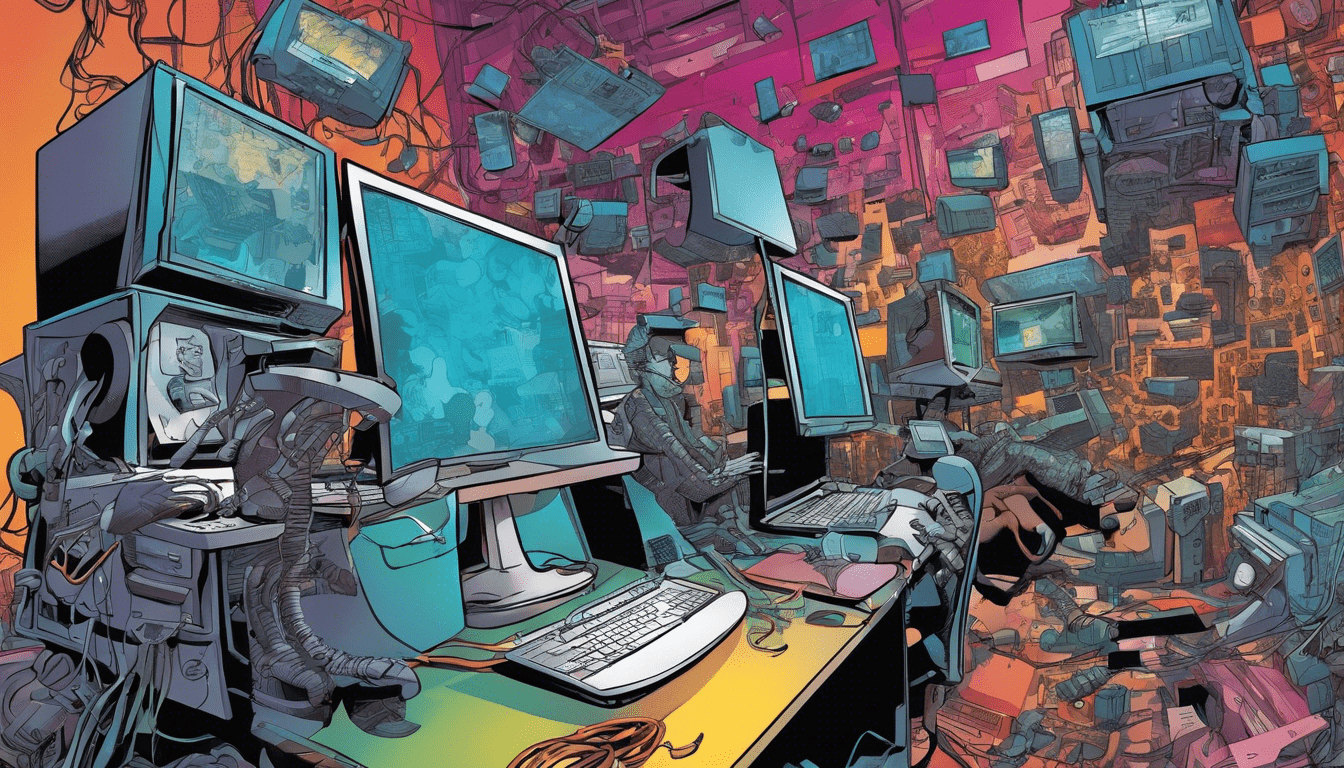
File Handling in Python: Read and Write Files
File handling is an essential aspect of programming, especially in Python. It enables you to interact with files stored on your computer’s hard disk or any other external storage devices. With file handling, you can read the contents of a file, write new information to an existing file, or even create a new file.
In this article, we will explore the process of file handling in Python, focusing on reading and writing files. We will cover the basic concepts and provide clear code examples to help beginners understand the topic.
Reading Files
To read a file in Python, you need to open it first. The `open()` function is used to open a file, and it takes two arguments – the name of the file and the mode in which it should be opened. The mode determines whether you want to read, write, or append to the file.
For reading a file, you need to specify the mode as `’r’`. Here’s an example that demonstrates how to read the contents of a file named `example.txt`:
file = open('example.txt', 'r') content = file.read() print(content) file.close()
Let’s break down the code:
– We use the `open()` function to open the file named `example.txt` in read mode (`’r’`).
– The `read()` method is used to read the contents of the file and store them in the `content` variable.
– Finally, we print out the content and close the file using the `close()` method.
It’s important to close the file after you have finished working with it to free up system resources. You can do this by calling the `close()` method on the file object.
Writing Files
To write to a file in Python, you also need to open it first. However, this time you need to specify the mode as `’w’` to indicate that you want to write to the file. If the file doesn’t exist, it will be created automatically.
Here is an example that demonstrates how to write information to a file:
file = open('example.txt', 'w') file.write('Hello, World!') file.close()
In this example, we open the `example.txt` file in write mode using the `open()` function. Then, we use the `write()` method to write the text `’Hello, World!’` to the file. Finally, we close the file.
If the file already exists and you open it in write mode, its previous contents will be overwritten. If you want to add new content to an existing file instead, you can use append mode (`’a’`) by specifying it as the second argument in the `open()` function.
Closing Files automatically with ‘with’
In Python, it is recommended to use a `with` statement when working with files. This ensures that the file is automatically closed once you are done with it, even if an exception occurs.
Here’s how the previous examples look using the `with` statement:
with open('example.txt', 'r') as file: content = file.read() print(content) with open('example.txt', 'w') as file: file.write('Hello, World!')
By using the `with` statement, you don’t have to explicitly call the `close()` method. The file will automatically be closed at the end of the `with` block.
Handling File Exceptions
When working with files, it’s common to encounter potential errors such as a file not found or permission denied. To handle these exceptions gracefully, you can wrap your file operations within a try-except block.
try: with open('example.txt', 'r') as file: content = file.read() print(content) except FileNotFoundError: print("File not found.") except PermissionError: print("Permission denied.")
In this example, we are attempting to read the contents of `example.txt`. If the file is not found, a `FileNotFoundError` exception will be raised. Similarly, if we don’t have the necessary permissions to access the file, a `PermissionError` exception will be raised.
By catching these exceptions, we can handle them appropriately and display an error message to the user.
File handling is a important aspect of programming, and Python provides convenient methods for reading and writing files. We explored how to open files, read their content, write new information, and close the files properly.
Remember to always close files after you have finished working with them, or better yet, use the `with` statement to ensure automatic closure. Additionally, handle potential exceptions that may occur when working with files to ensure your program behaves gracefully.
By mastering file handling in Python, you will have the necessary skills to interact with files effectively and build more advanced applications.
Great article on file handling in Python! One important piece of information that I would add is the concept of file paths. In the examples provided, the file ‘example.txt’ is assumed to be in the same directory as the Python script. However, it is important to note that files can be located in different directories or even on external storage devices.
To specify the path of a file, you can provide the absolute path (e.g., ‘C:/path/to/example.txt’) or the relative path (e.g., ‘../folder/example.txt’). The relative path is relative to the current working directory of the Python script.
Including file paths will help readers understand how to access files from different locations and make their file handling more flexible.
Overall, a well-written article that covers the basics of file handling in Python and provides clear examples. Keep up the good work!