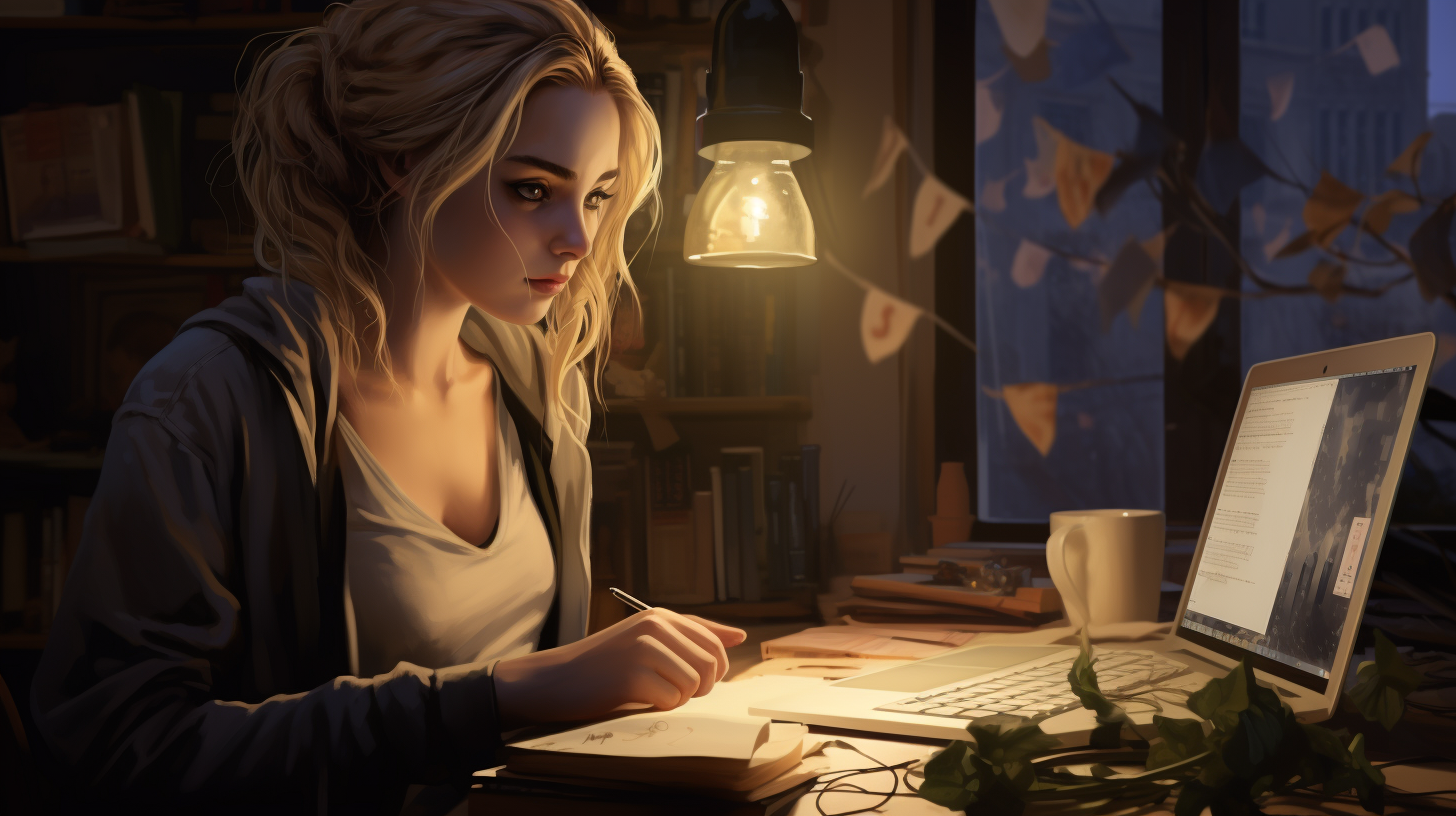
SQL for Data Validation and Quality Assurance
Data validation and quality assurance are critical components of any data management process. SQL, or Structured Query Language, is a powerful tool for ensuring that the data in your database is accurate, consistent, and reliable. In this article, we will explore some common SQL techniques for data validation and quality assurance.
One of the most fundamental aspects of data validation is ensuring that data is in the correct format. For example, if you have a column that is supposed to contain dates, you want to make sure that all the values in that column are valid dates. You can use the CAST and CONVERT functions in SQL to check the data type of a column and convert it if necessary.
SELECT column_name, CASE WHEN ISDATE(column_name) = 1 THEN 'Valid Date' ELSE 'Invalid Date' END AS Date_Validation FROM table_name;
Another important aspect of data validation is checking for missing or null values. Null values can cause issues when trying to perform calculations or generate reports. You can use the IS NULL or IS NOT NULL operators in SQL to identify and handle null values.
SELECT * FROM table_name WHERE column_name IS NULL;
Data quality assurance also involves ensuring that the data is consistent and free of duplicates. You can use the DISTINCT keyword in SQL to remove duplicate rows from your results.
SELECT DISTINCT column_name FROM table_name;
In addition to removing duplicates, you can also use SQL to identify and merge duplicate records. For example, if you have two records for the same customer with slightly different spellings of their name, you can use SQL to combine the records and update the data.
UPDATE table_name SET column_name = 'Correct Spelling' WHERE column_name = 'Incorrect Spelling';
Finally, data validation and quality assurance often involve setting constraints on the data. For example, you may want to ensure that all values in a column are within a certain range or meet a specific condition. You can use the CHECK constraint in SQL to enforce these rules.
ALTER TABLE table_name ADD CONSTRAINT constraint_name CHECK (column_name BETWEEN 1 AND 100);
To wrap it up, SQL provides a variety of tools and techniques for data validation and quality assurance. By using functions such as CAST, CONVERT, ISDATE, IS NULL, DISTINCT, and CHECK, you can ensure that your data is accurate, consistent, and reliable.