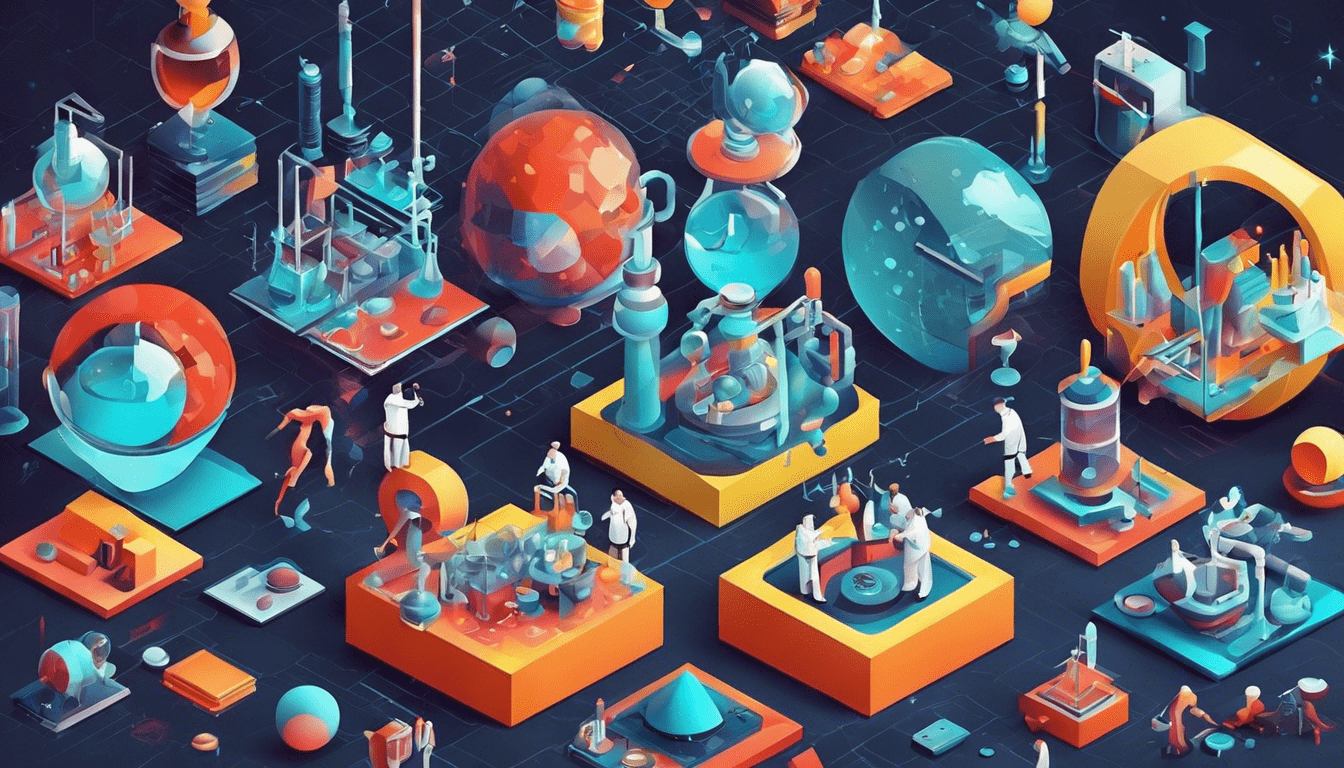
Java and Serverless Computing
Serverless computing is a cloud-computing execution model where the cloud provider dynamically manages the allocation and provisioning of servers. It’s a form of utility computing that can help developers execute code in response to events without managing the underlying infrastructure.
Java, being one of the most popular programming languages, is widely used in serverless computing platforms such as AWS Lambda. AWS Lambda is an event-driven, serverless computing platform provided by Amazon as a part of Amazon Web Services.
When using Java with serverless computing, it’s important to understand how these functions are structured and how they work. A typical AWS Lambda function in Java includes a handler that acts as the entry point for your code. This handler implements certain interfaces provided by AWS Lambda, such as RequestHandler or RequestStreamHandler.
import com.amazonaws.services.lambda.runtime.Context; import com.amazonaws.services.lambda.runtime.RequestHandler; public class LambdaFunctionHandler implements RequestHandler<String, String> { @Override public String handleRequest(String input, Context context) { context.getLogger().log("Input: " + input); // perform operations String output = "Hello, " + input + "!"; return output; } }
In the above example, the LambdaFunctionHandler
class implements RequestHandler
. The handleRequest method takes two parameters: input and context. The input is the data this is passed to your Lambda function and can be of any type that you define (in this case, it is a String
). The context provides information about the invocation, function, and execution environment.
Once you have written your Lambda function in Java, you can deploy it to AWS Lambda by packaging it as a .jar or .zip file and uploading it through the AWS Management Console or AWS CLI.
Here’s a basic guide on how to create a deployable package for your Java Lambda function:
- Compile your Java code with Maven or Gradle.
- Package your compiled code and any dependencies into a .jar or .zip file.
- Upload your package to AWS Lambda.
Note: When working with AWS Lambda and Java, you must include the AWS SDK for Java in your project to interact with AWS services.
com.amazonawsaws-lambda-java-core1.2.0
Serverless computing has several benefits including reduced operational costs since you don’t need to manage servers, improved scalability because resources are allocated dynamically based on demand, and faster deployments because you only need to worry about your code, not the entire application stack.
To summarize, Java is a powerful language for serverless computing, particularly with AWS Lambda. Understanding the basics of creating and deploying Java functions to a serverless platform can open doors to scalable, cost-effective, and event-driven architectures.
One important aspect that the article does not mention is the cold start problem that can occur with serverless computing. That is when a function has not been used for a while and takes longer to start up, which can impact performance. It is important for developers to be aware of this potential issue and consider strategies to mitigate it, such as keeping functions warm or using provisioned concurrency. Additionally, developers should think the limitations and restrictions of serverless computing, such as execution time limits and memory constraints, when designing their applications.