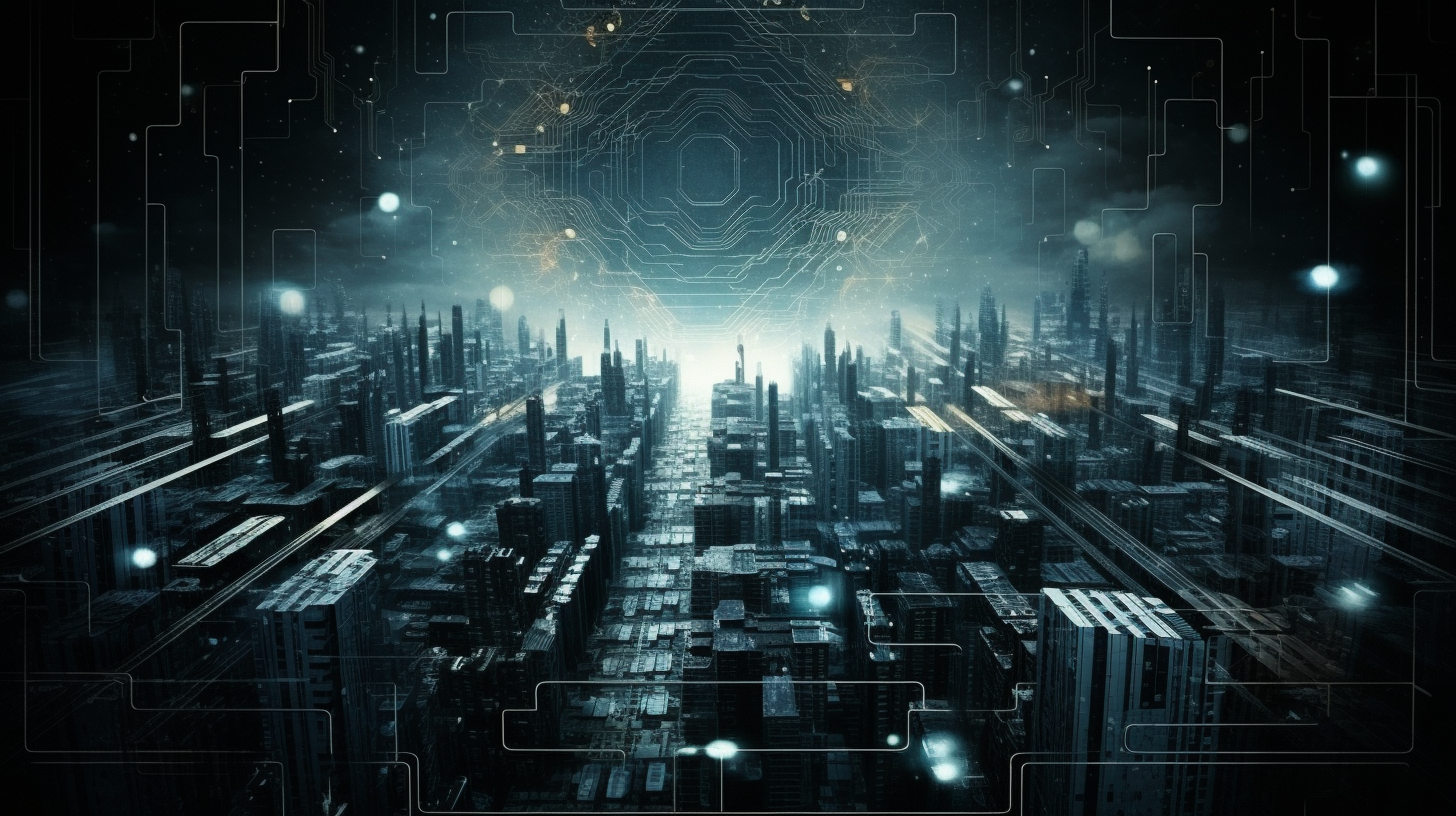
JavaScript and Cryptocurrency Applications
JavaScript, a high-level programming language, has become an essential tool for developing various cryptocurrency applications. Its versatility and event-driven nature make it particularly well-suited for handling the asynchronous operations that are common in the world of digital currencies. JavaScript’s ability to run both on the client-side and server-side, thanks to environments like Node.js, enables developers to create full-fledged cryptocurrency applications that are efficient and scalable.
One of the primary uses of JavaScript in cryptocurrency is in the creation of web-based wallets and user interfaces for interacting with blockchain networks. By using JavaScript frameworks like React or Angular, developers can build responsive and dynamic front-end applications that allow users to manage their digital assets conveniently.
