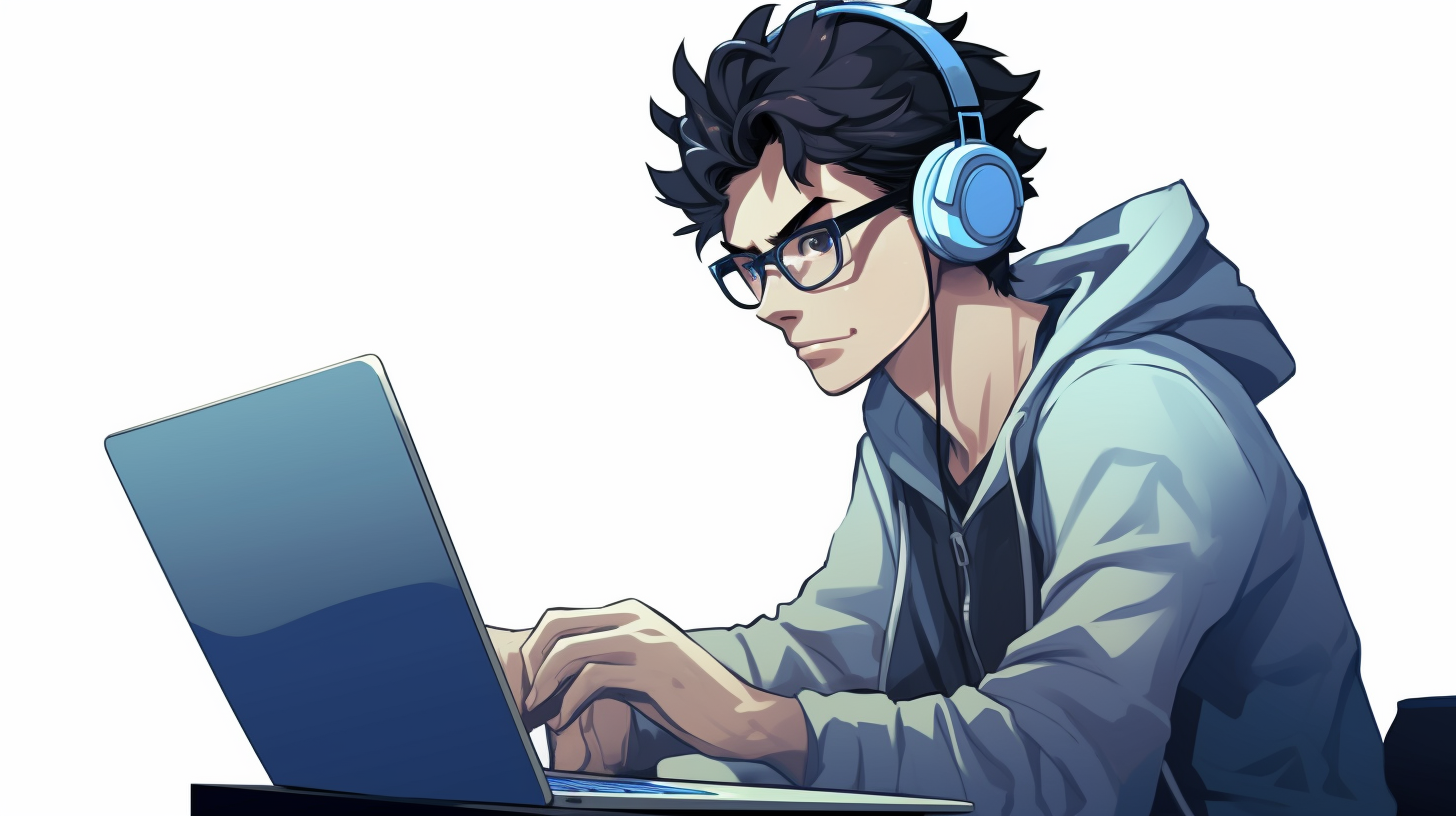
Java and Bioinformatics: An Introduction
Bioinformatics is a field that combines biology, computer science, and information technology to analyze and interpret biological data. It’s particularly important in genomics, where large amounts of DNA sequence data need to be processed and analyzed. Java is a popular programming language used in bioinformatics because it’s platform-independent, object-oriented, and has a vast array of libraries available for scientific computing and data analysis.
