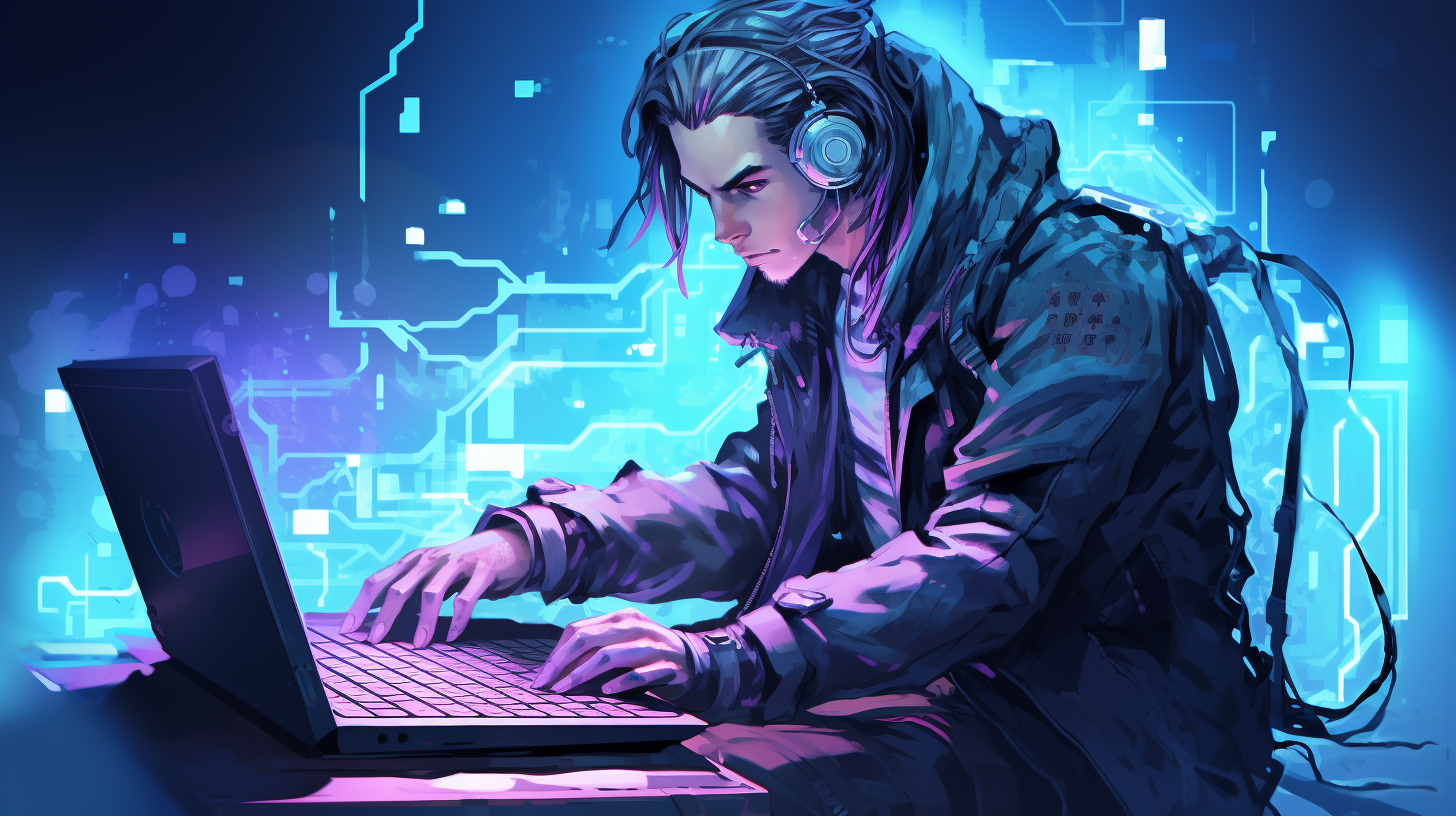
PHP and Graphics: Generating Images
Introduction to PHP Graphics
PHP, a server-side scripting language, is not just limited to creating web pages. It also offers powerful features for generating and manipulating graphics. PHP’s built-in GD library allows developers to create images on-the-fly, and manipulate existing images in various formats such as JPEG, PNG, and GIF.
The GD library provides a range of functions for drawing lines, shapes, and text, as well as applying filters and effects to images. This opens up a world of possibilities for dynamic image creation, from generating simple graphics like charts and graphs to creating complex thumbnails and photo galleries.
