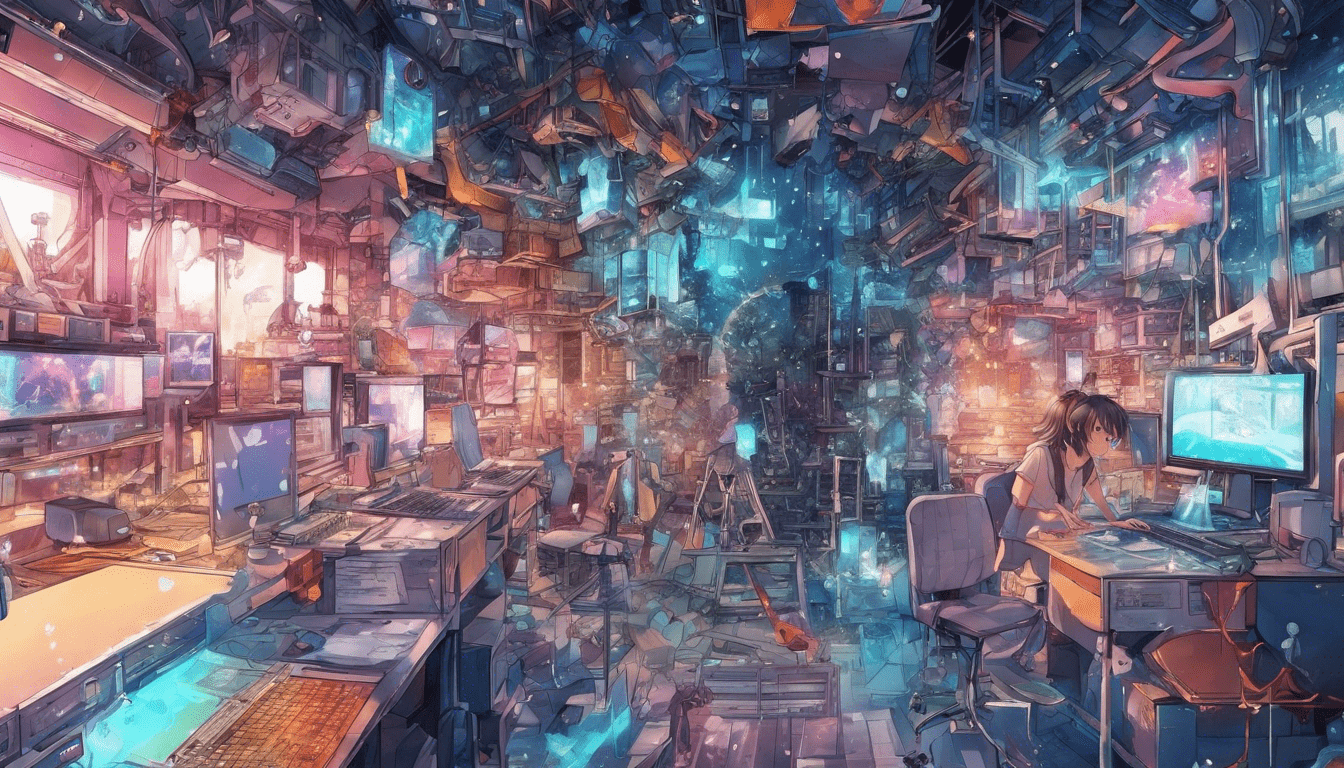
Working with Web APIs in JavaScript
Working with Web APIs in JavaScript is an essential skill for modern web development. An API, or Application Programming Interface, is a set of rules and protocols that allows different software programs to communicate with each other. Web APIs, in particular, allow web applications to interact with external services and resources over the HTTP protocol.
One of the most common tasks when working with Web APIs in JavaScript is making HTTP requests to retrieve or send data. This can be done using the fetch function, which is built into state-of-the-art browsers and provides a simple and powerful way to make HTTP requests.
The fetch function takes two arguments: the URL of the API endpoint you want to access, and an optional object that contains any additional options like headers or the request method (e.g. GET, POST, PUT, DELETE). The function returns a promise that resolves with a Response object, which contains the data returned by the API.
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
In the above example, we are making a GET request to the https://api.example.com/data
endpoint. We then use the .json()
method on the response object to parse the JSON data returned by the API. Finally, we log the data to the console or handle any errors that may occur during the request.
You can also send data to an API using the fetch function. To do this, you need to set the method and body properties in the options object. Here’s an example of how to send a POST request with JSON data:
fetch('https://api.example.com/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ key: 'value' }) }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
In this example, we are setting the request method to POST and specifying the content type as JSON in the headers. We then use JSON.stringify()
to convert our data into a JSON string before sending it in the body of the request.
It is important to note that some APIs may require authentication in order to access their data or perform certain actions. This can be done by including an authentication token or other credentials in the request headers. Be sure to read the API documentation carefully to understand how to properly authenticate your requests.
In conclusion, working with Web APIs in JavaScript is a powerful way to interact with external data and services. By using the fetch function, you can easily make HTTP requests, handle responses, and send data to APIs. With practice and patience, you’ll be able to integrate all sorts of exciting features into your web applications using APIs.