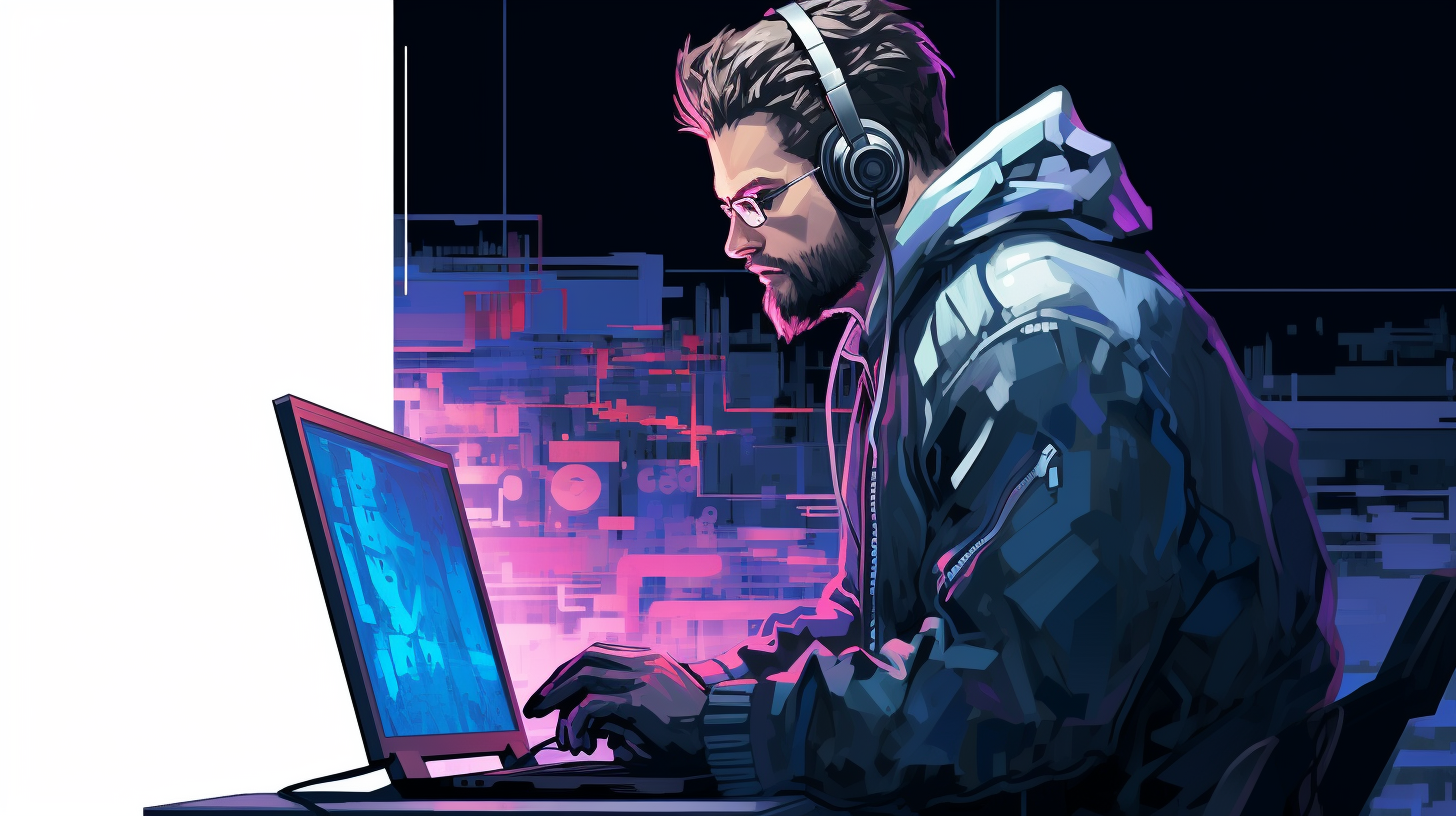
JavaScript and AJAX for Asynchronous Calls
Asynchronous programming in JavaScript is a critical paradigm that enables non-blocking operations, allowing developers to create smooth and responsive web applications. Unlike synchronous programming, where tasks are executed sequentially and the execution halts until each task completes, asynchronous programming allows JavaScript to initiate a process and move on to the next task, tackling the original task’s result at a later point in time.
In JavaScript, the primary mechanism for handling asynchronous operations includes callbacks, promises, and the more recent async/await syntax. Understanding these concepts is essential for creating efficient and responsive applications.
