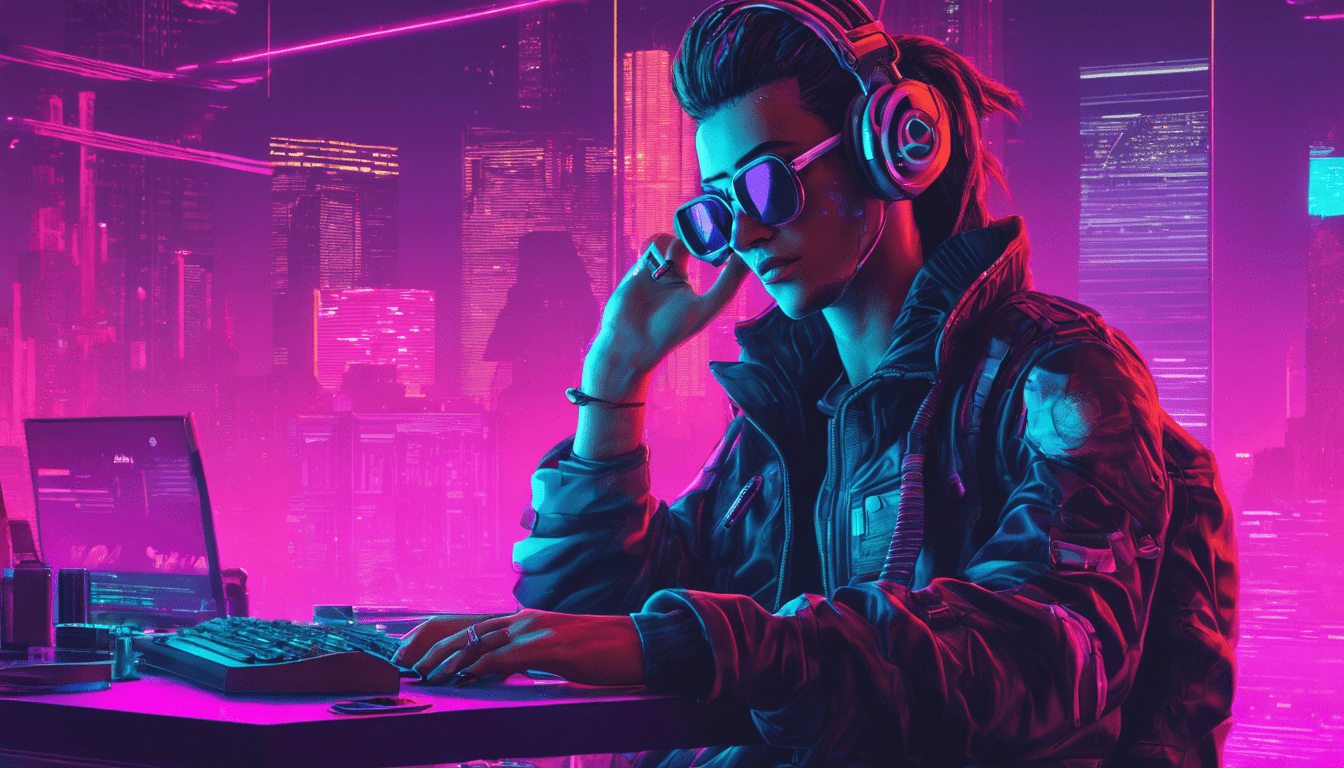
PHP Best Coding Practices
PHP is a widely-used open-source scripting language that’s especially suited for web development. If you are a beginner to PHP development, you are likely eager to dive in and start writing your first lines of code. However, it is important to take some time to understand the best coding practices for PHP. Following these practices will result in clean, efficient, and maintainable code this is easy to read and debug.
Coding Standards
One of the basic principles of good PHP coding practices is adherence to established PHP coding standards. The most widely accepted standard in the PHP community is the PHP Framework Interop Group’s PSR (PHP Standard Recommendation). The PSRs cover various aspects such as basic coding standards (PSR-1), coding style guide (PSR-2), logger interface (PSR-3), etc.
For beginners, it’s essential to understand PSR-1 and PSR-2 standards as they deal with basic coding and style guides. These standards help in maintaining uniformity and consistency in the code, which enhances readability and collaboration.
Here’s an example of how to use PSR-1 and PSR-2 standards:
<?php namespace VendorPackage; class ClassName { public function functionName($arg1, $arg2 = '') { // code here } } ?>
Readability
In addition to following standards, readability is another important aspect of good PHP coding practices. The goal of making your code readable is to ensure that other developers can understand your code. There are several ways to imropve readability:
- Comments: Use comments to explain complex code blocks. However, avoid over-commenting and try to write code that’s self-explanatory.
- Indentation: Consistent indentation makes your code easier to read and understand. The PSR-2 recommends using 4 spaces for each indent.
- Variable names: Use meaningful variable names that reflect what the variable is used for.
- White space: Use white space between operators, after commas in arrays and between control structures like if, while, for.
Here’s an example of readable PHP code:
<?php // Calculating the area of a rectangle $length = 10; $width = 20; $area = $length * $width; echo "The area of the rectangle is " . $area . " square units."; ?>
In this example, the variable names are meaningful, there’s appropriate white space, and a comment is used to explain the purpose of the code.
In conclusion, adhering to coding standards such as PSR and focusing on readability can significantly improve your PHP coding practices. As a beginner, incorporating these habits early on will help you write better, more efficient PHP code.