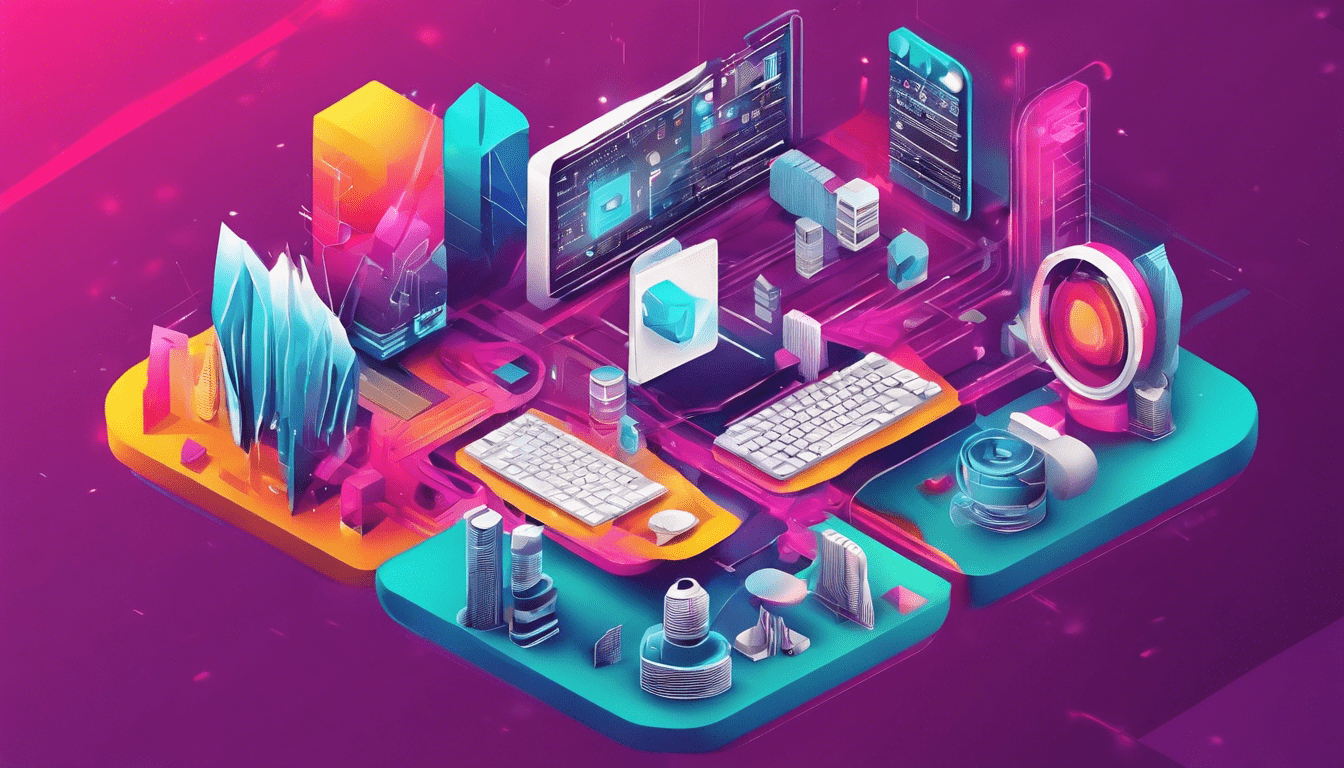
Getting Started with Java
Java is a popular programming language that’s used across various platforms and industries. It’s known for its versatility, ease of use, and robustness. If you are new to programming or looking to learn Java, this article will guide you through the basics of getting started with Java.
Before you start writing Java code, you need to set up your development environment. You will need to download and install the Java Development Kit (JDK) from the official Oracle website. The JDK includes the tools you need to compile and run Java programs, such as the Java compiler and the Java Virtual Machine (JVM).
Once you have installed the JDK, you can write your first Java program using a simple text editor like Notepad or an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA. IDEs provide additional features like code completion, debugging, and project management to make your development process easier.
A Java program consists of one or more classes, each containing methods and variables. The entry point of a Java program is the main
method, which is always defined within a class with the following signature:
public static void main(String[] args) { // Your code goes here }
Let’s write a simple Java program that prints “Hello, World!” to the console. Create a new file called HelloWorld.java
and add the following code:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
To compile the program, open the command prompt or terminal, navigate to the directory where you saved the file, and run the following command:
javac HelloWorld.java
This will generate a HelloWorld.class
file, which contains the bytecode that can be executed by the JVM. To run the program, use the following command:
java HelloWorld
You should see “Hello, World!” printed to the console.
Java is an object-oriented programming language, which means that it revolves around the idea of objects and classes. A class is a blueprint for creating objects, which are instances of the class. Classes contain fields (variables) and methods (functions) that define the properties and behavior of objects.
- Variables declared within a class that hold data.
- Functions defined within a class that perform actions.
- A special method this is called when an object is created.
Let’s create a simple class called Person
with fields for name and age, and a method to say hello:
public class Person { String name; int age; public Person(String name, int age) { this.name = name; this.age = age; } public void sayHello() { System.out.println("Hello, my name is " + name + " and I am " + age + " years old."); } }
To use the Person
class, create an instance of it and call the sayHello
method:
public class Main { public static void main(String[] args) { Person person = new Person("John", 30); person.sayHello(); } }
This will print “Hello, my name is John and I am 30 years old.” to the console.
Java has several built-in data types for variables, including:
- Integer numbers.
- Floating-point numbers.
- True or false values.
- Single characters.
- Sequences of characters (text).
Variables must be declared with their data type before they can be used, for example:
int number = 10; double pi = 3.14; boolean isTrue = true; char letter = 'A'; String text = "Hello";
Java also has control flow statements, such as if
, else
, for
, and while
, which allow you to make decisions and repeat actions in your code.
To wrap it up, starting with Java involves setting up your development environment, understanding classes and objects, writing Java code using variables and control flow statements, and running your programs using the command line or an IDE. Keep practicing and exploring more advanced topics as you become comfortable with the basics.