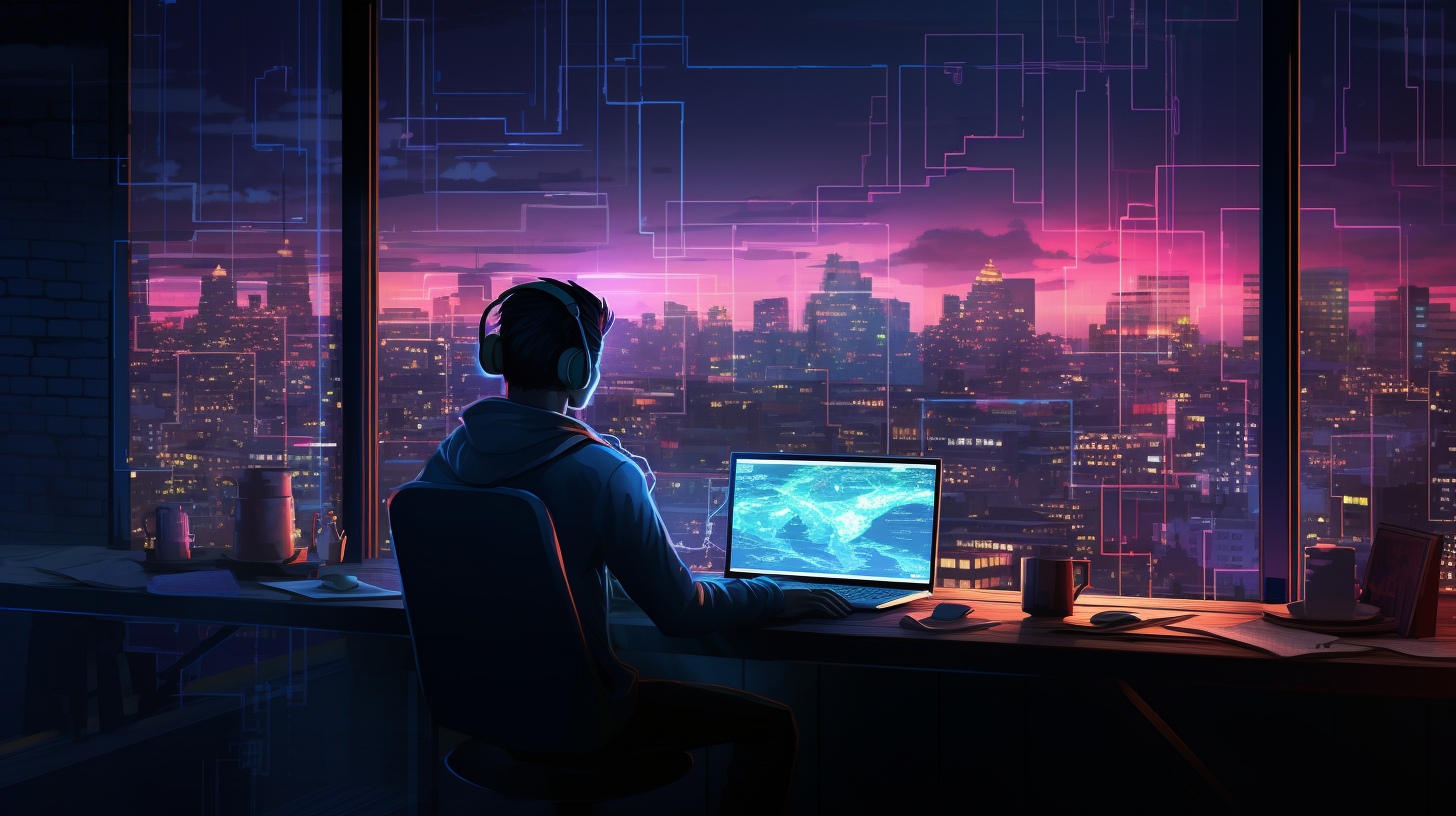
Swift Collections: Sets
Overview of Sets in Swift
In Swift, a Set is a collection type that stores unique values of the same type in an unordered list. It is similar to an array, but it does not have a defined order and cannot have duplicate values. Sets are particularly useful when you need to ensure that each element appears only once and when the order of elements is not important.
Sets in Swift are implemented as generic collections, which means they can store any type of value as long as it conforms to the Hashable protocol. This is because the set needs to be able to determine if it already contains a particular value, which it does by comparing hash values.
let numbers: Set = [1, 2, 3, 4, 5] let characters: Set = ["a", "b", "c", "d"]
One of the main advantages of using a set over an array is that looking up values is typically faster. This is because sets use hash values to quickly locate values.
let hasFive = numbers.contains(5) // true
However, since sets do not have an order, you cannot access their values using an index like you can with arrays. Instead, you have to either iterate over the set or use methods like contains
to check for the presence of a value.
Sets also offer powerful operations to combine, intersect, or subtract collections, which can be very useful for handling groups of values without needing to write complex loops or conditions.
Sets are an efficient way to manage collections of unique items in Swift. They provide fast lookup, prevent duplicates, and offer a suite of operations that can simplify your code when dealing with groups of values.
Creating Sets
To create a Set in Swift, you can use the Set initializer or simply declare a variable with the Set type and assign it an array literal containing the initial values. It’s important to note that the elements in the Set must conform to the Hashable protocol, which is automatically taken care of for basic data types like integers, strings, and booleans.
// Creating a Set with an array literal var primeNumbers: Set = [2, 3, 5, 7, 11] // Creating an empty Set var emptySet = Set()
When you initialize a Set with an array literal, Swift can infer the type of Set based on the values you provide. However, if you’re creating an empty Set, you must explicitly declare the type of elements it will contain, as shown in the second example.