To start working with PHP graphics, you need to make sure that the GD library is installed and enabled on your server. You can check this by running the phpinfo()
function and looking for the GD section. If it is not installed, you can enable it by uncommenting or adding the line extension=gd
in your php.ini
file.
<?php phpinfo(); ?>
Once you have confirmed that GD library is enabled, you can start creating images. The basic steps involved in generating an image using PHP are as follows:
- Create a blank image canvas
- Set the colors you want to use
- Draw your shapes or text
- Output the image to the browser or save it to a file
Here’s an example of creating a simple red rectangle:
<?php // Create a 100*100 image $im = imagecreatetruecolor(100, 100); // Create some colors $white = imagecolorallocate($im, 255, 255, 255); $red = imagecolorallocate($im, 255, 0, 0); // Draw a red rectangle imagefilledrectangle($im, 0, 0, 99, 99, $red); // Output the image to browser header('Content-Type: image/png'); imagepng($im); imagedestroy($im); ?>
This code will generate a simple red square and display it in the browser. With the basics covered, we can move on to more advanced techniques such as incorporating text and fonts, applying filters, and optimizing performance.
Basic Image Generation with PHP
Now that we have a basic understanding of PHP’s capabilities in generating graphics, let’s dive deeper into the process of creating images from scratch. The GD library functions offer a set of tools that let us start with an empty canvas and then add colors, shapes, and text to it.
To create an image, you first need to define the dimensions of your canvas using imagecreatetruecolor()
for true color images or imagecreate()
for indexed color images. True color images allow for a broader range of colors, which is ideal for photographs and complex images.
<?php // Create a true color image with width 200 and height 100 $image = imagecreatetruecolor(200, 100); ?>
Once you have your canvas, the next step is to set up the colors you’ll be using. Colors in the GD library are represented by a resource identifier created with imagecolorallocate()
. This function takes in the image resource and the RGB values of the color you want to allocate.
<?php // Allocate a color for the image $black = imagecolorallocate($image, 0, 0, 0); $green = imagecolorallocate($image, 0, 255, 0); ?>
With your colors ready, you can start drawing on the canvas. The GD library provides functions like imagefilledrectangle()
, imageellipse()
, and imagearc()
to draw various shapes. For example, to draw a filled green ellipse:
<?php // Draw a filled green ellipse imagefilledellipse($image, 100, 50, 150, 80, $green); ?>
After creating your masterpiece, you’ll want to display it or save it. To output an image directly to the browser, you need to send the appropriate header followed by an image output function such as imagepng()
or imagejpeg()
. To save the file instead, just pass a filename to the output function.
<?php // Output the image to browser header('Content-Type: image/png'); imagepng($image); // Or save it as a file // imagepng($image, 'myimage.png'); // Free up memory imagedestroy($image); ?>
Remember to call imagedestroy()
after you’re done with an image. This function releases any memory associated with the image resource.
In conclusion, generating basic images with PHP is straightforward once you familiarize yourself with the GD library functions. With these tools at your disposal, you can dynamically create graphics tailored to your web application’s needs.
Advanced Techniques for Image Manipulation
As we delve into the more advanced techniques for image manipulation using PHP, we’ll explore how to apply filters, rotate images, and merge multiple images into one. These techniques can enhance the functionality and aesthetics of your graphics, making them more dynamic and engaging.
Applying Filters: PHP’s GD library includes a variety of filters that can be applied to images. These filters can adjust brightness, contrast, colorize, and more. To apply a filter, use the imagefilter()
function along with the desired filter constant.
// Load an existing image $image = imagecreatefromjpeg('photo.jpg'); // Apply a grayscale filter imagefilter($image, IMG_FILTER_GRAYSCALE); // Save the modified image imagejpeg($image, 'photo_grayscale.jpg'); imagedestroy($image);
Rotating Images: Rotating an image is another useful technique in image manipulation. The imagerotate()
function enables you to rotate an image by a given angle. The function also requires a background color for the uncovered zone after the rotation.
// Load an existing image $image = imagecreatefromjpeg('photo.jpg'); // Set the background color $bgColor = imagecolorallocatealpha($image, 255, 255, 255, 127); // Rotate the image by 45 degrees $rotatedImage = imagerotate($image, 45, $bgColor); // Save the rotated image imagejpeg($rotatedImage, 'photo_rotated.jpg'); imagedestroy($image); imagedestroy($rotatedImage);
Merging Images: Combining two or more images into one can be achieved using the imagecopy()
or imagecopymerge()
functions. These functions are useful for creating watermarks or layering images on top of each other.
