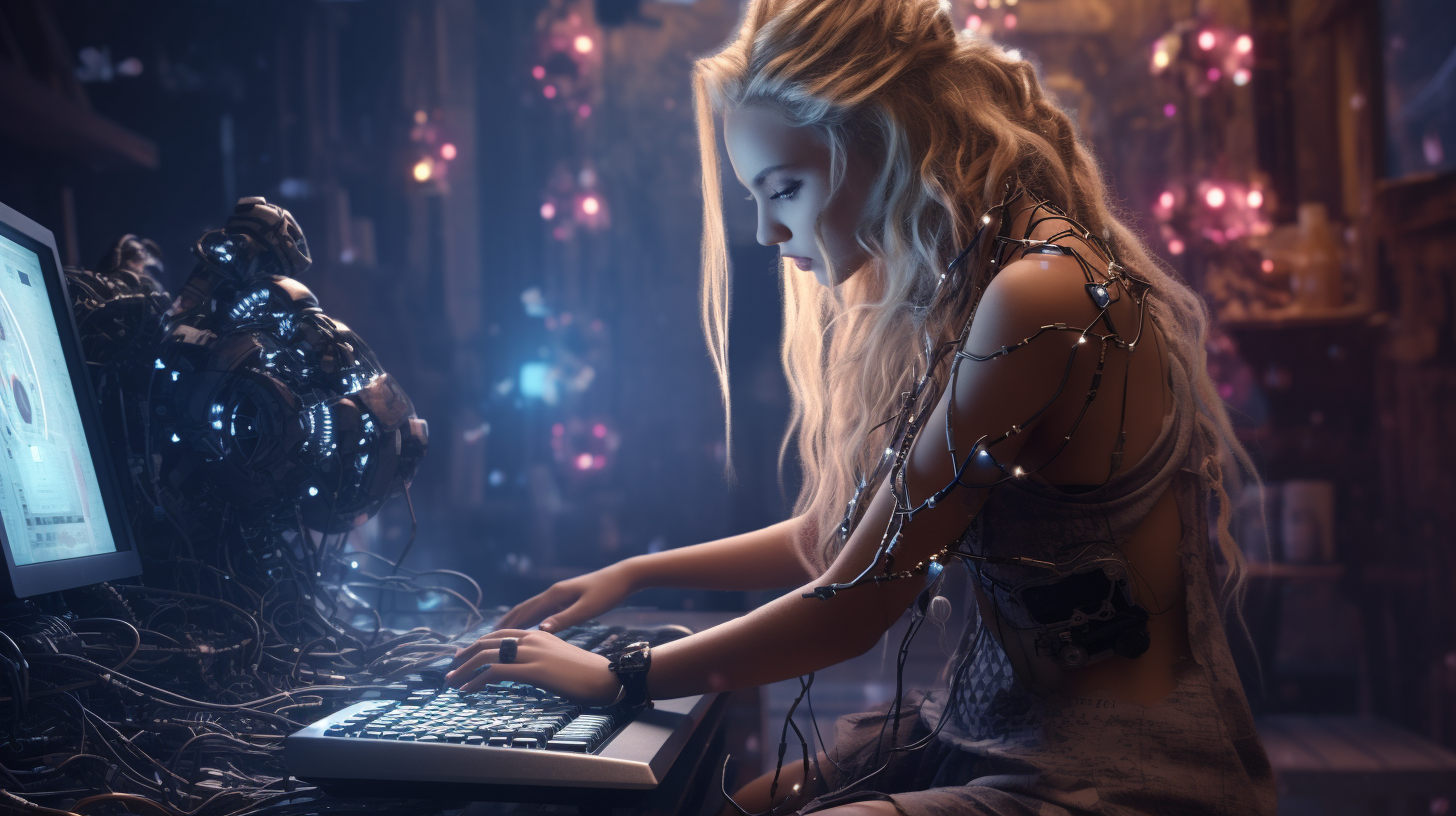
JavaScript and Online Payment Systems
Overview of JavaScript in Online Payment Systems
JavaScript plays an important role in the functionality of online payment systems, providing a seamless and interactive user experience. It is often used to create dynamic payment forms, validate user input, and communicate with payment gateways via APIs. One of the core advantages of using JavaScript is its ability to run on the client-side, reducing server load and speeding up transaction processing.
