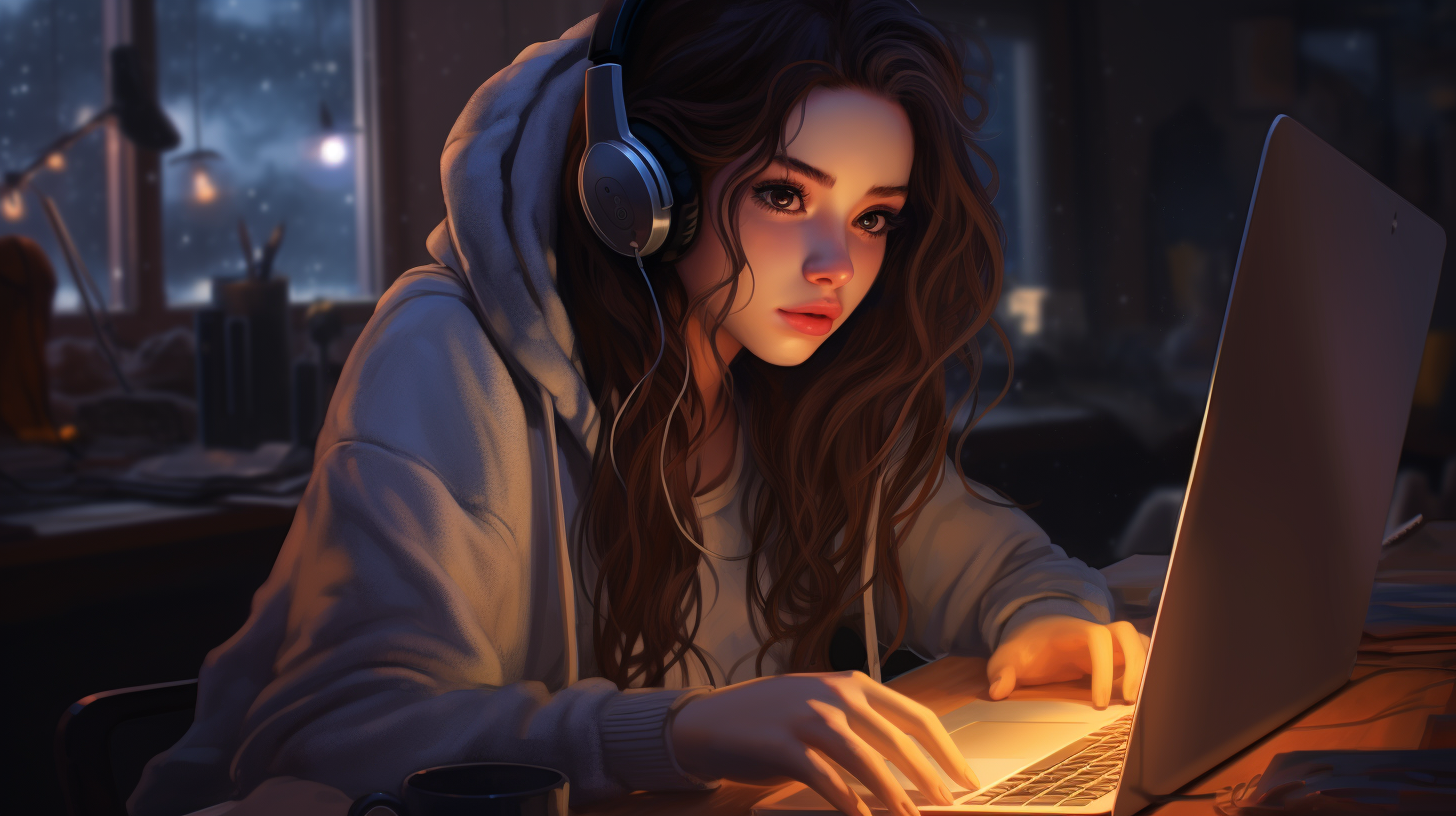
PHP and Web Crawling
Introduction to Web Crawling
Web crawling, also known as web scraping or web harvesting, is the process of automatically navigating through the web, accessing web pages, and extracting useful information from them. That is commonly done by software known as a web crawler, which is designed to follow links and gather data from various sources across the internet.
Web crawlers can be used for a variety of purposes, such as indexing web pages for search engines, archiving websites, or gathering specific data for research or business intelligence. The data collected can range from simple text content, images, and links to more complex data like structured metadata or social media statistics.
The basic workflow of a web crawler involves starting with a list of URLs to visit, known as seeds. The crawler visits each URL, parses the content of the page, and extracts the desired information. It also identifies all the hyperlinks on the page and adds them to the list of URLs to visit next. This process continues recursively, allowing the crawler to navigate through the web, hopping from one page to another.
