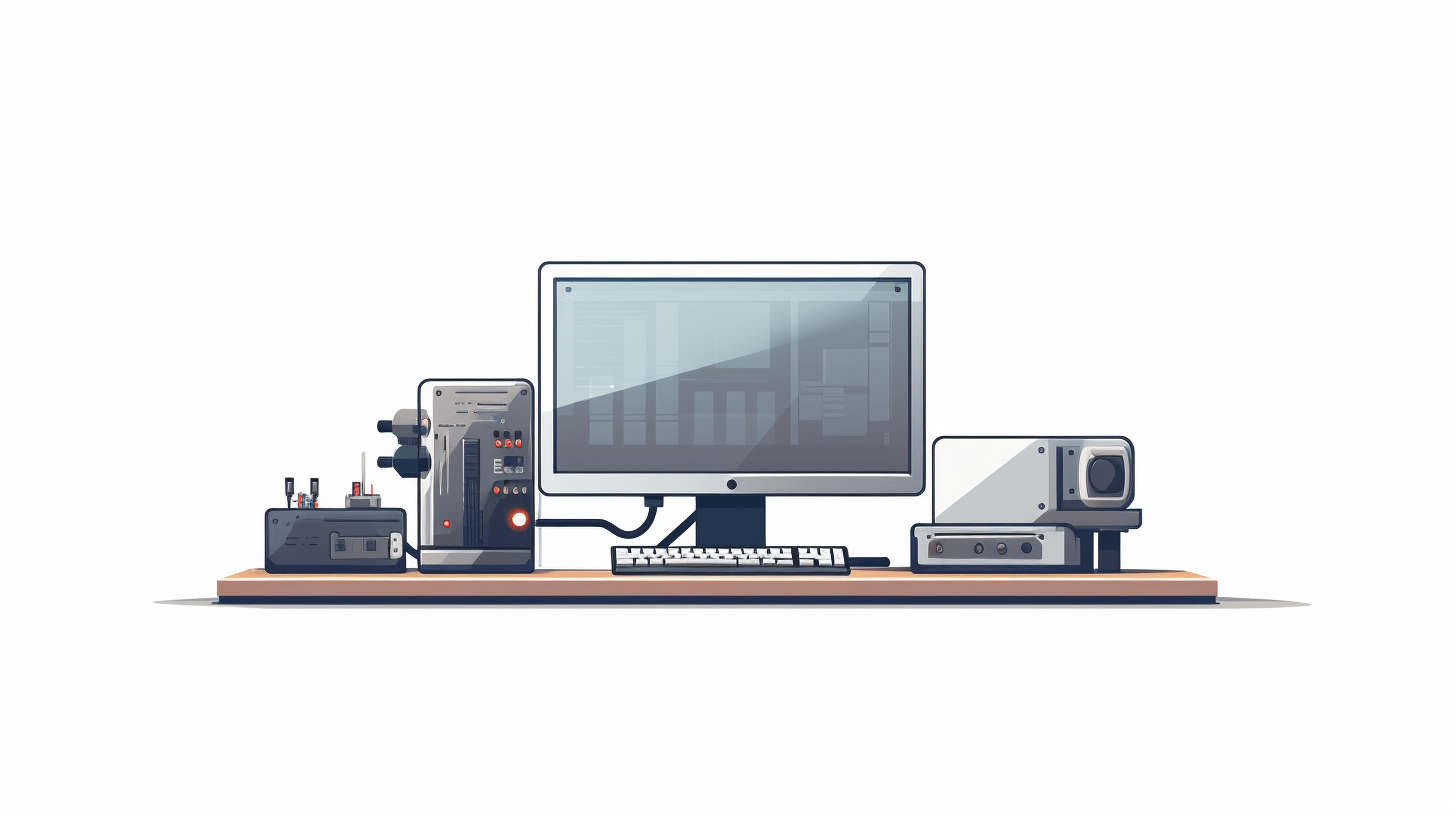
Java Security: Best Practices and Encryption
When it comes to developing applications in Java, security is a critical aspect that every developer should ponder. Security involves protecting your application from unauthorized access, data breaches, and other malicious activities. This article will discuss Java security best practices and encryption techniques that you can implement in your Java applications.
Best Practices for Java Security
- Always keep your Java development kit (JDK) and runtime environment (JRE) up to date with the latest security patches. This reduces the risk of exploitation of known vulnerabilities.
- Implement strong authentication and authorization mechanisms. Ensure that users are who they claim to be and that they only have access to resources they’re permitted to use.
- Always validate user input to protect against SQL injection and other types of attacks. Never trust the data coming from users without checking it first.
- Follow secure coding guidelines to avoid common pitfalls that can lead to security vulnerabilities, such as buffer overflows or cross-site scripting (XSS).
- A security manager is a class that allows applications to implement a security policy. It acts as a guard against unauthorized actions by your code.
Encryption in Java
Encryption is the process of converting readable data into an unreadable format to protect it from unauthorized access. In Java, you can use various encryption algorithms provided by the Java Cryptography Architecture (JCA). Here we’ll look at two main types of encryption: symmetric and asymmetric.
- Also known as private-key encryption, this method uses a single key for both encryption and decryption. An example of a symmetric encryption algorithm is AES (Advanced Encryption Standard).
- Also known as public-key encryption, this method uses two different keys – one for encryption (public key) and one for decryption (private key). An example of an asymmetric encryption algorithm is RSA.
Encrypting Data Using AES in Java
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(128); // 128-bit AES SecretKey secretKey = keyGenerator.generateKey();
Cipher cipher = Cipher.getInstance("AES"); cipher.init(Cipher.ENCRYPT_MODE, secretKey);
String plainText = "This is a secret message"; byte[] encryptedBytes = cipher.doFinal(plainText.getBytes());
cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedBytes = cipher.doFinal(encryptedBytes); String decryptedString = new String(decryptedBytes);
Using SSL/TLS for Secure Communication
If your Java application communicates over a network, it is essential to secure the data transmission using SSL/TLS protocols. SSL (Secure Sockets Layer) and TLS (Transport Layer Security) provide a secure channel between two machines operating over the internet or an internal network.
To implement SSL/TLS in your Java application:
- Use
SSLSocket
for secure socket communication. - Configure your server with an SSL certificate.
- When dealing with HTTPS requests, use
HttpsURLConnection
.
In conclusion, Java security is a vast field, but following best practices such as keeping Java updated, using strong access controls, validating inputs, adhering to secure coding practices, and utilizing security managers can significantly enhance your application’s security posture. Furthermore, encrypting sensitive data and securing communication channels with SSL/TLS are important steps in protecting data integrity and privacy. By understanding and implementing these concepts, developers can build more robust and secure Java applications.