Callbacks are functions passed as arguments to other functions, to be executed once a certain operation completes. This method, while simpler, can lead to complex and hard-to-manage code, famously known as “callback hell”.
function fetchData(callback) { setTimeout(() => { const data = { message: "Data loaded!" }; callback(data); }, 1000); } fetchData((data) => { console.log(data.message); // Outputs: Data loaded! });
To mitigate the complications of callback hell, promises were introduced. A promise represents a value that may be available now, or in the future, or never. They allow for better chaining of operations and error handling.
const fetchDataPromise = () => { return new Promise((resolve) => { setTimeout(() => { resolve({ message: "Data loaded!" }); }, 1000); }); }; fetchDataPromise() .then(data => { console.log(data.message); // Outputs: Data loaded! }) .catch(error => { console.error("Error:", error); });
Finally, the async/await syntax builds on promises by allowing asynchronous code to be written in a more synchronous fashion, making it easier to read and maintain. The async
keyword defines a function as asynchronous, while await
pauses the execution of the function until the promise settles.
const fetchDataAsync = async () => { const data = await fetchDataPromise(); console.log(data.message); // Outputs: Data loaded! }; fetchDataAsync();
The Role of AJAX in Modern Web Development
AJAX, or Asynchronous JavaScript and XML, plays a pivotal role in modern web development by enabling the creation of dynamic and interactive web applications. With AJAX, web applications can communicate with a server asynchronously without reloading the entire web page, leading to a smoother and faster user experience. This ability to send and receive data in the background allows developers to create more responsive interfaces that feel like native applications.
At its core, AJAX utilizes a combination of JavaScript and the XMLHttpRequest (XHR) object, or more recently, the Fetch API. The XMLHttpRequest object allows developers to make HTTP requests to the server and handle the responses, all while maintaining the current state of the web page. This means that data can be fetched, submitted, or updated without requiring a full page refresh.
For instance, when a user fills out a form and submits it, AJAX can send the form data to the server in the background, allowing the user to continue interacting with the application while waiting for the server’s response. This capability is essential for applications that require real-time data updates, such as chat applications, live notifications, or content management systems.
Here’s a simple example of making an AJAX call using the XMLHttpRequest object:
const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data', true); xhr.onreadystatechange = function () { if (xhr.readyState === 4 && xhr.status === 200) { const responseData = JSON.parse(xhr.responseText); console.log(responseData); } }; xhr.send();
In this example, we create a new XMLHttpRequest object and specify the HTTP method (GET) and the URL from which we want to fetch data. We then define a callback function that will handle the server’s response. Once the request is complete and successful, we parse the JSON response and log it to the console.
With the advent of the Fetch API, making asynchronous calls has become even more streamlined and intuitive. The Fetch API returns a promise that resolves to the Response object representing the response to the request. This enhances error handling and allows for a more simpler approach to handling responses.
Here’s how we can achieve the same result using the Fetch API:
fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { console.log(data); }) .catch(error => { console.error('There was a problem with the fetch operation:', error); });
This code snippet demonstrates the clarity and simplicity of the Fetch API. The promise-based structure allows for chaining, making it easier to read and understand. Error handling is also simplified, as we can catch any issues that arise during the request process.
Making Your First Asynchronous Call with AJAX
Making your first asynchronous call with AJAX is an exciting step that opens up a world of interactive web application possibilities. In this section, we’ll dive into the practicalities of initiating an asynchronous request, examining both the traditional XMLHttpRequest method and the modern Fetch API.
To get started with AJAX, you’ll want to focus on the XMLHttpRequest object, which serves as the backbone of traditional AJAX calls. The following code snippet illustrates how to create a basic GET request to retrieve data from a server:
const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data', true); xhr.onreadystatechange = function () { if (xhr.readyState === 4) { if (xhr.status === 200) { const responseData = JSON.parse(xhr.responseText); console.log(responseData); } else { console.error('Error fetching data:', xhr.statusText); } } }; xhr.send();
In this code, we start by creating a new instance of the XMLHttpRequest object. We specify the HTTP method—here, ‘GET’—and the URL from which we wish to retrieve data. By setting the third parameter to true, we configure the request to be asynchronous.
Next, we define a callback function using the onreadystatechange event handler. This function checks if the request has completed by assessing the readyState property. A value of 4 indicates that the operation is complete. We then check for a successful response using the status property. If successful, we parse the JSON response and log it. If there’s an error, we output an error message.
However, as web development has evolved, so too has the approach to making asynchronous calls. The Fetch API, introduced in modern browsers, simplifies this process significantly. With the Fetch API, we can send requests and handle responses using the promise-based syntax, making our code cleaner and more intuitive. Here’s how you can use the Fetch API to achieve the same effect:
fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { console.log(data); }) .catch(error => { console.error('There was a problem with the fetch operation:', error); });
This example shows the ease of use that comes with the Fetch API. We initiate a request to the same endpoint, and the Fetch API automatically returns a promise that resolves to the response object. By checking the response’s ok property, we can quickly ascertain whether the request was successful before proceeding to parse the JSON data. The promise chain allows us to handle both success and error cases seamlessly.
Handling Responses and Errors in AJAX Requests
When working with AJAX, one of the most critical aspects is handling responses and errors effectively. Properly managing the responses from your server can make the difference between a smooth user experience and a frustrating one. In this section, we will explore how to handle responses effectively, as well as how to manage errors gracefully in both XMLHttpRequest and Fetch API contexts.
