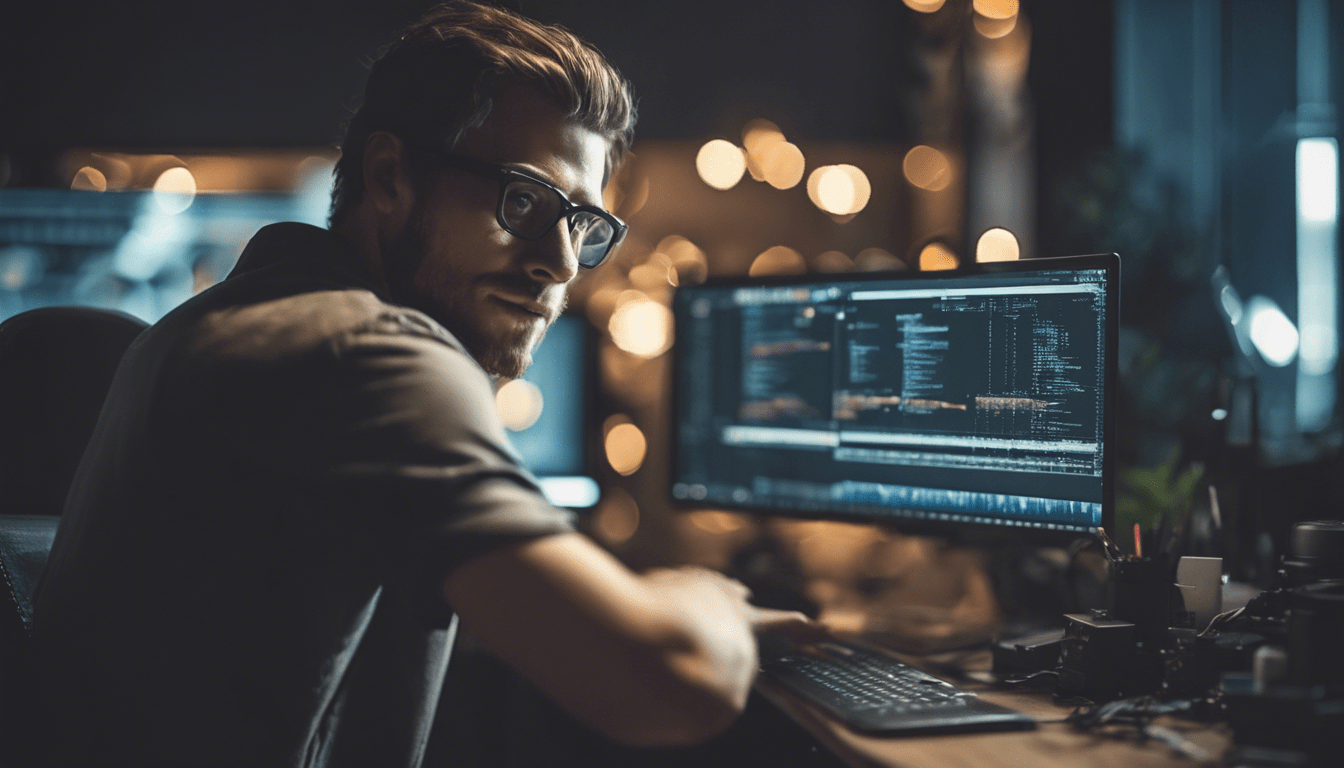
JavaScript for Video Processing and Streaming
Detailed Explanation of Concepts
JavaScript is a versatile programming language that can also be used for video processing and streaming. With the help of the Media API, JavaScript allows developers to manipulate and stream videos directly within web applications. In this article, we will delve into the key concepts related to JavaScript for video processing and streaming, and explain them in simple terms using JavaScript code examples.
1. Video Element
The Video element is an HTML5 element that provides a built-in video player in web browsers. To work with videos, we can use JavaScript to access and manipulate the Video element through its associated DOM methods and properties. Here’s a simple example to programmatically control a video:
let video = document.querySelector('video'); video.play();
2. Video Format and Codec
Videos come in various formats like MP4, WebM, and Ogg. Each format requires a specific codec for encoding and decoding the video data. JavaScript provides support for different video formats through the Video element’s src
attribute. Here’s an example of setting the video source dynamically:
let video = document.querySelector('video'); video.src = 'path/to/video.mp4'; video.play();
3. Video Manipulation
JavaScript allows us to manipulate videos on-the-fly by modifying various attributes and properties of the Video element. For example, we can control the video playback speed, volume, and current time. Here’s how to speed up a video:
let video = document.querySelector('video'); video.playbackRate = 2;
4. Video Streaming
Streaming videos over the web is a common requirement for modern web applications. JavaScript can help achieve this by utilizing streaming protocols like HTTP Live Streaming (HLS) or Dynamic Adaptive Streaming over HTTP (DASH). These protocols break the video into smaller chunks and deliver them to the client progressively. Here’s an example of using HLS:
let video = document.querySelector('video'); let hls = new Hls(); hls.loadSource('path/to/video.m3u8'); hls.attachMedia(video);
Step-by-Step Guide
1. Setting Up the HTML
To start, create an HTML file and add a Video element to it. Give the Video element an ID so that we can easily access it with JavaScript.
2. Selecting the Video Element
In the JavaScript code, use the querySelector()
method to select the Video element and assign it to a variable.
let video = document.querySelector('#myVideo');
3. Controlling Video Playback
After selecting the Video element, use its properties and methods to control playback. For example, to play the video, use the play()
method.
video.play();
4. Updating Video Source
To change the video source dynamically, assign a new URL to the Video element’s src
attribute. Make sure to convert the ‘<' and '>‘ characters to ‘<' and '>‘ within the HTML code.
video.src = 'path/to/newVideo.mp4'; video.load(); video.play();
5. Manipulating Video Properties
JavaScript allows us to control various properties of the Video element, such as playback rate, volume, and current time. Here’s an example of increasing the playback rate:
video.playbackRate = 2;
Common Pitfalls and Troubleshooting Tips
1. Not Including the MIME Type
When specifying the video source using the
element, make sure to include the correct MIME type attribute. This ensures that the browser can understand and handle the video format properly.
2. Incompatible Codecs
Not all browsers support all video codecs. It is essential to choose a video codec this is widely supported across different browsers and platforms. MP4 with H.264 is a reliable choice.
3. CORS Issues
If you are trying to load a video from another domain, ensure that the server includes proper CORS headers to enable cross-origin requests. Otherwise, the video may fail to load or display.
Access-Control-Allow-Origin: *
Further Learning Resources
- “JavaScript: The Definitive Guide” by David Flanagan, “Eloquent JavaScript” by Marijn Haverbeke
- Udemy’s “JavaScript Fundamentals” by Colt Steele, Coursera’s “JavaScript for Beginners” by University of Michigan
- MDN Web Docs’ “Manipulating video using canvas” tutorial, YouTube tutorials by The Net Ninja
JavaScript provides powerful capabilities for video processing and streaming within web applications. By understanding the concepts explained in this article, beginners can start integrating videos into their JavaScript projects and create engaging and interactive user experiences. Remember to practice and experiment with the code examples provided, and continue learning from additional resources to imropve your JavaScript skills. Happy coding!