Web crawlers must be designed to handle various challenges such as different website structures, dynamic content loaded with JavaScript, handling of cookies and sessions, and respecting the rules set by website owners in the robots.txt file. Additionally, web crawlers need to be considerate of the websites they visit by not overwhelming the servers with too many requests in a short period, which is often referred to as polite crawling.
In the context of PHP, a server-side scripting language, developers can create custom web crawlers tailored to their specific needs. PHP offers a range of tools and libraries that facilitate the process of sending HTTP requests, parsing HTML content, and extracting data.
// Example PHP code for a simple web crawler $seed_url = 'http://example.com'; $visited_urls = array(); function crawl_page($url) { global $visited_urls; $visited_urls[] = $url; $html = file_get_contents($url); preg_match_all('/<a href="([^"]+)"/', $html, $matches); foreach ($matches[1] as $new_url) { if (!in_array($new_url, $visited_urls)) { crawl_page($new_url); } } // Extract and process data from $html here } crawl_page($seed_url);
While the above code is a very simplified example, it demonstrates the basic structure of a web crawler. It starts with a seed URL, fetches the HTML content, and uses a regular expression to extract all the hyperlinks on the page. It then recursively visits each new URL, adding them to the list of visited URLs to avoid revisiting the same page.
As we dive deeper into the topic, we will explore the basics of PHP, how to use PHP for web crawling, handling data extraction, and best practices for web crawling with PHP.
Basics of PHP
Before diving into the creation of web crawlers using PHP, it is essential to understand the basics of PHP itself. PHP, which stands for Hypertext Preprocessor, is a widely-used open source scripting language that’s especially suited for web development and can be embedded into HTML.
PHP scripts are executed on the server, and the result is returned to the client as plain HTML. The language is easy to learn for beginners, yet offers many advanced features for professional programmers.
Here’s an example of a basic PHP script:
<?php echo "Hello, World!"; ?>
This script will output “Hello, World!” to the browser. In PHP, all scripts start with <?php
and end with ?>
. The echo
statement is used to output text.
PHP is particularly strong in its ability to interact with databases. It can communicate with different database types, such as MySQL, PostgreSQL, Oracle, and others. Database interactions are essential for web crawling as they allow for the storage and retrieval of crawled data. Here’s an example of connecting to a MySQL database using PHP:
$servername = "localhost"; $username = "username"; $password = "password"; $dbname = "myDB"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected successfully";
Another crucial aspect of PHP is its ability to handle and manipulate strings, which comes in handy when extracting and processing data from web pages. PHP offers a variety of functions for string manipulation, such as str_replace, preg_match, and strpos.
For instance, if you want to search for a specific word within a string and replace it, you could use the following PHP code:
$text = "The quick brown fox jumps over the lazy dog"; $search = "fox"; $replace = "cat"; $newText = str_replace($search, $replace, $text); echo $newText; // Outputs: The quick brown cat jumps over the lazy dog
A solid understanding of PHP’s basics is vital for web crawling. Familiarity with server-side scripting, database interactions, and string manipulation will lay the foundation for building effective web crawlers.
Using PHP for Web Crawling
Using PHP for web crawling is a powerful way to automate the process of gathering data from the web. PHP provides several functions and libraries that can handle HTTP requests, parse HTML content, and extract the information you need. One such library is cURL, which is used to transfer data with URLs. Another popular choice is the DOMDocument class, which allows you to navigate and manipulate the DOM of a webpage.
To start crawling a webpage using PHP, you first need to make an HTTP request to fetch the page content. You can use the file_get_contents() function for simple GET requests, or cURL for more complex scenarios that require handling cookies, redirects, or POST data.
// Fetching a webpage content using file_get_contents $html = file_get_contents('http://example.com'); // Fetching a webpage content using cURL $curl = curl_init('http://example.com'); curl_setopt($curl, CURLOPT_RETURNTRANSFER, true); $response = curl_exec($curl); curl_close($curl);
Once you have the HTML content, you can parse it to extract the data you need. For simple string matching, you can use regular expressions with preg_match() or preg_match_all(). However, for more complex HTML structures, it’s recommended to use the DOMDocument class along with DOMXPath to query the DOM using XPath expressions.
// Loading HTML content into DOMDocument $dom = new DOMDocument(); @$dom->loadHTML($html); // Suppress warnings from malformed HTML // Querying the DOM with XPath $xpath = new DOMXPath($dom); $nodes = $xpath->query("//a[@href]"); // Extracting href attributes from anchor tags foreach ($nodes as $node) { $links[] = $node->getAttribute('href'); }
When you have extracted the links from a page, you can recursively visit each link to crawl additional pages. It’s essential to keep track of the URLs you have already visited to avoid infinite loops and to be considerate of the website’s server by introducing delays between requests, if necessary.