Another significant application of JavaScript in cryptocurrency is in the development of decentralized applications (dApps). These are applications that run on a blockchain network rather than a single centralized server. JavaScript, in conjunction with blockchain-specific libraries and APIs, allows developers to create dApps that provide various services, such as decentralized exchanges, tokenization platforms, or gaming applications.
Here’s a simple example of how JavaScript can be used to interact with a blockchain network:
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_API_KEY'); web3.eth.getBlockNumber().then((result) => { console.log('Current block number:', result); });
In the above code snippet, we are using the popular Web3.js library, which provides a collection of modules for interacting with a local or remote Ethereum node using HTTP, IPC, or WebSocket. By calling web3.eth.getBlockNumber()
, we can retrieve the current block number on the Ethereum blockchain. That’s a simple demonstration of how JavaScript can be used to query blockchain data.
As the cryptocurrency space continues to evolve, JavaScript’s role is likely to grow even further. Its ability to integrate with other technologies and its vast ecosystem of libraries and frameworks make it an ideal choice for building the next generation of cryptocurrency applications.
Building Cryptocurrency Wallets with JavaScript
Building cryptocurrency wallets is one of the most practical applications of JavaScript in the cryptocurrency space. A wallet is essentially a software program that stores private and public keys and interacts with various blockchains to enable users to send and receive digital currency and monitor their balance. JavaScript’s capabilities allow developers to create secure and user-friendly wallets that can operate on different devices and platforms.
To build a cryptocurrency wallet with JavaScript, developers usually start by creating the wallet’s user interface. This can be done using JavaScript frameworks such as React or Vue.js, which provide a modular approach to building web applications. The user interface needs to be intuitive and uncomplicated to manage, allowing users to perform actions such as creating a new wallet, importing an existing wallet, sending and receiving cryptocurrency, and viewing transaction history.
Here’s an example of how a simple wallet interface can be created using React:
import React, { useState } from 'react'; const Wallet = () => { const [balance, setBalance] = useState(0); const handleSend = (amount, address) => { // Logic to send cryptocurrency }; const handleReceive = (amount) => { setBalance(balance + amount); }; return (
After setting up the user interface, the next step is to integrate the wallet with the blockchain. This is where JavaScript libraries like Web3.js come into play. Web3.js allows the wallet to communicate with a blockchain network, enabling it to perform transactions and query blockchain data.
For example, to send Ether using Web3.js, you would use the following code:
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_API_KEY'); const senderAddress = 'sender-address'; const recipientAddress = 'recipient-address'; const privateKey = 'sender-private-key'; const transaction = { to: recipientAddress, value: web3.utils.toWei('1', 'ether'), gas: 21000, }; web3.eth.accounts.signTransaction(transaction, privateKey) .then(signedTx => web3.eth.sendSignedTransaction(signedTx.rawTransaction)) .then(receipt => console.log('Transaction receipt:', receipt)) .catch(err => console.error(err));
Security is paramount when building cryptocurrency wallets. Developers must ensure that private keys are stored securely and that the wallet is protected against various types of attacks. This can involve using secure storage solutions, implementing two-factor authentication, and following best practices for cryptographic operations.
Building cryptocurrency wallets with JavaScript involves creating a easy to use interface, integrating with blockchain networks using libraries like Web3.js, and ensuring the security of the users’ keys and funds. With JavaScript’s flexibility and the rich ecosystem of libraries and frameworks available, developers have all the tools they need to create robust and secure cryptocurrency wallets.
Implementing Smart Contracts with JavaScript
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They run on a blockchain network and automatically enforce and execute the terms of a contract when certain conditions are met. JavaScript, with its event-driven nature and asynchronous capabilities, is well-suited for implementing smart contracts, especially on platforms like Ethereum that support the Ethereum Virtual Machine (EVM).