One of the key tasks in bioinformatics is to process and analyze sequence data. This can involve tasks such as sequence alignment, pattern searching, and phylogenetic analysis. Java provides several libraries and tools that can help with these tasks. For example, BioJava is an open-source library that provides tools for sequence analysis, protein structure analysis, and more.
To work with sequence data in Java, you first need to read in the sequence from a file. This can be done using the BioJava library, which provides classes for parsing common sequence file formats such as FASTA and GenBank. Here is an example of how to read a FASTA file using BioJava:
import org.biojava.nbio.core.sequence.io.FastaReaderHelper; import org.biojava.nbio.core.sequence.ProteinSequence; import java.io.File; import java.util.LinkedHashMap; public class ReadFastaFile { public static void main(String[] args) throws Exception { File file = new File("sequence.fasta"); LinkedHashMap proteinSequences = FastaReaderHelper.readFastaProteinSequence(file); for (String key : proteinSequences.keySet()) { System.out.println(">" + key); System.out.println(proteinSequences.get(key).getSequenceAsString()); } } }
Once you have read in the sequence data, you can perform various analyses on it. For example, you might want to search for a particular pattern in the sequence. This can be done using regular expressions in Java. Here is an example of how to search for a pattern in a DNA sequence:
import java.util.regex.Matcher; import java.util.regex.Pattern; public class PatternSearch { public static void main(String[] args) { String dnaSequence = "ATGCGATACGCTTGA"; String pattern = "ATG"; Pattern p = Pattern.compile(pattern); Matcher m = p.matcher(dnaSequence); while (m.find()) { System.out.println("Pattern found at index " + m.start()); } } }
Another common task in bioinformatics is sequence alignment. This involves comparing two or more sequences to identify regions of similarity. There are many algorithms for sequence alignment, such as the Needleman-Wunsch and Smith-Waterman algorithms. BioJava provides classes for performing sequence alignment. Here is an example of how to perform a pairwise sequence alignment using BioJava:
import org.biojava.nbio.alignment.Alignments; import org.biojava.nbio.alignment.Alignments.PairwiseSequenceAlignerType; import org.biojava.nbio.alignment.SimpleGapPenalty; import org.biojava.nbio.core.alignment.matrices.SubstitutionMatrixHelper; import org.biojava.nbio.core.alignment.template.GapPenalty; import org.biojava.nbio.core.alignment.template.SequencePair; import org.biojava.nbio.core.alignment.template.SubstitutionMatrix; import org.biojava.nbio.core.sequence.ProteinSequence; public class SequenceAlignment { public static void main(String[] args) { ProteinSequence s1 = new ProteinSequence("MGLSDGEWQLVLNVWGKVEADVAGHGQDILIRLFKSHPETLEKFDRFKHLKTEAEMKASEDLKKHGVTVLTALGAILKKKGHHEAELKPLAQSHATKHKIPVKYLEFISECIIQVLQSKHPGDFGADAQGAMNKALELFRNDIAAKYKELGYQG"); ProteinSequence s2 = new ProteinSequence("MGDVEKGKKIFIMKCSQCHTVEKGGKHKTGPNEKGKKIFVQKCSQCHTVLHGLFGRKTGQA"); SubstitutionMatrix matrix = SubstitutionMatrixHelper.getBlosum62(); GapPenalty penalty = new SimpleGapPenalty(); penalty.setOpenPenalty(10); penalty.setExtensionPenalty(1); SequencePair pair = Alignments.getPairwiseAlignment(s1, s2, PairwiseSequenceAlignerType.LOCAL, penalty, matrix); System.out.println(pair.toString()); } }
In summary, Java is a powerful tool for bioinformatics analysis. With its object-oriented design and extensive library support, Java allows bioinformaticians to write code that’s efficient, maintainable, and easy to understand. Whether you are working with sequence data, analyzing protein structures, or designing algorithms for genetic analysis, Java provides the necessary tools to get the job done.