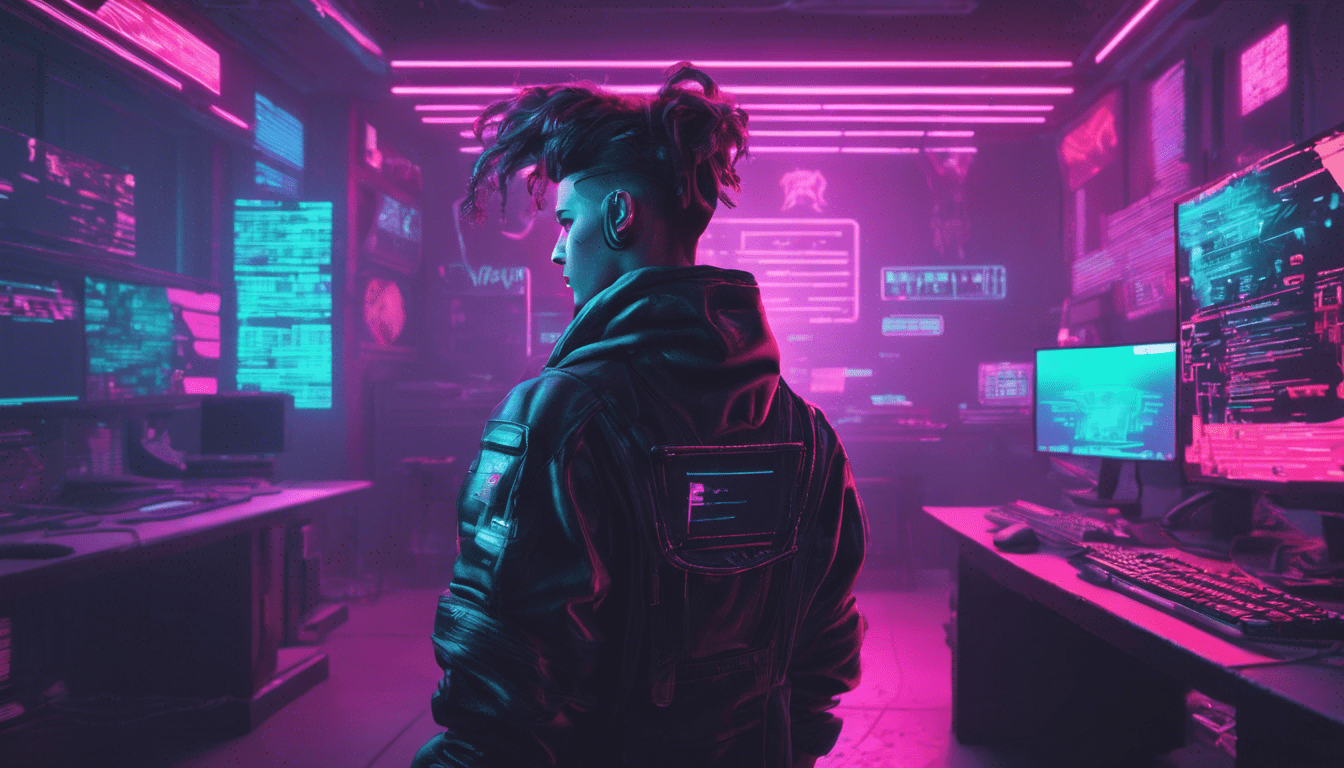
PHP and E-commerce: Building Shopping Carts
If you’re familiar with the internet, you have most likely come across the term e-commerce. E-commerce is simply buying and selling goods or services using the internet, and the transfer of money and data to execute these transactions. A key component of e-commerce is the shopping cart, which is essentially a software that allows online shoppers to select items for purchase, review what they have selected, make necessary modifications or additions and purchase the items.
PHP is a popular server-side scripting language that’s widely used to develop dynamic and interactive websites including e-commerce platforms. One of the reasons PHP is preferred for e-commerce development is its ability to easily integrate with database systems like MySQL, making it easier to manage product listings, inventory, and customer data.
Building a shopping cart using PHP can seem daunting at first, but with a step-by-step approach, it is doable for beginners as well. Let’s take a look at some of the key concepts you need to understand to build a shopping cart in PHP.
1. Sessions:
Sessions are a way to store information across multiple pages. In the context of a shopping cart, sessions are used to store the items a user has selected until they are ready to checkout. When a user selects an item, it is added to their session, and when they visit another page or refresh the page, the selected items remain in their cart.
<?php // Start a session session_start(); // Add an item to the cart $_SESSION['cart'][] = 'Product 1'; // Display the cart print_r($_SESSION['cart']); ?>
2. POST/GET requests:
To add items to a shopping cart, you’ll use forms with POST or GET methods to submit data. POST requests are used when you want to send data securely and without exposing it in the URL, while GET requests are used when you want to retrieve data using query strings in the URL.
<form action="add_to_cart.php" method="post"> <input type="hidden" name="product_id" value="1"> <input type="submit" value="Add to Cart"> </form>
3. Processing form data:
When a form is submitted with product details, you need to process this data and add it to the shopping cart. That’s done by capturing data from the POST request and updating the session accordingly.
<?php // Start a session session_start(); // Check if there's a POST request if($_SERVER['REQUEST_METHOD'] == 'POST') { // Add this product ID to the cart $_SESSION['cart'][] = $_POST['product_id']; } header('Location: view_cart.php'); exit(); ?>
4. Displaying the cart:
You also need a way to display items that are in the shopping cart. This can be done by iterating over the items stored in the session and presenting them in a table or list format.
<?php session_start(); if (!empty($_SESSION['cart'])) { echo '<ul>'; foreach ($_SESSION['cart'] as $item) { echo '<li>' . htmlspecialchars($item) . '</li>'; } echo '</ul>'; } ?>
5. Integrating payment gateways:
Once your shopping cart is functioning correctly, you’ll need to integrate a payment gateway like PayPal. This involves working with APIs provided by these payment gateways to handle payment processing securely.
To wrap it up, building a shopping cart for an e-commerce site in PHP requires understanding sessions, handling POST/GET requests, processing form data, displaying the cart items and integrating payment gateways. It may seem complex at first, but by breaking it down into smaller steps and using PHP’s built-in functions, it’s a task that can be accomplished even by beginners.