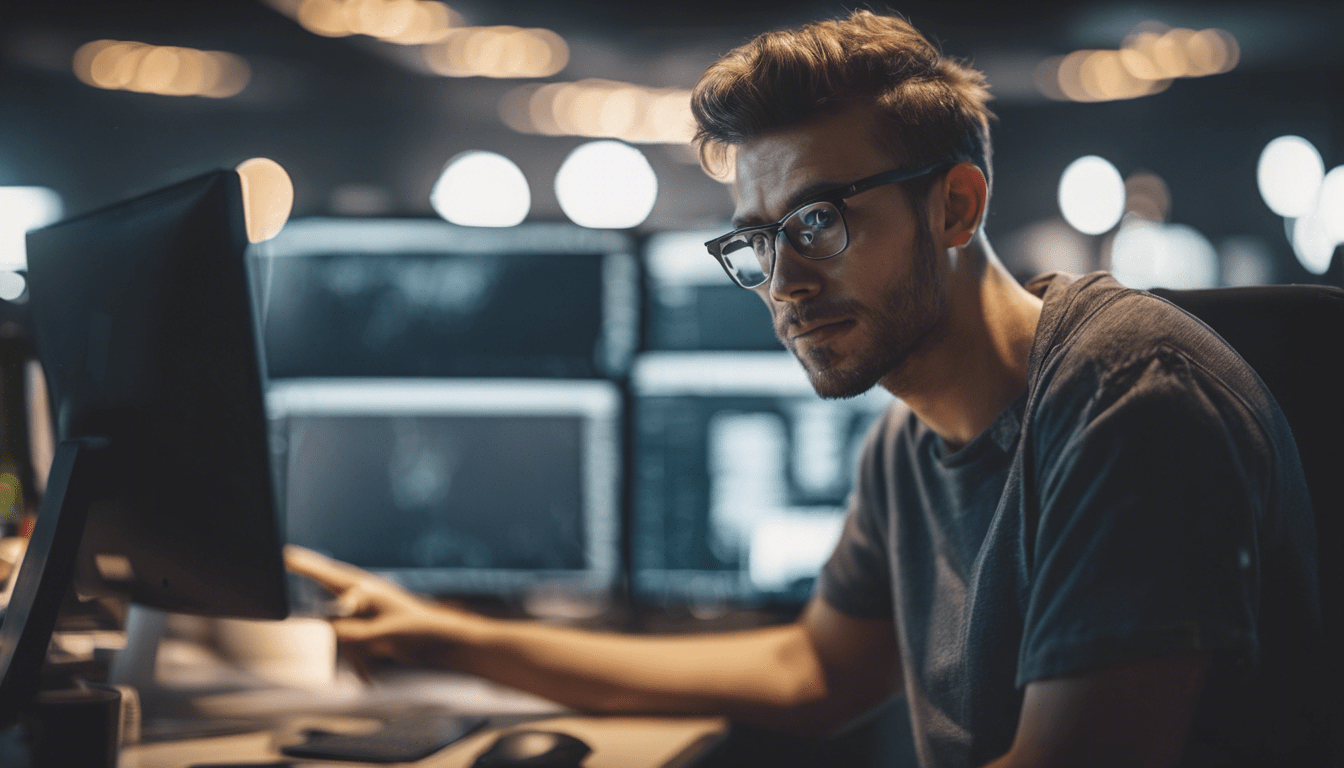
PHP and Cookies: Setting and Retrieving
When it comes to web development, PHP is a popular programming language for creating dynamic websites. One of the essential tasks in web development is setting and retrieving cookies. Cookies are small pieces of data stored on a user’s computer by a website. In this article, we will explore the concepts of setting and retrieving cookies in PHP.
Detailed Explanation of Concepts
Before diving into the code, let’s understand the key concepts related to PHP and cookies.
What are cookies?
Cookies are small files that contain data, often in the form of key-value pairs, which are stored on the user’s computer by a website. These cookies can be accessed and modified by both the server and the client-side scripts.
Setting cookies with setcookie()
In PHP, the setcookie() function is used to set cookies. It takes in several parameters:
- The name of the cookie.
- The value associated with the cookie.
- The expiration time of the cookie (number of seconds since the Unix Epoch). If omitted or set to 0, the cookie will expire at the end of the session.
- The path on the server in which the cookie will be available. If set to ‘/’, the cookie will be available throughout the entire website.
- The domain on which the cookie will be available. If omitted, the cookie will be available on the current domain.
- Specifies whether the cookie should only be sent over secure HTTPS connections. Default is false.
- Specifies whether the cookie should be accessible only through the HTTP protocol. Default is false.
Accessing cookies with $_COOKIE
In PHP, cookies are automatically stored in the $_COOKIE superglobal array. The array contains the name-value pairs of all the cookies set by the current script.
Step-by-Step Guide
Now that we understand the concepts, let’s see how to set and retrieve cookies in PHP using a step-by-step guide.
- Open your favorite text editor and create a new PHP file, let’s call it ‘cookie_example.php’.
<?php setcookie('username', 'Mitch Carter', time() + 3600, '/'); ?>
<?php echo $_COOKIE['username']; ?>
- Save the file and open it in a web browser. You should see the value of the ‘username’ cookie displayed on the screen.
Common Pitfalls and Troubleshooting Tips
Setting and retrieving cookies in PHP can sometimes lead to common pitfalls and issues. Here are some tips to overcome them:
1. Forgetting to call setcookie() before outputting any content
In order to set a cookie, you need to call the setcookie()
function before any content is sent to the browser. Make sure to set cookies at the very beginning of your PHP script.
2. Cookies not being set or retrieved
Ensure that cookies are enabled in the user’s browser. Sometimes, users may have disabled cookies, or their browser settings might prevent the storage and retrieval of cookies.
3. Cookie values not updating
If you’re modifying a cookie value, make sure to refresh the page after making the changes. The updated value will only be available on subsequent page loads.
Further Learning Resources
If you want to dive deeper into PHP and learn more about working with cookies, here are some recommended resources:
- “PHP and MySQL Web Development” by Luke Welling and Laura Thomson, “PHP Solutions: Dynamic Web Design Made Easy” by David Powers.
- Udemy’s “The Complete PHP Course” by Rob Percival, Codecademy’s “Learn PHP”.
- The PHP.net official documentation provides comprehensive guides on cookies.
Understanding how to set and retrieve cookies is essential for any PHP developer. Cookies enable websites to personalize user experiences, remember user preferences, and track user activity. By implementing cookies in your PHP projects, you can enhance the functionality and user-friendliness of your websites. Continuously learning and exploring new concepts in PHP will broaden your skillset and open up possibilities for creating more dynamic and interactive web applications.