It is also possible to create a Set from an array or another sequence, ensuring that only unique elements are kept. If there are duplicate values in the array, they will be discarded when the Set is created.
let arrayOfNumbers = [1, 2, 2, 3, 4, 4, 5] let uniqueNumbers = Set(arrayOfNumbers) // [1, 2, 3, 4, 5]
Remember that the order of elements in a Set is not guaranteed. When you create a Set or iterate over its elements, the order in which the elements appear can be different from the order in the original array or from one iteration to the next.
Once you’ve created a Set, you can start adding, removing, or checking for the presence of items, which will be covered in the next sections of this article.
Modifying Sets
Sets in Swift are mutable, which means you can add or remove items after the set is created. Modifying a set is a common task, and Swift provides several methods to do that efficiently.
To add a new element to a set, you use the insert
method. This method adds an element to the set if it is not already present.
var fruits: Set = ["Apple", "Banana"] fruits.insert("Cherry") // fruits now contains "Apple", "Banana", and "Cherry"
If you want to remove an element, you can use the remove
method. This method removes an element if it’s present in the set and returns the removed element, or it returns nil
if the element was not found.
if let removedFruit = fruits.remove("Banana") { print("(removedFruit) was removed from the set") } // Prints "Banana was removed from the set"
For situations where you want to remove all the elements from a set, you can use the removeAll
method.
fruits.removeAll() // fruits is now an empty set
Another useful method is update
, which updates a value in the set. If the value is found, it’s replaced with the new value. If it’s not found, the new value is added to the set.
fruits.insert("Apple") fruits.update(with: "Apricot") // fruits now contains "Apple" and "Apricot"
Lastly, you can check if a set contains a particular element using the contains
method, which returns a Boolean value.
let hasApple = fruits.contains("Apple") // true
Modifying sets in Swift is straightforward and efficient. By using these methods, you can manage the contents of a set effectively in your code.
Operations on Sets
Swift provides a set of powerful operations for performing common set-related tasks, such as union, intersection, subtraction, and symmetric difference. These operations are particularly useful when you want to combine or compare sets in various ways.
Union is used to create a new set with all the unique elements from two sets. The union
method takes another set as its parameter and returns a new set containing elements from both sets.
let evens: Set = [2, 4, 6, 8] let odds: Set = [1, 3, 5, 7] let allNumbers = evens.union(odds) // allNumbers contains [1, 2, 3, 4, 5, 6, 7, 8]
Intersection creates a new set with elements that are common to both sets. The intersection
method can be used to find shared values between sets.
let multiplesOfTwo: Set = [2, 4, 6, 8, 10] let multiplesOfThree: Set = [3, 6, 9, 12] let commonMultiples = multiplesOfTwo.intersection(multiplesOfThree) // commonMultiples contains [6]
Subtraction is used to create a new set with elements from one set that are not present in another set. The subtracting
method removes all elements of the specified set from the calling set.
let primeNumbers: Set = [2, 3, 5, 7, 11] let nonPrimes = allNumbers.subtracting(primeNumbers) // nonPrimes contains [1, 4, 6, 8]
Symmetric Difference creates a new set with elements that are in either set, but not in both. The symmetricDifference
method is useful for finding elements that are unique to each set.
let naturalNumbers: Set = [1, 2, 3, 4, 5] let fibonacciNumbers: Set = [1, 1, 2, 3, 5, 8] let uniqueNumbers = naturalNumbers.symmetricDifference(fibonacciNumbers) // uniqueNumbers contains [4, 8]
These operations can also be performed in place, modifying the original set, by using their respective mutating forms: formUnion
, formIntersection
, subtract
, and formSymmetricDifference
.
Besides these set algebra operations, you can also check relationships between sets, such as whether one set is a subset, superset, or is disjoint with another set.
- ) method.
- ) method checks for this relationship.
- ) method.
let setA: Set = [1, 2, 3] let setB: Set = [3, 4, 5] let setC: Set = [4, 5, 6] setA.isSubset(of: allNumbers) // true setC.isSuperset(of: setB) // false setA.isDisjoint(with: setC) // true
By using these operations and methods, you can handle complex set manipulations with ease, making your Swift code cleaner and more concise.
Conclusion and Further Resources
In conclusion, sets in Swift are a powerful tool for managing unique collections of items. They offer efficient lookup, prevent duplicates, and provide a suite of operations that can simplify code when dealing with groups of values. Whether you need to combine sets, find common elements, or check for relationships between sets, Swift’s set operations have you covered.
For further resources on Swift collections and sets, ponder the following:
- https://developer.apple.com/documentation/swift/set
- Apple’s Swift Programming Language Guide, which includes a section on Collection Types
- Online Swift playgrounds and coding challenges that allow you to practice set operations and other collection manipulations
- Swift forums and community discussions on best practices for using sets in different scenarios
As you continue to work with sets, remember to consider their unique characteristics and choose the right collection type for your needs. Here’s a quick code example to illustrate a practical use of sets:
// A practical example: Finding unique tags from a list of articles let articleTags = [ "Swift", "iOS", "Swift", "UI", "Design", "SwiftUI", "iOS", "Performance", "Swift" ] let uniqueTags = Set(articleTags) print(uniqueTags) // Output: ["Swift", "iOS", "UI", "Design", "SwiftUI", "Performance"]
By using the power of sets, you can simplify many common tasks in Swift programming. Don’t hesitate to dive deeper into the language’s documentation and community resources to further enhance your understanding and skills with Swift collections.