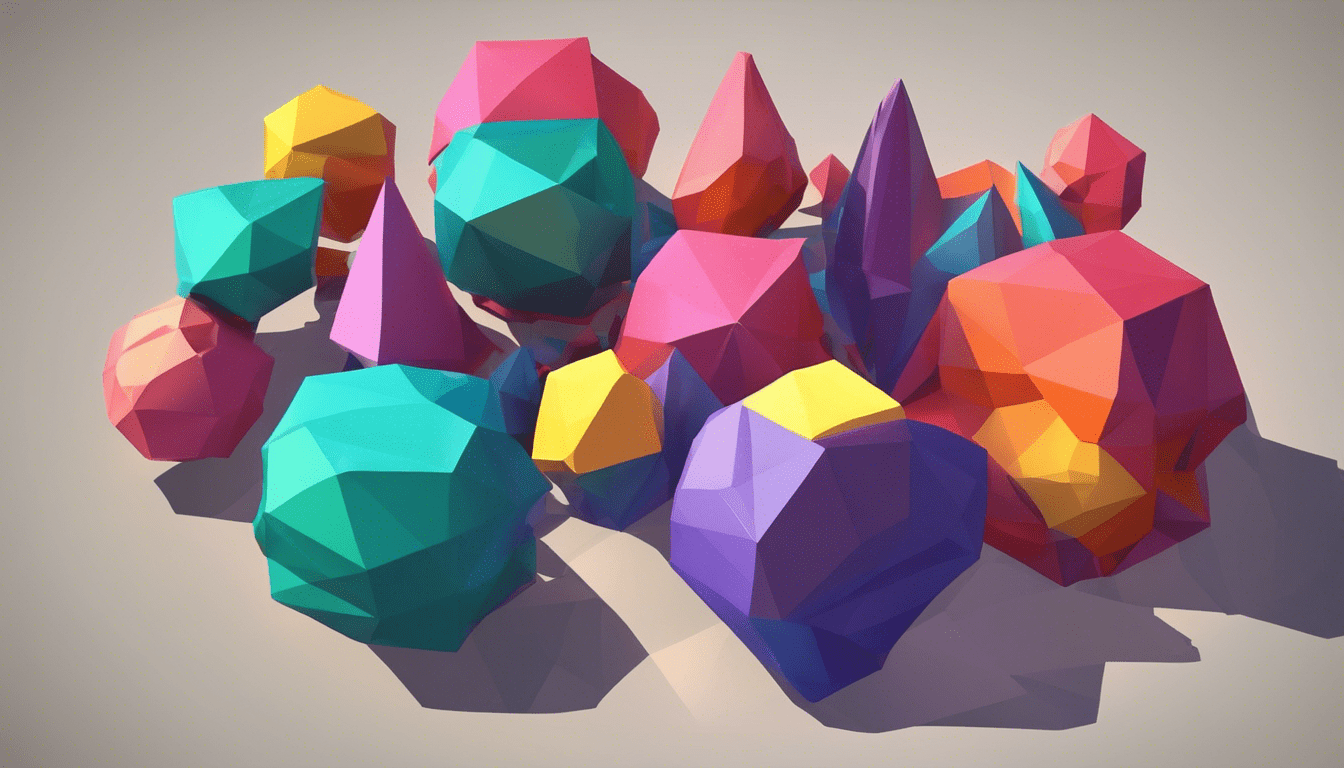
Java and Cloud Services: AWS, Azure, and More
Cloud services have become an integral part of state-of-the-art software development. Java developers can leverage cloud services from various providers such as AWS, Azure, and others to build scalable, reliable applications. With the help of cloud services, Java developers can focus on writing code without worrying about the underlying infrastructure.
AWS (Amazon Web Services) is one of the leading cloud service providers that offer a wide range of services for Java developers. AWS provides services like EC2 (Elastic Compute Cloud) for virtual servers, RDS (Relational Database Service) for database management, and S3 (Simple Storage Service) for storage solutions. To use AWS services in Java, developers need to use the AWS SDK for Java.
For example, to create an S3 bucket using the AWS SDK for Java, the following code can be used:
import com.amazonaws.services.s3.AmazonS3; import com.amazonaws.services.s3.AmazonS3ClientBuilder; import com.amazonaws.services.s3.model.CreateBucketRequest; import com.amazonaws.services.s3.model.Bucket; AmazonS3 s3Client = AmazonS3ClientBuilder.standard().build(); Bucket bucket = s3Client.createBucket(new CreateBucketRequest("my-new-bucket"));
To make sure the AWS SDK works correctly, developers need to provide their AWS credentials. This can be done by setting environment variables or by using a credentials file.
Azure, provided by Microsoft, is another popular cloud service platform. Azure offers services like Azure Virtual Machines for compute resources, Azure SQL Database for database management, and Azure Blob Storage for storing large amounts of unstructured data. Java developers can use the Azure SDK for Java to interact with these services.
For instance, to upload a file to Azure Blob Storage using the Azure SDK for Java, you can use this code:
import com.azure.storage.blob.BlobClient; import com.azure.storage.blob.BlobClientBuilder; BlobClient blobClient = new BlobClientBuilder() .connectionString("your-connection-string") .containerName("your-container-name") .blobName("your-blob-name") .buildClient(); blobClient.uploadFromFile("path-to-your-file");
When using Azure SDK, similar to AWS, you need to provide the credentials which could be managed identities for Azure resources, service principal with a client secret, or a user principal with multi-factor authentication.
Besides AWS and Azure, there are other cloud service providers like Google Cloud Platform (GCP), IBM Cloud, Oracle Cloud Infrastructure (OCI), and more. Each of these providers has their own set of services and tools that Java developers can utilize.
To wrap it up, cloud services offer Java developers a powerful way to create and deploy applications efficiently. By leveraging services from providers like AWS and Azure, developers can minimize the complexity of infrastructure management and focus on developing high-quality applications. With a basic understanding of these services and the associated SDKs, even beginner Java developers can start incorporating cloud services into their projects.
One important aspect that wasn’t mentioned in the article is the importance of understanding the pricing models of these cloud services. AWS, Azure, and other providers charge based on usage, so it’s crucial for Java developers to monitor their application’s resource consumption to avoid unexpected costs. Additionally, developers should consider the security measures provided by these services, such as data encryption and access control, to protect sensitive information.