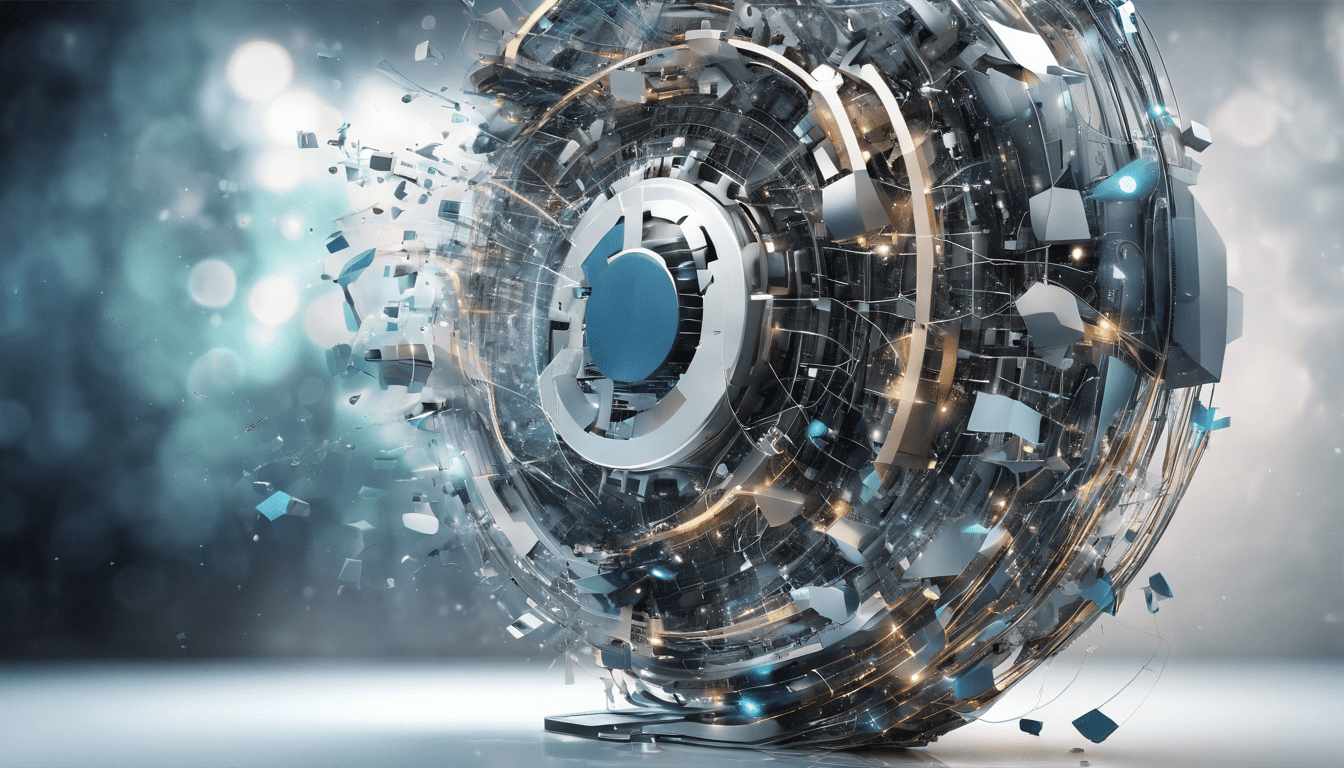
Advanced Data Filtering with SQL
Data filtering is a important aspect of working with databases. It allows us to retrieve only the information that we are interested in. SQL, or Structured Query Language, is the standard language used for managing and manipulating databases. When it comes to data filtering, SQL provides various advanced techniques that can help us refine our data retrieval. In this article, we will explore some of these advanced data filtering options with SQL.
WHERE Clause
The WHERE clause is used to filter records based on specified conditions. It can be used with SELECT, UPDATE, and DELETE statements to apply the conditions.
SELECT * FROM Customers WHERE Country = 'Germany';
The above statement retrieves all records from the Customers table where the Country is Germany.
AND, OR, and NOT Operators
The AND and OR operators are used with the WHERE clause to combine multiple conditions. The NOT operator is used to exclude records that match the condition.
SELECT * FROM Customers WHERE Country = 'Germany' AND City = 'Berlin';
The above query selects all customers from Germany who are also in Berlin.
IN Operator
The IN operator enables you to specify multiple values in a WHERE clause. It is a shorthand for multiple OR conditions.
SELECT * FROM Customers WHERE Country IN ('Germany', 'France', 'UK');
This selects all customers who are in either Germany, France, or the UK.
BETWEEN Operator
The BETWEEN operator selects values within a given range. The values can be numbers, text, or dates. It is inclusive: begin and end values are included.
SELECT * FROM Products WHERE Price BETWEEN 10 AND 20;
This selects all products with a price between 10 and 20.
LIKE Operator
The LIKE operator is used in a WHERE clause to search for a specified pattern in a column.
SELECT * FROM Customers WHERE City LIKE 's%';
The above query selects all customers whose city starts with the letter “s”. The “%” sign is used as a wildcard character to denote zero or more characters.
Wildcard Characters in LIKE Operator
- Represents zero or more characters.
- Represents a single character.
- Sets and ranges of characters to match.
SELECT * FROM Customers WHERE City LIKE '[bsp]%';
This will select all customers whose city starts with b, s, or p.
GROUP BY Statement
The GROUP BY statement is used with aggregate functions like COUNT(), MAX(), MIN(), SUM(), AVG() to group the result set by one or more columns.
SELECT COUNT(CustomerID), Country FROM Customers GROUP BY Country;
The above query counts the number of customers in each country.
HAVING Clause
The HAVING clause is used to filter groups based on some condition. It is often used with the GROUP BY clause.
SELECT COUNT(CustomerID), Country FROM Customers GROUP BY Country HAVING COUNT(CustomerID) > 5;
This will select the count of customers in each country where the count is greater than 5.
JOIN Clauses
Joins are used to combine rows from two or more tables based on a related column between them. There are different types of joins: INNER JOIN, LEFT JOIN, RIGHT JOIN, FULL OUTER JOIN.
SELECT Orders.OrderID, Customers.CustomerName FROM Orders INNER JOIN Customers ON Orders.CustomerID = Customers.CustomerID;
The above statement selects order ID and customer name from orders and customers tables where the customer ID matches in both tables.
To wrap it up, SQL provides advanced data filtering techniques that can be utilized to efficiently retrieve specific data from a database. These methods include WHERE clause, AND, OR, NOT operators, IN operator, BETWEEN operator, LIKE operator along with wildcard characters, GROUP BY statement with HAVING clause and different types of JOINs. By mastering these techniques, you can handle complex data retrieval tasks with ease.