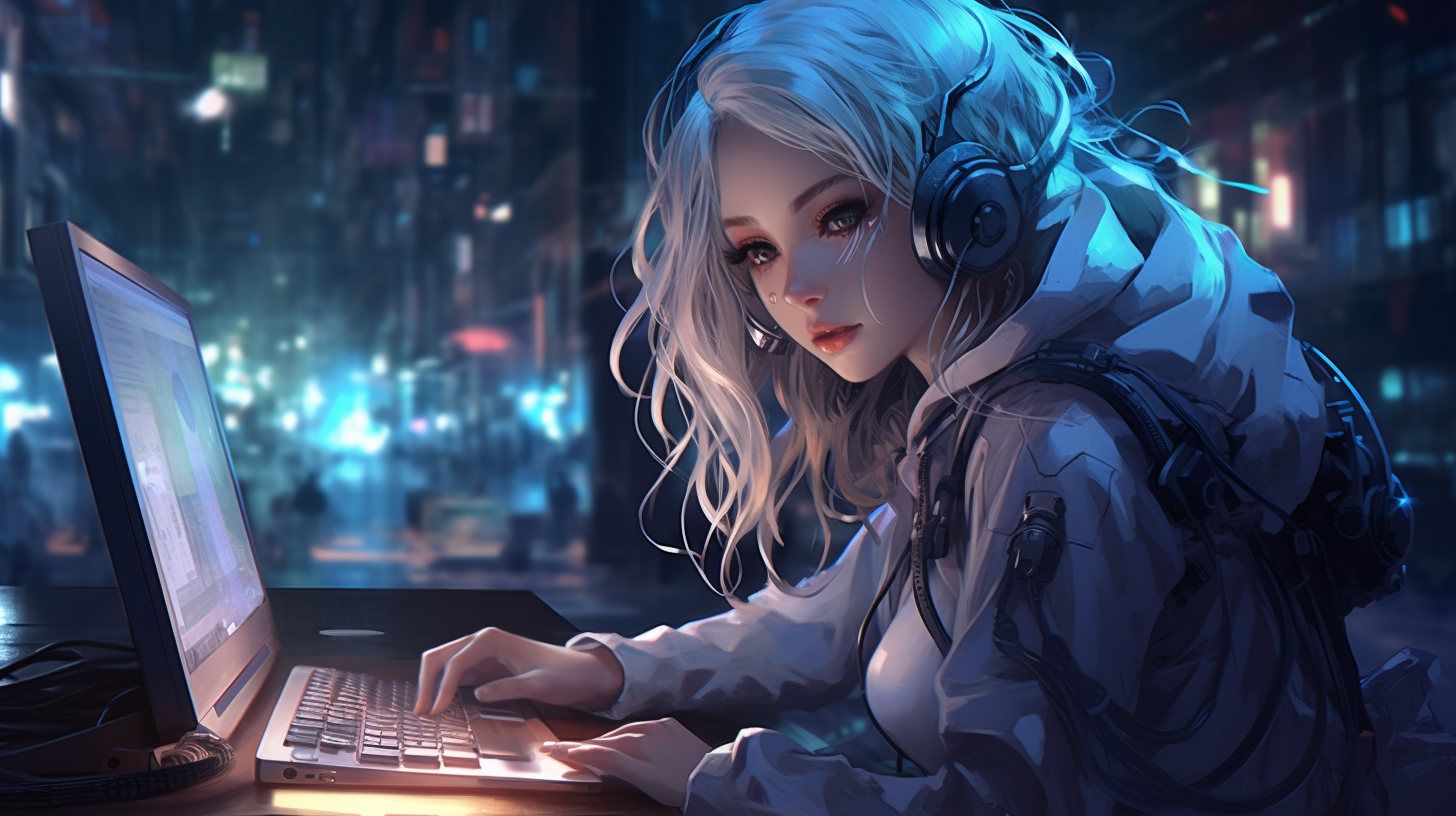
Advanced Swift: SwiftUI Basics
SwiftUI is a new framework introduced by Apple that allows developers to build user interfaces in a declarative way using Swift. With SwiftUI, you can easily create complex layouts with less code, and the framework automatically handles platform-specific features and animations. In this article, we’ll cover the basics of SwiftUI and show you how to get started with building your first SwiftUI app.
Creating a New SwiftUI Project
To get started with SwiftUI, you’ll need Xcode 11 or later. When creating a new project, make sure to select the ‘Use SwiftUI’ checkbox in the project options.
import SwiftUI @main struct MyApp: App { var body: some Scene { WindowGroup { ContentView() } } }
Understanding the View Protocol
In SwiftUI, everything is a view. A view is a piece of UI that can be displayed on the screen. To create a custom view, you’ll need to conform to the View protocol and implement the required body property.
struct ContentView: View { var body: some View { Text("Hello, World!") } }
Building UI with Stacks
SwiftUI provides three kinds of stacks: VStack, HStack, and ZStack. VStack arranges views vertically, HStack arranges them horizontally, and ZStack layers views on top of each other.
VStack { Text("First") Text("Second") Text("Third") }
Adding Modifiers
Modifiers allow you to customize the appearance and behavior of views. You can add modifiers by chaining them to a view.
Text("Hello, World!") .font(.title) .foregroundColor(.blue)
Using State and Binding
State is a property wrapper that allows you to create mutable state within a view. Binding is used to pass state between views.
@State private var isOn = false Toggle(isOn: $isOn) { Text("Toggle Switch") }
Previewing Your UI
Xcode provides a powerful preview feature that allows you to see your UI changes in real-time as you code. To preview your SwiftUI view, click on the Resume button in the canvas.
struct ContentView_Previews: PreviewProvider { static var previews: some View { ContentView() } }