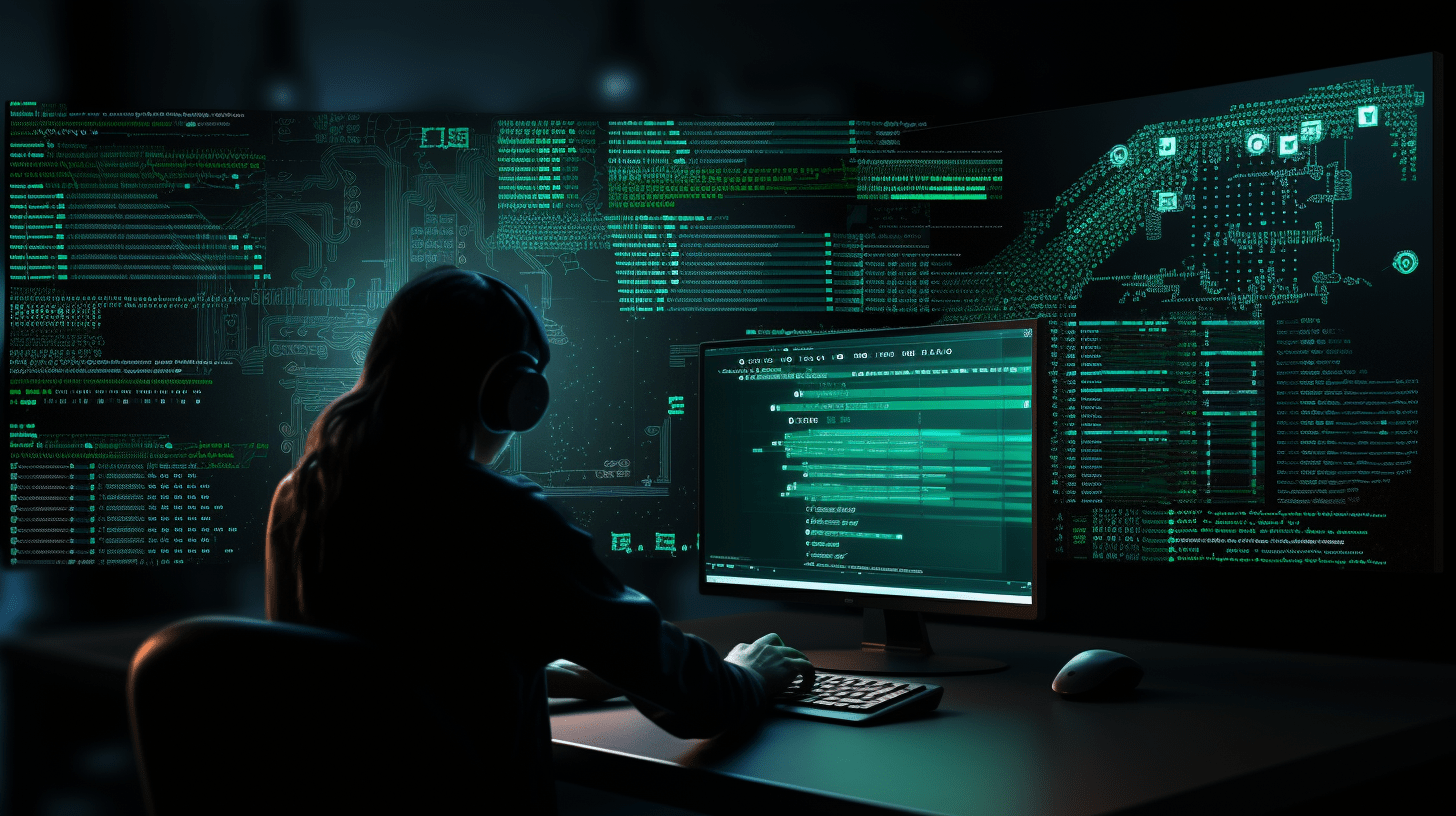
PHP Performance Optimization
When it comes to web development, the performance of your website is important. The speed and efficiency of how your PHP code runs can make a significant difference in user experience and search engine rankings. Therefore, optimizing your PHP code for better performance is essential. Here are some tips on how to improve the performance of your PHP scripts.
Use Updated PHP Version
One of the easiest ways to improve PHP performance is to use the latest version of PHP. Newer versions of PHP come with performance improvements and optimizations that can make your code run faster.
Using Single Quotes Over Double Quotes for Performance Boost
In PHP programming, a subtle but impactful optimization technique involves preferring single quotes (”) over double quotes (“”) for string declarations. This approach significantly enhances the performance of PHP scripts, especially when dealing with real-time interpreters as opposed to compilers.
The key difference between single and double quotes in PHP lies in the way the interpreter processes the string. When a string is enclosed in double quotes, the PHP interpreter scans through it to identify and parse any variables or special characters. This parsing requires additional processing time and resources, particularly noticeable in loops or large-scale string operations.
In contrast, strings enclosed in single quotes are taken as literal values. The interpreter does not attempt to parse or evaluate any content within single quotes. This means that the processing time is reduced, as the interpreter simply treats the content as plain text without looking for variables or special characters.
This distinction is important for real-time interpreters, where every millisecond of processing time counts. By avoiding unnecessary parsing, you can ensure that your PHP code runs more efficiently, using less CPU time and potentially reducing server load.
