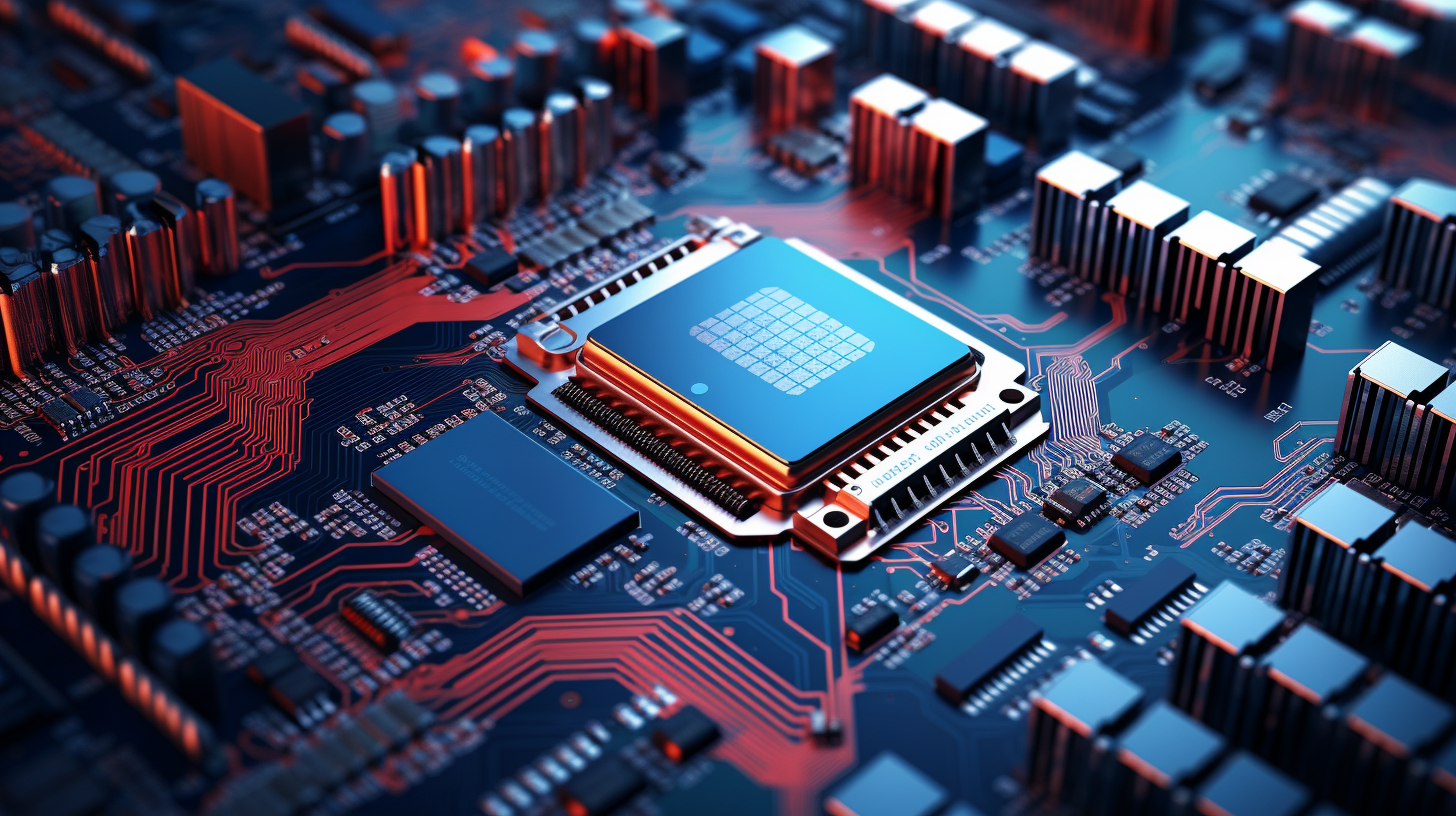
Python and APIs: Interacting with Web Services
Python is a versatile programming language that can be used to interact with web services through APIs (Application Programming Interfaces). APIs allow different software applications to communicate and interact with each other, enabling the exchange of data and functionality.
In this tutorial, we will explore how to use Python to interact with web services using APIs. Specifically, we will focus on the concept of RESTful APIs, which are widely used in web development. REST stands for Representational State Transfer and is an architectural style for designing networked applications.
