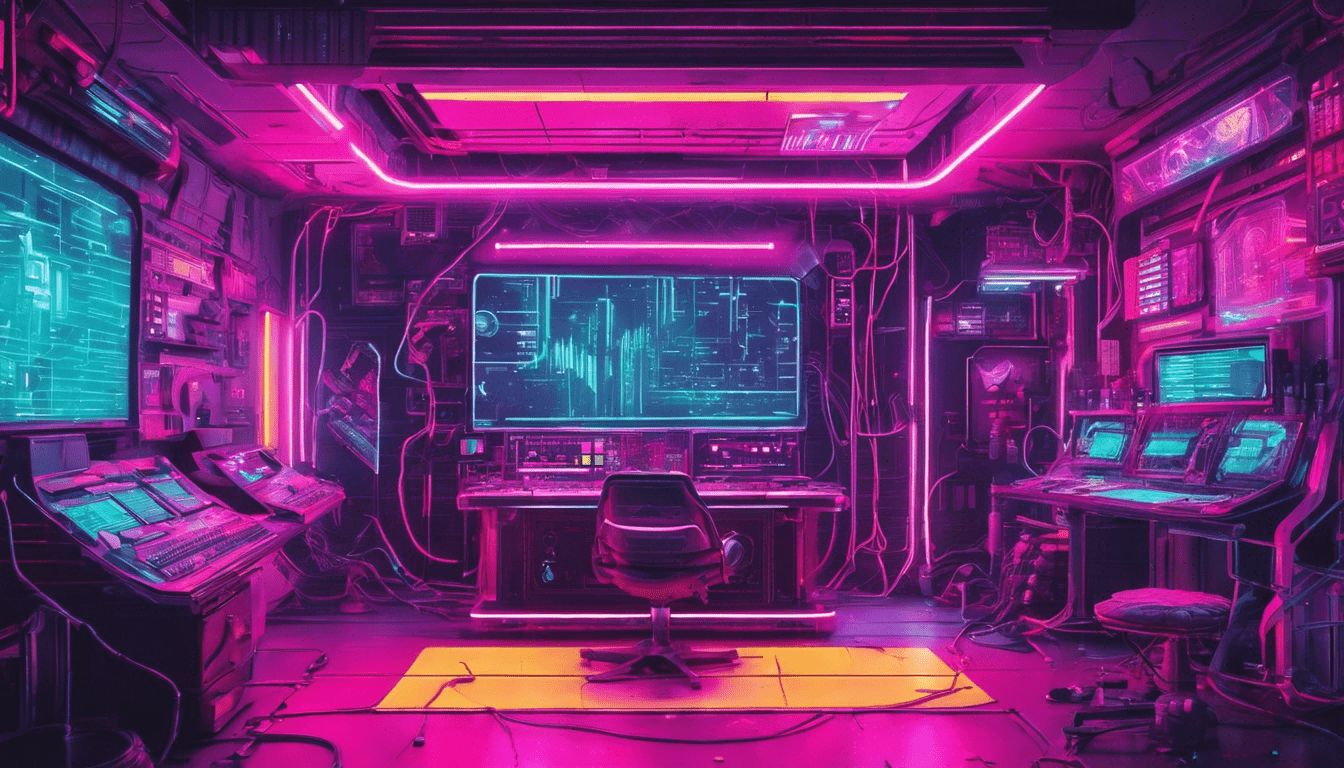
PHP Arrays: Indexed and Associative
Arrays in PHP are a fundamental data structure that allow you to store multiple values in a single variable. There are two main types of arrays in PHP: indexed and associative. Understanding the difference between these two types of arrays is important for any beginner looking to work with PHP.
Indexed Arrays
An indexed array is an array that uses numeric indexes to access its values. In other words, the values are stored in a list and each value is assigned a unique index number, starting from 0. To create an indexed array, you can use the array() function or the shorthand array syntax with square brackets.
$fruits = array("apple", "banana", "cherry"); // or $fruits = ["apple", "banana", "cherry"];
To access a value in an indexed array, you can use its index number:
echo $fruits[0]; // Outputs: apple
Indexed arrays are great for storing ordered collections of data where you want to access values using their position in the array.
Associative Arrays
An associative array is an array that uses named keys to access its values. Instead of using numeric indexes, you can use meaningful strings as keys to store and retrieve values. Associative arrays are created in a similar way to indexed arrays, but with the addition of key-value pairs.
$person = array("name" => "John", "age" => 30, "job" => "developer"); // or $person = ["name" => "John", "age" => 30, "job" => "developer"];
To access a value in an associative array, you can use its key:
echo $person["name"]; // Outputs: John
Associative arrays are useful for storing data with named keys, making it easier to read and maintain your code.
It is also possible to mix indexed and associative arrays, creating a multi-dimensional array that contains both numeric indexes and named keys. However, it’s important to keep your arrays organized to avoid confusion.
To wrap it up, understanding the difference between indexed and associative arrays is important for working with PHP. Indexed arrays are great for ordered lists, while associative arrays are perfect for named collections of data. By using these two types of arrays effectively, you can store and manage your data efficiently in your PHP applications.