To implement a smart contract with JavaScript, developers typically use a combination of Solidity, the programming language for writing smart contracts on Ethereum, and JavaScript libraries like Web3.js or ethers.js to interact with the Ethereum network. Here’s a step-by-step guide to creating a simple smart contract using Solidity and deploying it with JavaScript using Web3.js:
pragma solidity ^0.8.0; contract SimpleStorage { uint storedData; function set(uint x) public { storedData = x; } function get() public view returns (uint) { return storedData; }
Use a tool like Truffle, Remix, or solc-js to compile the Solidity code to bytecode that can be deployed to the Ethereum network.
const Web3 = require('web3'); const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_API_KEY'); const contractABI = []; // ABI generated from the compilation const contractBytecode = '0x...'; // Bytecode generated from the compilation const deployContract = async () => { const accounts = await web3.eth.getAccounts(); const result = await new web3.eth.Contract(contractABI) .deploy({ data: contractBytecode }) .send({ from: accounts[0], gas: 1500000, gasPrice: '30000000000' }); console.log('Contract deployed at address:', result.options.address); }; deployContract().catch(console.error);
The JavaScript code above uses Web3.js to deploy the compiled smart contract to the Ethereum network. You need to replace YOUR_INFURA_API_KEY
with your actual Infura API key, and contractABI
and contractBytecode
with the actual ABI and bytecode output from the Solidity compilation.
Once the smart contract is deployed, you can interact with it using JavaScript. For instance, to call the set
and get
functions of the SimpleStorage
contract, you would use the following code:
const contractAddress = 'deployed-contract-address'; const storageContract = new web3.eth.Contract(contractABI, contractAddress); const setValue = async (value) => { const accounts = await web3.eth.getAccounts(); await storageContract.methods.set(value).send({ from: accounts[0] }); }; const getValue = async () => { const value = await storageContract.methods.get().call(); console.log('Stored value:', value); }; setValue(42).catch(console.error); getValue().catch(console.error);
In the code above, we are creating an instance of the smart contract using its ABI and address, and then we’re calling its set
and get
functions.
Implementing smart contracts with JavaScript allows developers to create decentralized applications that are secure, transparent, and run without the need for intermediaries. By combining Solidity for smart contract creation and JavaScript for deployment and interaction, developers can harness the full potential of blockchain technology.
Securing Cryptocurrency Transactions with JavaScript Libraries
When it comes to securing cryptocurrency transactions, JavaScript libraries play an important role. They provide the necessary tools to ensure that transactions are not only processed correctly but also protected from potential threats. In this section, we’ll explore some of the JavaScript libraries that are instrumental in securing cryptocurrency transactions and how they can be utilized within your applications.
1. Crypto-js
Crypto-js is a popular JavaScript library for cryptography. It provides a variety of cryptographic algorithms and utilities that can be used to secure transactions. For instance, you can use Crypto-js to hash transaction details before sending them over the network, ensuring the integrity of the data.
const CryptoJS = require("crypto-js"); const transaction = { from: "Alice", to: "Bob", amount: 10 }; const hash = CryptoJS.SHA256(JSON.stringify(transaction)).toString(); console.log(hash);
2. Bitcore-lib
Bitcore-lib is a JavaScript library for Bitcoin protocol. It provides a robust set of tools to manage Bitcoin transactions, including creating, signing, and verifying transactions. Using bitcore-lib, developers can build applications that handle Bitcoin transactions with high levels of security.
const bitcore = require('bitcore-lib'); const privateKey = new bitcore.PrivateKey(); const utxo = { "txId" : "transaction-id", "outputIndex" : 0, "address" : "public-address", "script" : "script", "satoshis" : 100000 }; const transaction = new bitcore.Transaction() .from(utxo) .to('destination-address', 15000) .sign(privateKey); console.log(transaction.toString());
3. Ethereumjs-tx
For Ethereum-based transactions, ethereumjs-tx is a useful library. It allows you to create, manipulate, and sign Ethereum transactions. It supports both standard transactions and those with complex smart contract interactions.
const EthereumTx = require('ethereumjs-tx').Transaction; const privateKey = Buffer.from('private-key', 'hex'); const txParams = { nonce: '0x00', gasPrice: '0x09184e72a000', gasLimit: '0x2710', to: 'recipient-address', value: '0x00', data: '0x0' }; const tx = new EthereumTx(txParams); tx.sign(privateKey); const serializedTx = tx.serialize(); console.log('Transaction:', serializedTx.toString('hex'));
Securing cryptocurrency transactions involves more than just the correct handling of the transaction data. It also requires protection against common vulnerabilities and attacks such as double-spending, replay attacks, and phishing. JavaScript libraries like Crypto-js, bitcore-lib, and ethereumjs-tx provide developers with the necessary tools to secure transactions and safeguard the integrity of their cryptocurrency applications.
By integrating these libraries into your JavaScript applications, you can ensure that your cryptocurrency transactions are not only smooth and efficient but also secure from potential threats. As the cryptocurrency landscape continues to evolve, the importance of security cannot be overstated, and these JavaScript libraries will remain vital in maintaining the trust and reliability of digital currency transactions.