For instance, when a user enters their payment details on a website, JavaScript can be used to validate the information in real-time before it’s sent to the server. This immediate feedback is valuable in preventing errors and ensuring the correct data is collected. An example of a simple validation check for a credit card number might look like this:
function validateCardNumber(number) { var regex = new RegExp("^[0-9]{16}$"); if (!regex.test(number)) { alert("Invalid card number!"); return false; } return true; }
In addition to validation, JavaScript interfaces with payment gateways through the use of APIs. This allows for secure processing of credit card transactions without sensitive information ever touching the merchant’s server. A typical API call to process a payment might include code similar to the following:
function processPayment(details) { fetch('https://api.paymentgateway.com/v1/payments', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify(details), }) .then(response => response.json()) .then(data => { console.log('Payment successful:', data); }) .catch((error) => { console.error('Payment failed:', error); }); }
It’s important to note that while JavaScript is powerful in managing client-side interactions, all sensitive payment information should be handled in a secure, PCI-compliant manner. This typically involves tokenization and encryption of credit card data before it’s transmitted over the internet.
Security is also a top priority when integrating JavaScript with online payment systems. Developers must ensure they are following best practices to protect against common vulnerabilities such as cross-site scripting (XSS) and injection attacks. This often includes encoding and properly escaping user input, as well as implementing Content Security Policy (CSP) headers.
Overall, JavaScript’s versatility and efficiency make it an invaluable asset in the realm of online payments, enabling developers to create robust, effortless to handle, and secure payment processes.
Benefits of Using JavaScript for Online Payments
One of the primary benefits of using JavaScript for online payments is its flexibility and ease of integration. JavaScript can be easily incorporated into existing websites and applications, allowing for the addition of payment functionality without the need for extensive redevelopment. This makes it an ideal choice for businesses looking to quickly and efficiently expand their payment options.
Another significant advantage is the enhanced user experience JavaScript provides. With its ability to update the user interface without reloading the entire page, JavaScript ensures a smooth and uninterrupted payment process. This not only improves customer satisfaction but also helps reduce cart abandonment rates, which can directly impact a business’s bottom line.
JavaScript also allows for customization and personalization of the payment process. Developers can use JavaScript to create custom payment forms that match the look and feel of their website, providing a cohesive and branded experience. Additionally, JavaScript can be used to implement features like coupon code validation and dynamic pricing updates, further enhancing the user experience.
Security is another area where JavaScript shines. While handling sensitive payment information requires careful consideration, JavaScript provides tools and frameworks that help ensure data is handled securely. For example, the use of fetch()
for making secure HTTP requests, as shown in the code example in the previous section, is a standard practice for interacting with payment gateways in a secure manner.
Furthermore, JavaScript’s asynchronous capabilities allow for non-blocking operations, which is essential for payment processing. The async
and await
syntax, along with promises, enable developers to handle multiple payment processes simultaneously without slowing down the user interface. Here’s a simple example of asynchronous JavaScript code for processing a payment:
async function asyncProcessPayment(details) { try { const response = await fetch('https://api.paymentgateway.com/v1/payments', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify(details), }); const data = await response.json(); console.log('Payment successful:', data); } catch (error) { console.error('Payment failed:', error); } }
Lastly, JavaScript’s large community and wealth of resources mean that developers have access to a vast array of libraries, frameworks, and tools to aid in the development of online payment systems. This community support can be invaluable in resolving issues, finding best practices, and staying up-to-date with the latest security measures.
JavaScript offers a high number of benefits for online payment systems, including ease of integration, improved user experience, customization options, secure data handling, asynchronous processing, and strong community support. These advantages make it a powerful tool for businesses looking to provide their customers with a secure and efficient way to make online payments.
Common Challenges and Solutions in Implementing JavaScript for Online Payments
Implementing JavaScript for online payment systems comes with its own set of challenges. One common issue is dealing with multiple payment gateways and their varying APIs. Each gateway may have different requirements for data formatting, authentication, and error handling. To address this, developers can create a wrapper function that standardizes API calls to different gateways.
function makePaymentGatewayCall(gateway, details) { switch(gateway) { case 'GatewayA': // API call for Gateway A break; case 'GatewayB': // API call for Gateway B break; // Add more cases for other gateways default: throw new Error('Unsupported payment gateway'); } }
Another challenge is managing the security of sensitive information. JavaScript operates on the client-side, which means that any data handled by JavaScript can potentially be exposed to malicious actors. The solution is to use tokenization, where sensitive data is replaced with a non-sensitive equivalent, known as a token, that has no extrinsic or exploitable meaning. Here’s an example:
function tokenizeCard(details) { return fetch('https://api.paymentgateway.com/v1/tokenize', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify(details), }) .then(response => response.json()) .then(data => { return data.token; // Use this token for the payment process }); }
Handling cross-browser compatibility is also a significant challenge. Not all browsers support the latest JavaScript features, so developers must ensure their code is compatible with older versions or use transpilers like Babel to convert contemporary JavaScript into older syntax.