// Load the main image $mainImage = imagecreatefromjpeg('photo.jpg'); // Load the watermark image $watermark = imagecreatefrompng('watermark.png'); // Get the width and height of the watermark image $watermarkWidth = imagesx($watermark); $watermarkHeight = imagesy($watermark); // Merge the watermark onto the main image at the bottom-right corner imagecopy($mainImage, $watermark, imagesx($mainImage) - $watermarkWidth, imagesy($mainImage) - $watermarkHeight, 0, 0, $watermarkWidth, $watermarkHeight); // Save the merged image imagejpeg($mainImage, 'photo_with_watermark.jpg'); imagedestroy($mainImage); imagedestroy($watermark);
These advanced techniques allow for more sophisticated manipulations of graphics in PHP. By mastering these methods, you can create visually appealing images with complex effects and layers that enhance your web applications.
Incorporating Text and Fonts in PHP Images
Adding text to images in PHP is a common requirement for many web applications, whether it’s for creating dynamic banners, watermarked photos, or personalized graphics. The GD library in PHP offers functions that make incorporating text into your images straightforward. These functions allow you to specify the font, size, color, and placement of the text on the image.
To add text to an image, you can use the imagestring()
function for basic font support or imagettftext()
for TrueType fonts. The imagestring()
function is simpler but offers less control over the appearance of the text, while imagettftext()
requires you to have the .ttf font file available on your server but gives you the ability to use a wide range of fonts.
Here’s how you can add text to an image using imagestring()
:
// Create a 200*100 image $image = imagecreatetruecolor(200, 100); // Allocate colors $white = imagecolorallocate($image, 255, 255, 255); $black = imagecolorallocate($image, 0, 0, 0); // Fill the background with white color imagefilledrectangle($image, 0, 0, 199, 99, $white); // Add some text to the image imagestring($image, 5, 10, 10, 'Hello World!', $black); // Output the image to browser header('Content-Type: image/png'); imagepng($image); imagedestroy($image);
For more advanced text rendering using TrueType fonts, you can use imagettftext()
. This function enables you to specify the path to the .ttf font file and gives you greater control over font size, angle, and placement. Here’s an example:
// Create a 200*100 image $image = imagecreatetruecolor(200, 100); // Allocate colors $white = imagecolorallocate($image, 255, 255, 255); $black = imagecolorallocate($image, 0, 0, 0); // Fill the background with white color imagefilledrectangle($image, 0, 0, 199, 99, $white); // Set the path to the TrueType font you want to use $fontPath = 'path/to/your/font.ttf'; // Add some text to the image using a TrueType font imagettftext($image, 20, 0, 10, 50, $black, $fontPath, 'Hello World!'); // Output the image to browser header('Content-Type: image/png'); imagepng($image); imagedestroy($image);
When using imagettftext()
, ensure that the font file path is correct and that you have permission to read the font file. Also note that different fonts may render differently at various sizes and angles, so you may need to experiment to get the desired result.
Incorporating text into your PHP images can significantly enhance their functionality and allure. Whether you choose basic or TrueType fonts, PHP’s GD library makes it easy to integrate text into your graphics with just a few lines of code.
Optimizing Performance and Best Practices
When working with PHP and graphics, it’s essential to think performance and follow best practices to ensure efficient image generation and manipulation. Here are some tips to optimize performance and maintain best practices:
- If you’re generating multiple images in a loop or function, reuse the image canvas and colors whenever possible to save memory and processing time.
// Reusing the image canvas $image = imagecreatetruecolor(100, 100); $white = imagecolorallocate($image, 255, 255, 255); for ($i = 0; $i < 10; $i++) { // Draw something on the image // ... // Output or save the image // ... // Clean up the drawing but keep the canvas imagefilledrectangle($image, 0, 0, 99, 99, $white); } imagedestroy($image);
// Saving a JPEG with compression imagejpeg($image, 'image.jpg', 75); // 75 is the quality setting
// Error handling when loading an image $image = @imagecreatefromjpeg('photo.jpg'); if (!$image) { die('Error: Unable to open image.'); }
By following these best practices and focusing on optimization, you can efficiently utilize PHP's capabilities for generating and manipulating graphics without compromising on performance.