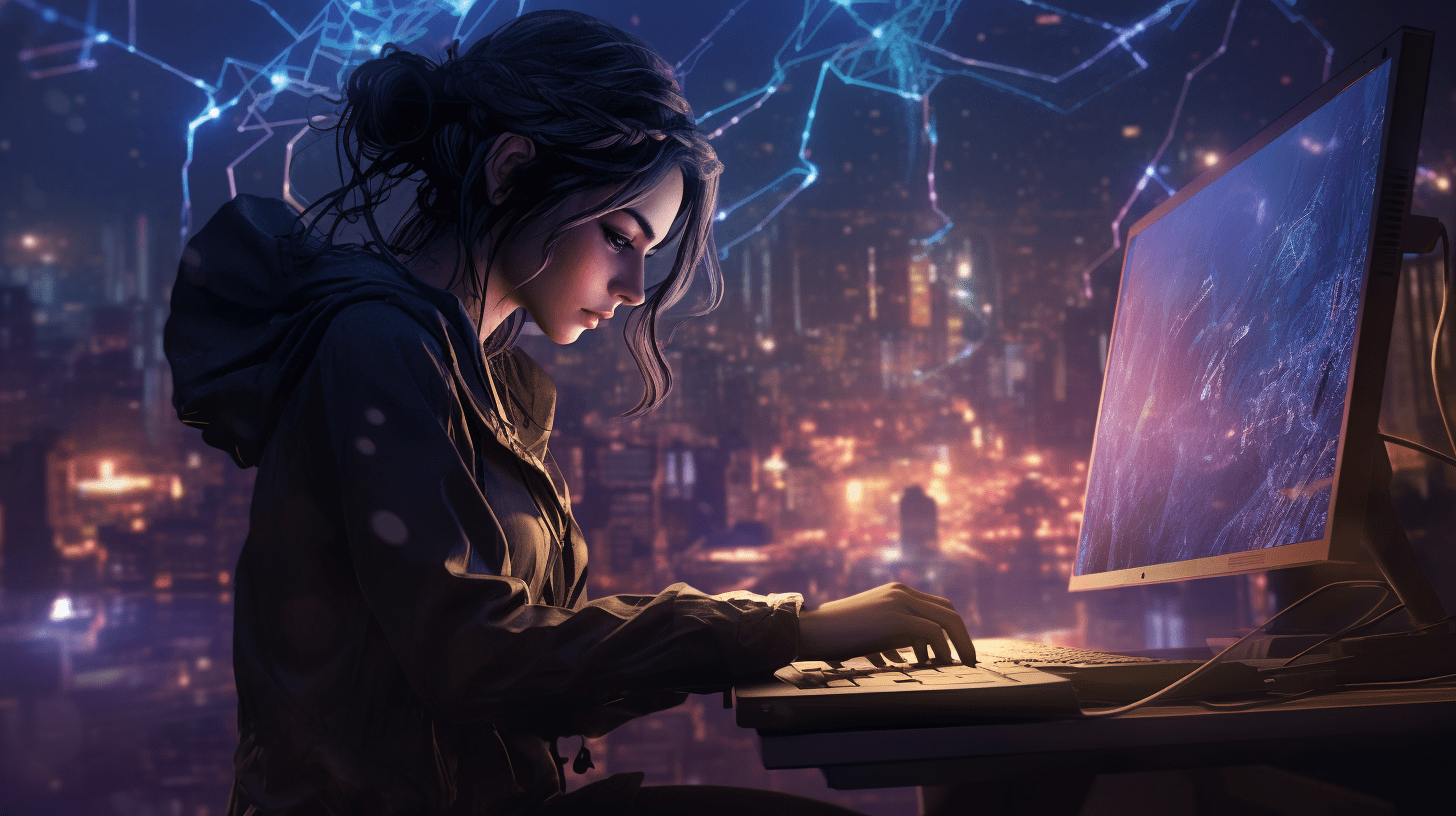
PHP and Microservices Architecture
PHP and Microservices Architecture is a growing trend within the software development industry. Microservices are a design approach to building a single application as a suite of small, independent services. Each of these services runs its process and communicates via lightweight APIs. This architectural style has gained popularity over the monolithic approach because it offers increased agility, scalability, and faster deployment times.
When building microservices with PHP, developers need to focus on creating small, standalone modules that each handle a specific part of the application’s functionality. These services should be loosely coupled and can be developed, deployed, and scaled independently.
Let’s dive into some of the core concepts of using PHP in a microservices architecture:
- This is the underlying principle behind microservices. Each service is designed to handle a specific business capability and should be able to work independently of other services.
- Microservices need to communicate with each other to form a fully functioning application. This can be achieved using RESTful APIs or message brokers like RabbitMQ.
- Each microservice should have its separate database to ensure loose coupling and individual scalability.
- To maximize scalability, each service should be stateless and any state should be managed by the client or a shared caching layer.
Now let’s look at a simple code example of a PHP microservice:
Note: This example assumes you have a working knowledge of PHP and you’ve set up your development environment accordingly.
<?php // Service to handle user registration // Include necessary files include 'User.php'; include 'ResponseHandler.php'; // Get user data from POST request $userData = json_decode(file_get_contents('php://input'), true); // Instantiate User object $user = new User($userData); // Attempt to register user $registrationStatus = $user->register(); // Prepare response $responseHandler = new ResponseHandler(); $response = $responseHandler->generateResponse($registrationStatus); // Send JSON response back to client header('Content-Type: application/json'); echo json_encode($response);
In the code above, we have a microservice that handles user registration. It includes files for the User class, which contains the registration logic, and the ResponseHandler class to format the response. The service receives user data via a POST request, attempts to register the user, and then sends back a JSON response.
When you’re building microservices with PHP, here are some best practices you should follow:
- Keep services small and focused on a single responsibility.
- Adopt Continuous Integration/Continuous Deployment (CI/CD) for testing and deploying services independently.
- Use API gateways to manage requests between various client types and microservices.
- Implement proper logging and monitoring for troubleshooting and performance optimizations.
- Think using frameworks like Lumen or Slim to create lightweight microservices.
In conclusion, adopting the microservices architecture in PHP applications can significantly improve flexibility and scalability. By breaking down the application into smaller independent services, PHP developers can benefit from easier maintainability, quicker deployments, and more robust system architecture. With APIs acting as the glue between these services, PHP applications can evolve more naturally over time while adding or updating features with minimal impact on other services.