// Function to crawl a webpage and extract links function crawl_page($url, &$visited_urls) { if (in_array($url, $visited_urls)) { return; } $visited_urls[] = $url; $html = file_get_contents($url); // Parse and extract links // ... (Use DOMDocument or regex as shown above) // Crawl each link foreach ($links as $link) { crawl_page($link, $visited_urls); } } // Usage $seed_url = 'http://example.com'; $visited_urls = array(); crawl_page($seed_url, $visited_urls);
Using PHP for web crawling is a flexible and efficient way to automate data collection. By using PHP’s built-in functions and libraries, you can build customized web crawlers that fit your data extraction needs. Just remember to always respect the website’s terms of service and crawl responsibly.
Handling Data Extraction
When it comes to handling data extraction in PHP, there are several techniques and libraries that can be used to efficiently scrape and parse the information from web pages. The most common approach is to use regular expressions or DOM parsing to extract the data.
For example, if we want to extract all the links from a webpage, we can use the preg_match_all()
function with a regular expression that matches HTML anchor tags:
$html = file_get_contents('http://example.com'); preg_match_all('/(.+?)/i', $html, $matches); $links = $matches[1];
However, regular expressions can be complex and fragile, especially when dealing with nested HTML elements. A more robust solution is to use a DOM parser, which allows you to navigate the structure of the HTML document and extract elements based on their tags, attributes, and content.
PHP has a built-in class called DOMDocument
that can be used for this purpose:
$dom = new DOMDocument(); @$dom->loadHTML($html); $xpath = new DOMXPath($dom); $nodes = $xpath->query('//a'); foreach($nodes as $node) { $links[] = $node->getAttribute('href'); }
In the above code, we use the DOMXPath
class to query the document for all anchor tags and then loop through each node to extract the href
attribute.
Another powerful tool for data extraction in PHP is the Simple HTML DOM Parser library. This library provides a simple and consistent interface for working with HTML elements. Here’s how you can use it to extract links:
include('simple_html_dom.php'); $html = file_get_html('http://example.com'); foreach($html->find('a') as $element) { $links[] = $element->href; }
After extracting the data, it’s important to store it in a structured format such as an array or a database. This will make it easier to manipulate and analyze the data later on. For instance, you can store the links in an array and then save them to a CSV file:
$file = fopen('links.csv', 'w'); foreach ($links as $link) { fputcsv($file, array($link)); } fclose($file);
Handling data extraction requires careful consideration of the website’s structure and the type of data you need to extract. By using PHP’s built-in functions, DOM parsing, or external libraries like Simple HTML DOM Parser, you can create efficient and reliable web crawlers that collect valuable data from the web.
Best Practices for Web Crawling with PHP
When it comes to web crawling with PHP, following best practices especially important for both the efficiency of your crawler and the respect of the websites you are visiting. Below are some key best practices to keep in mind:
- Always check the site’s robots.txt file before crawling. This file outlines the areas of the site that the owner does not want crawlers to access. Ignoring these rules can lead to your crawler being blocked or even legal repercussions.
- Identify your crawler with a unique user-agent string. This allows website owners to easily identify your crawler in their logs and set specific rules for it in robots.txt.
- Implement delays between requests to avoid overwhelming the server. This can be done using the
sleep()
function in PHP. - Your crawler should be able to handle HTTP errors like 404 or 503 without crashing. Use proper error handling techniques to manage these scenarios.
- Keep track of URLs that you have already visited to prevent crawling the same page multiple times. This can be managed with an array or a database.
- Use efficient parsing methods to extract data. Libraries like Simple HTML DOM Parser can simplify this process.
Here’s an example of how to implement some of these best practices in PHP:
$seed_url = 'http://example.com'; $visited_urls = array(); $user_agent = 'MyCrawlerBot/1.0'; function crawl_page($url, $user_agent) { global $visited_urls; // Check if URL has already been visited if(in_array($url, $visited_urls)) { return; } // Respect robots.txt if(!is_allowed_to_crawl($url)) { return; } // Set custom user-agent $options = array('http' => array('user_agent' => $user_agent)); $context = stream_context_create($options); // Get HTML content $html = file_get_contents($url, false, $context); // Implement delay sleep(1); // Error handling if($html === FALSE) { // Handle error } else { // Parse and extract data // ... // Add URL to visited list $visited_urls[] = $url; } // Find and crawl all links on the page // ... } function is_allowed_to_crawl($url) { // Check robots.txt rules // ... return true; // Placeholder for actual logic }
By adhering to these best practices, you can ensure that your PHP web crawler is both effective and respectful of the websites it interacts with.