Lastly, handling payment errors gracefully is essential for maintaining a good user experience. JavaScript’s try...catch
blocks can be used to catch errors and display effortless to handle messages instead of raw error data.
async function processPaymentWithGracefulErrorHandling(details) { try { const token = await tokenizeCard(details); // Proceed with payment using the token } catch (error) { console.error('Error during payment process:', error); alert('An error occurred while processing your payment. Please try again.'); } }
By addressing these challenges with standardized API calls, secure handling of sensitive data, cross-browser compatibility considerations, and graceful error handling, developers can create a robust and reliable online payment system using JavaScript.
Best Practices for Secure Online Payment Processing with JavaScript
When it comes to online payment processing, security is paramount. The following best practices will help ensure that your JavaScript code is not only effective but also secure.
- Use HTTPS: Always use HTTPS to encrypt data in transit. This prevents attackers from intercepting sensitive information sent to the server.
- Tokenization: As mentioned before, replace sensitive data with tokens to minimize exposure to potential threats.
- Sanitize Input: Always sanitize user input to protect against XSS attacks. Make sure to escape any characters that could be used in a malicious way.
- Content Security Policy: Implement CSP headers to control the resources that the client is allowed to load for a given page.
- Keep Dependencies Updated: Regularly update all libraries and dependencies to protect against known vulnerabilities.
- Limit Payment Attempts: To prevent brute force attacks, limit the number of payment attempts from a single user or IP address.
- Monitor and Log: Monitor for suspicious activity and maintain logs for all payment transactions to aid in any necessary investigations.
Here’s an example of how you might implement input sanitization in your JavaScript code:
function sanitizeInput(input) { const div = document.createElement('div'); div.textContent = input; return div.innerHTML; } const userInput = sanitizeInput(document.getElementById('payment-input').value);
And here’s how you might set up a CSP header in your JavaScript code:
function setCSP() { const csp = "default-src 'self'; script-src 'self' https://trustedscripts.example.com; object-src 'none';"; document.addEventListener('DOMContentLoaded', function() { const meta = document.createElement('meta'); meta.httpEquiv = "Content-Security-Policy"; meta.content = csp; document.getElementsByTagName('head')[0].appendChild(meta); }); } setCSP();
Note: Always consult with a security expert when implementing payment systems to ensure that all best practices and security measures are properly applied.
Future Trends and Developments in JavaScript for Online Payment Systems
As the online payment landscape continues to evolve, JavaScript developers must stay ahead of the curve to meet the demands of state-of-the-art e-commerce. One of the most exciting future trends is the integration of AI and machine learning algorithms within payment systems. These technologies can be employed to detect and prevent fraud, personalize user experiences, and optimize transaction success rates.
Another area of development is the expansion of cryptocurrency payment options. With the growing popularity of digital currencies, JavaScript developers will need to adapt their systems to support blockchain-based transactions:
async function processCryptoPayment(details) { // Code to interact with a cryptocurrency payment gateway }
Mobile payments are also on the rise, and JavaScript is at the forefront of this trend. Developers can leverage frameworks like React Native to build cross-platform mobile payment applications that provide a seamless experience across devices:
function initiateMobilePayment(details) { // Code to handle mobile payment processes }
Furthermore, the adoption of Web Payments API is set to simplify and standardize online payments. This browser-native API allows users to make payments quickly and easily using their preferred payment methods, all within the browser environment:
if (window.PaymentRequest) { // Initialize a new PaymentRequest object }
Serverless architectures are also gaining traction, reducing the need for server management and maintenance. This approach allows developers to focus on frontend JavaScript code while using cloud services for backend processes:
fetch('https://api.serverless.paymentgateway.com/pay', { method: 'POST', // Additional options and data })
Lastly, the emergence of Progressive Web Apps (PWAs) has implications for online payments. PWAs offer an app-like experience in a web browser, and JavaScript developers can incorporate payment functionalities that work offline or in low-network conditions:
window.addEventListener('load', () => { if (navigator.serviceWorker) { navigator.serviceWorker.register('/service-worker.js').then(() => { // Payment code that works offline }); } });
JavaScript’s role in online payment systems is set to become even more significant. Developers should prepare for these future trends by embracing new technologies, staying informed about industry developments, and continually refining their skills. The future of online payments in JavaScript promises enhanced security, user convenience, and innovative features that will shape the e-commerce experience for years to come.