Prerequisites
- A basic understanding of Python programming language.
- An internet connection to access web services.
- A text editor or integrated development environment (IDE) for writing Python code.
pip install requests
Understanding APIs and Web Services
An API is a set of rules and protocols that allows different software applications to communicate with each other. It defines the structure and format of requests and responses between the client (the application making the request) and the server (the application processing the request).
A web service is a type of API that operates over the internet, allowing software applications to interact and exchange data. Web services are built on top of existing internet protocols like HTTP, and they allow applications to communicate regardless of the programming languages or platforms they’re built on.
Interacting with Web Services using Python
Python provides several libraries and modules that make it easy to interact with web services using APIs. One of the most commonly used libraries for this purpose is requests
.
The requests
library allows us to send HTTP requests to web services and handle their responses. It provides an intuitive and effortless to handle interface, making it convenient for developers to work with APIs.
Let’s start by making a simple GET request to a web service using the requests
library:
import requests response = requests.get('https://api.example.com/data') print(response.status_code) # Prints the status code of the response print(response.json()) # Prints the response content in JSON format
In the example above, we imported the requests
library and made a GET request to the URL ‘https://api.example.com/data’. We stored the response in a variable called response
.
We then printed the status code of the response using response.status_code
and the content of the response in JSON format using response.json()
.
The status code indicates the success or failure of the request. For example, a status code of 200 means the request was successful, while a status code of 404 means the requested resource was not found.
The response content can be accessed and manipulated using various methods and attributes provided by the requests
library. For example, you can access the response headers using response.headers
, retrieve specific data from the response using response.json()
, or parse HTML content using third-party libraries like BeautifulSoup
.
Sending Data with HTTP Methods
HTTP methods like GET, POST, PUT, and DELETE are commonly used when interacting with web services. By default, the requests
library uses the GET method, but we can easily send data using other methods as well.
For example, to send data as JSON in a POST request:
import requests data = { 'name': 'Mitch Carter', 'email': '[email protected]' } response = requests.post('https://api.example.com/users', json=data) print(response.status_code) # Prints the status code of the response
In the example above, we created a dictionary called data
containing the name and email of a user. We then made a POST request to the URL ‘https://api.example.com/users’ and passed the data
dictionary as JSON using the json
parameter.
We can also send data as form-urlencoded or multipart form data using the data
parameter instead of json
.
Adding Headers and Authentication
The requests
library allows us to add custom headers to our HTTP requests. Headers can be used to pass additional information or authentication credentials to the web service.
Here’s an example that adds a custom header to a request:
import requests headers = { 'Authorization': 'Bearer xyz123' } response = requests.get('https://api.example.com/data', headers=headers) print(response.status_code) # Prints the status code of the response
In the example above, we created a dictionary called headers
that contains the authorization token as a Bearer token. We then made a GET request to the URL ‘https://api.example.com/data’ and passed the headers
dictionary using the headers
parameter.
In addition to custom headers, the requests
library provides built-in support for different authentication methods like Basic Authentication, OAuth, and JWT (JSON Web Token).
Error Handling and Exception Handling
When interacting with web services, it’s important to handle errors and exceptions gracefully. The requests
library raises various exceptions for different types of errors, such as invalid URLs, connection errors, or HTTP errors.
Here’s an example that demonstrates how to handle different types of exceptions:
import requests try: response = requests.get('https://api.example.com/data') response.raise_for_status() # Raise an exception if the status code is not 2xx except requests.exceptions.RequestException as e: print('An error occurred:', e)
In the example above, we used a try-except
block to handle exceptions. If an exception occurs during the execution of the try
block, it’s caught and the except
block is executed.
We used the raise_for_status()
method to raise an exception if the status code of the response is not within the 2xx range (indicating a successful request).
By handling exceptions, we can gracefully handle errors and provide meaningful feedback to the user.
In this tutorial, we explored how to use Python to interact with web services using APIs. We learned about the idea of RESTful APIs, made GET and POST requests using the requests
library, added custom headers and authentication, and handled errors and exceptions.
Python provides a powerful and easy-to-use toolset for interacting with web services, allowing developers to integrate their applications with a wide range of APIs and leverage the functionality and data they provide.
By mastering the art of working with APIs, you can unlock endless possibilities for your Python applications and create powerful and seamless integrations with other software systems.