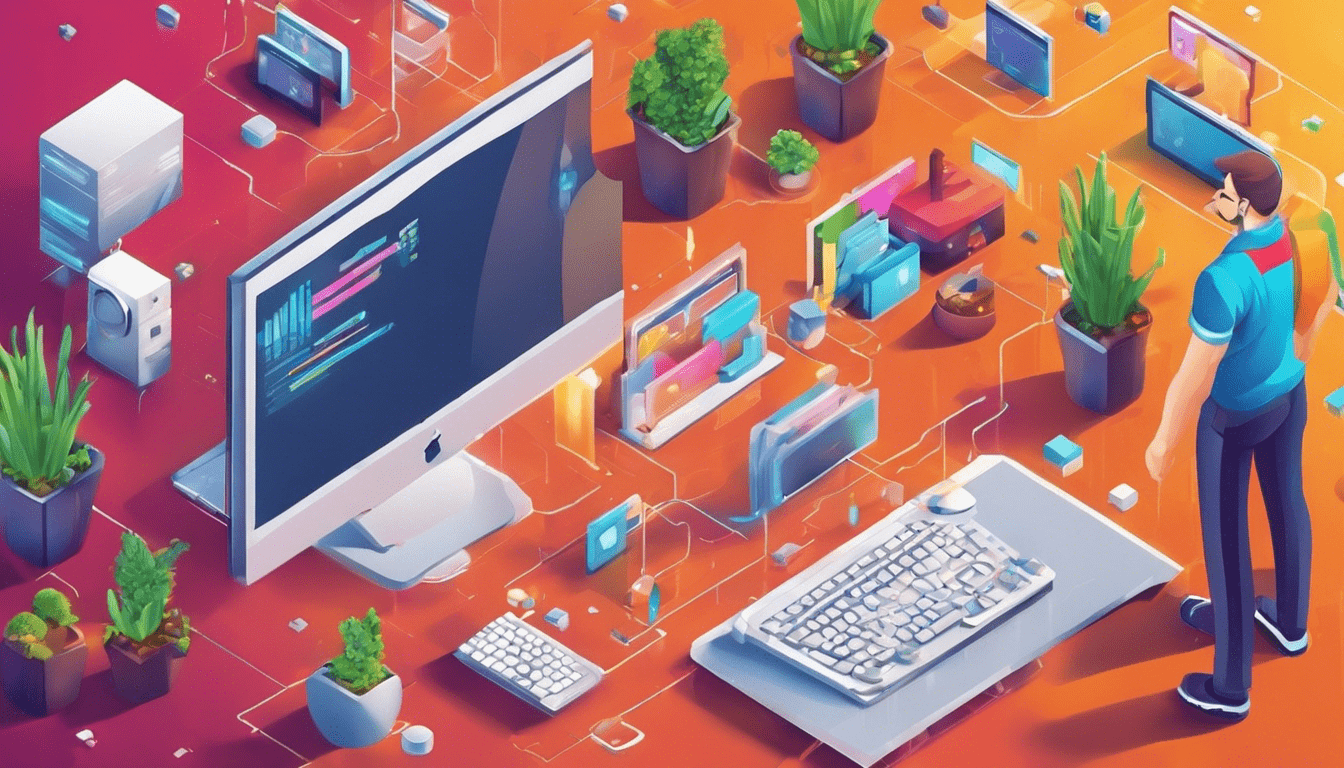
Java and NoSQL Databases: An Introduction
In the ever-evolving technology world, data forms the backbone of all operations. We encounter enormous volumes of data in different formats, from various sources, in a variety of structures. To handle such complex and heterogeneous data efficiently, tech experts introduced NoSQL databases, a flexible and scalable database solution.
Among various programming languages, Java stands out as the preferred choice when dealing with NoSQL databases due to its powerful, platform-independent features. In this article, we will provide an introduction to Java and NoSQL databases, focusing on MongoDB and Cassandra.
What is NoSQL?
NoSQL, a term evolved from “Non SQL” or “Not Only SQL”, describes a type of database that provides a mechanism for storage and retrieval of data that diverges from the traditional tabular relations used in relational databases (RDBMS).
NoSQL databases are especially useful for working with large sets of distributed data. They come in four primary types – document, key-value, wide-column, and graph based. Each type is designed to manage specific types of data efficiently.
The predominant features of NoSQL databases include ease of replication processes, simple API’s, eventual consistency rather than instant consistency per transaction, and the ability to handle large amounts of unstructured data.
Java and NoSQL
Java stands as a mature, versatile language with well-established libraries and APIs. By binding Java and NoSQL, developers can leverage robust solutions to deal with immense and complex datasets. MongoDB and Cassandra are two popular NoSQL databases often used with Java.
MongoDB
MongoDB is an open-source, document-oriented database. It stores data in flexible documents similar to JSON, meaning no predefined document schema structure is necessary. This leads to easy storage of data of any structure directly. To connect Java with MongoDB, we use MongoDB Java Driver.
Here is a simple example of establishing a connection between Java and MongoDB:
import com.mongodb.*; public class MongoDBConnect { public static void main( String args[] ) { try{ MongoClient mongoClient = new MongoClient( "localhost" , 27017 ); System.out.println("Connect to MongoDB successfully"); DB db = mongoClient.getDB( "testDB" ); System.out.println("Database selected successfully"); } catch(Exception e){ System.err.println( e.getClass().getName() + ": " + e.getMessage() ); } } }
Cassandra
Apache Cassandra provides a highly scalable, distributed database system that ensures high availability and failover, with a flexible schema design. Cassandra is a column-oriented database, and it communicates over Java-native-client. Here is a minimal Java code demonstrating a connection with Cassandra:
import com.datastax.oss.driver.api.core.CqlSession; public class CassandraConnect { public static void main(String[] args) { try (CqlSession session = CqlSession.builder().build()) { System.out.println("Connect to Cassandra successfully"); } catch(Exception e){ System.err.println( e.getClass().getName() + ": " + e.getMessage() ); } } }
By exploring NoSQL databases in conjunction with Java, developers can design versatile and efficient systems for managing complex data. Both MongoDB and Cassandra offer their unique advantages, better suited to certain types of tasks and data. Be sure to choose the one that best fits the nature of your project and data needs.
Aspiring Java developers are encouraged not only to understand the basic commands but to explore and understand the NoSQL databases. This understanding will provide them with additional power and flexibility when it comes to handling big and complex datasets.