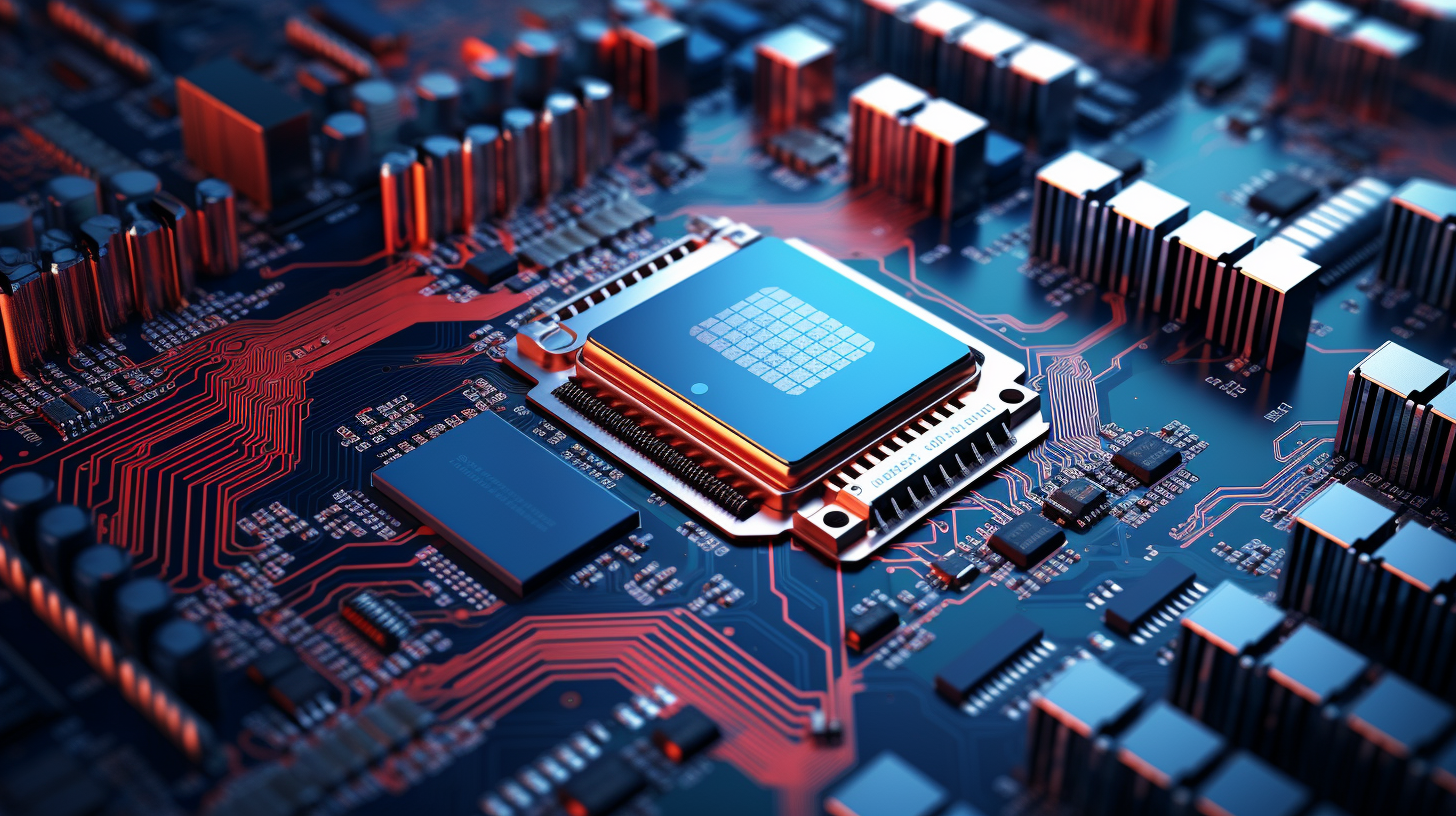
Creating Interactive Bash Scripts
Bash scripts are a powerful tool for automating tasks in Unix-like operating systems. By creating interactive bash scripts, you can make complex workflows more simple to operate, by prompting users for input and making decisions based on their responses. In this article, we’ll explore how to create interactive bash scripts using the read command and the dialog utility.
The read command is built into bash and enables you to capture user input from the command line. Here’s a simple example of using read to ask the user for their name:
echo "What's your name?" read name echo "Hello, $name!"
In this script, the user is greeted and then prompted to type their name. The script then inserts the name into a greeting message. You can prompt for multiple inputs like this:
read -p "Enter your first and last name: " first last echo "Hello, $first $last!"
The -p
option allows you to provide a prompt inline, rather than using echo
.
If you want to make your script more robust, you can also handle cases where the user doesn’t provide input:
echo "What's your name?" read name if [ -z "$name" ]; then echo "You must enter your name." exit 1 fi echo "Hello, $name!"
The -z
test checks if the variable name
is empty, and if it’s, prints a message and exits the script with a non-zero exit code indicating an error.
For more complex user interfaces, you can use the dialog utility which provides graphical dialogs. To use dialog, you’ll first need to install it using your system’s package manager (e.g., apt-get install dialog
on Debian/Ubuntu).
Here’s an example of using dialog with its –inputbox option to prompt the user for their name in a text box:
name=$(dialog --stdout --inputbox "Enter your name" 0 0) echo "Hello, $name!"
This will open a graphical dialog box where the user can enter their name. The result is captured in the name
variable.
You can also create more advanced interfaces like menus. Here’s an example of a simple menu that lets the user choose an action:
exec 3>&1 selection=$(dialog --backtitle "Menu" --title "Menu Title" --clear --cancel-label "Exit" --menu "Please select:" 15 50 4 "1" "Option 1" "2" "Option 2" "3" "Option 3" 2>&1 1>&3) exit_code=$? exec 3>&- case $exit_code in 0) echo "You selected $selection" ;; 1) echo "Exiting" ;; *) echo "An unexpected error occurred" ;; esac
This script will display a menu with three options. When the user makes a selection, the script will print which option was selected. If the user chooses ‘Exit’, the script will print ‘Exiting’ instead.
To wrap it up, Bash scripts can be made interactive by using simple commands like read, as well as more complex tools like dialog. By asking for user input, scripts can be more dynamic and useful in a variety of scenarios.