// Using double quotes // The interpreter checks for variables or special characters $doubleQuotedString = "This is a string with a variable: $value"; // Using single quotes // The interpreter treats this as a literal string, no parsing for variables $singleQuotedString = 'This is a string without variable parsing'; // When needing to include variables, concatenate with single quotes $name = 'John'; $concatenatedString = 'Hello, ' . $name . '. Welcome back!'; // In a loop, using single quotes can improve performance for ($i = 0; $i < 1000; $i++) { $str = 'Iteration number ' . $i; // Faster processing with single quotes }
Optimize Database Queries
Database queries can be a bottleneck in PHP applications. To improve performance, make sure to index your database and write optimized SQL queries. Avoid using SELECT *
when you only need specific columns, and limit the number of rows returned by using LIMIT
.
$query = 'SELECT id, name FROM users LIMIT 10';
Also don’t forget to optimize database interaction by reducing the number of queries. Use JOINs instead of multiple queries, cache results when possible, and avoid querying within loops.
Use Caching
Caching can significantly improve the performance of your PHP application. By storing frequently accessed data in cache, you can reduce the number of database queries and speed up page loading times.
$cacheKey = 'user_list'; if (apc_exists($cacheKey)) { $users = apc_fetch($cacheKey); } else { $users = getUsersFromDatabase(); apc_store($cacheKey, $users); }
Minimize Use of Loops
Loops can be resource-intensive, especially if they’re nested or iterate over large datasets. Always look for ways to minimize the use of loops in your code.
Avoid Unnecessary Calculations
Performing calculations or calling functions inside loops can slow down your code. Perform any necessary calculations outside of loops whenever possible.
$total = calculateTotal($items); foreach ($items as $item) { // do something with $total and $item }
Use Opcode Caching
Opcode caching is a technique that stores precompiled script bytecode in memory, which can speed up PHP execution. Popular opcode caches include APCu and OPcache.
// Opcode caching is enabled at the server level. // To enable OPcache in PHP.ini: // opcache.enable=1
Use Static Methods and Variables
Static methods and properties in a class do not require an instance to be accessed. They’re stored in memory only once during a script’s execution, making them faster than repeatedly instantiating a class. Use static methods and variables when you don’t need an object’s state.
class Util { public static $count = 0; public static function increment() { self::$count++; } } Util::increment(); echo Util::$count; // Outputs: 1
Prefer Foreach Over Other Looping Constructs
In PHP, foreach
loops are generally faster and more optimized compared to for
or while
loops, especially when iterating over arrays. They provide cleaner code and better performance.
$array = [1, 2, 3, 4, 5]; // Using foreach foreach ($array as $value) { echo $value . ' '; }
Avoid Excessive Use of Regular Expressions
Regular expressions are powerful but computationally expensive. Use string functions like strpos()
, str_replace()
, or substr()
whenever possible, as they’re faster and consume less memory.
$string = 'Hello World'; // Instead of using regex if (preg_match('/World/', $string)) { // do something } // Use strpos if (strpos($string, 'World') !== false) { // do something }
Use In-Built PHP Functions
PHP has a vast array of built-in functions optimized for performance. Often, these functions perform tasks more efficiently than custom code written for the same purpose.
// Instead of writing a custom function to reverse a string $reversed = strrev('Hello World'); // Outputs: dlroW olleH
Use Proper Data Structures
Choosing the right data structure for a task can significantly impact performance. For example, if you frequently need to look up values, an associative array might be more efficient than a numerically indexed array.
// Using an associative array for faster lookups $users = [ 'john' => ['age' => 25, 'email' => '[email protected]'], 'jane' => ['age' => 28, 'email' => '[email protected]'] ]; echo $users['john']['email']; // Faster lookup
Avoid Using @ Error Suppression Operator
The @ operator suppresses error messages, which can make debugging harder and incurs performance overhead. Handle errors gracefully through proper exception handling.
$value = 'Hello World'; // Instead of suppressing errors $result = @$someArray['key']; // Handle errors properly if (isset($someArray['key'])) { $result = $someArray['key']; } else { // handle the error }
Profile Your Code
Profiling tools such as Xdebug can help you identify bottlenecks in your code. By analyzing your code’s performance, you can focus your optimization efforts where they’re most needed.
To wrap it up, optimizing PHP performance involves using the latest PHP version, optimizing database queries, caching frequently accessed data, minimizing the use of loops, avoiding unnecessary calculations, using opcode caching, and profiling your code. By implementing these optimizations, you can improve the speed and efficiency of your PHP applications.
I found this article to be informative and interesting. It highlighted some of the popular essential oils like lavender, peppermint, and tea tree oil and explained their therapeutic properties. However, I believe the article could have also highlight the safety precautions and potential side effects of using essential oils.
It is important to remember that essential oils are highly concentrated substances and should be used with caution. Some essential oils can cause skin irritation or allergic reactions, especially when applied directly to the skin. It would have been helpful if the article included information on proper dilution ratios and recommended carrier oils for safe usage.
Additionally, it would have been beneficial if the writer discussed the sourcing and quality of essential oils. Not all essential oils on the market are created equal, and it is important to choose high-quality oils from reputable sources.