With the traditional XMLHttpRequest approach, handling the server’s response is done through the onreadystatechange event. Within this event, you need to check both the readyState and status properties to ensure that the request has completed successfully. Here’s a more detailed example demonstrating this:
const xhr = new XMLHttpRequest(); xhr.open('GET', 'https://api.example.com/data', true); xhr.onreadystatechange = function () { if (xhr.readyState === 4) { // Check if the request is complete if (xhr.status === 200) { // Check for a successful response try { const responseData = JSON.parse(xhr.responseText); console.log(responseData); // Process the response data } catch (error) { console.error('Error parsing JSON:', error); } } else { console.error('Error fetching data:', xhr.status, xhr.statusText); } } }; xhr.send();
In this example, if the server responds with a status code of 200, indicating success, we attempt to parse the response as JSON. If any error occurs during parsing, we catch it and log it. If the request fails, we log the status code and status text for debugging purposes.
When using the Fetch API, handling responses and errors becomes more streamlined due to its promise-based structure. Here’s how you can handle responses and potential errors using Fetch:
fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok: ' + response.status); } return response.json(); }) .then(data => { console.log(data); // Process the data }) .catch(error => { console.error('There was a problem with the fetch operation:', error); });
In this Fetch example, we first check if the response is ok using the ok property, which is true for status codes between 200 and 299. If the response isn’t okay, we throw an error that can be caught in the subsequent catch block. This not only simplifies error handling but also ensures that any issues will result in a clear and concise error message.
Handling errors gracefully is essential for maintaining a good user experience. Whether using XMLHttpRequest or Fetch, always anticipate potential issues, such as network failures or server errors, and provide clear feedback to the user. This practice not only aids in debugging but also enhances the robustness of your application.
Best Practices for Efficient Asynchronous Programming
Efficient asynchronous programming very important in crafting modern web applications that are not just functional but also responsive and effortless to handle. Here are some best practices that can elevate your asynchronous JavaScript code and ensure it runs smoothly, enhancing performance and maintainability.
1. Use Async/Await for Readability: One of the most effective ways to manage asynchronous code is by using the async/await syntax. It eliminates the complexity often associated with promise chains and callbacks, making the code easier to read and maintain.
const fetchData = async () => { try { const response = await fetch('https://api.example.com/data'); if (!response.ok) { throw new Error('Network response was not ok: ' + response.status); } const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching data:', error); } }; fetchData();
This approach maintains a clean structure that resembles synchronous code, making it easier for developers to follow the flow of execution. Error handling is also neatly encapsulated within a try/catch block, providing a robust strategy to manage unforeseen issues.
2. Limit Concurrent Requests: While JavaScript’s asynchronous nature allows multiple requests to be sent simultaneously, flooding your server with too many requests concurrently can lead to performance degradation. It may also overwhelm the server, leading to dropped requests or slower responses. Consider implementing a concurrency limit to ensure your application behaves optimally.
const fetchWithLimit = async (urls, limit) => { const results = []; const executing = new Set(); for (const url of urls) { const promise = fetch(url).then(response => response.json()); results.push(promise); executing.add(promise); if (executing.size >= limit) { await Promise.race(executing); } executing.delete(promise); } return Promise.all(results); }; const urls = ['https://api.example.com/data1', 'https://api.example.com/data2', 'https://api.example.com/data3']; fetchWithLimit(urls, 2).then(data => console.log(data));
This sample illustrates how to limit the number of concurrent requests to a specified threshold. The use of a Set
helps manage active promises efficiently, allowing the program to wait for the first request to complete before sending more requests.
3. Optimize Network Requests: Minimize the number of network requests by combining multiple API calls into a single request when possible. This can significantly reduce latency and improve the overall efficiency of the application. For example, if you’re fetching related data, ponder creating an endpoint that aggregates this information.
const fetchCombinedData = async () => { try { const response = await fetch('https://api.example.com/combined-data'); if (!response.ok) { throw new Error('Network response was not ok: ' + response.status); } const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching combined data:', error); } }; fetchCombinedData();
By aggregating data requests into a single call, we minimize the network overhead and cut down on the number of round trips to the server.
4. Implement Caching Strategies: To improve performance, think caching the results of expensive API calls. By storing responses in memory or using localStorage, you can avoid unnecessary network requests and improve loading times.
const cache = new Map(); const fetchWithCache = async (url) => { if (cache.has(url)) { return cache.get(url); } const response = await fetch(url); if (!response.ok) { throw new Error('Network response was not ok: ' + response.status); } const data = await response.json(); cache.set(url, data); return data; }; fetchWithCache('https://api.example.com/data').then(data => console.log(data));
Here, we check the cache before making a network request. If the data exists in the cache, we return it immediately, thereby speeding up the response time for the user.
5. Handle Errors Gracefully: Error handling is an integral part of asynchronous programming. Ensure that you not only catch errors but also provide meaningful feedback to users. Use easy to use error messages and ponder implementing a retry mechanism for transient errors.
const fetchWithRetry = async (url, retries = 3) => { for (let i = 0; i console.log(data)).catch(error => console.error('Failed to fetch:', error));
This example demonstrates a simple retry mechanism. If a fetch operation fails, it will retry a specified number of times before ultimately throwing an error. This can help mitigate issues due to temporary network problems.