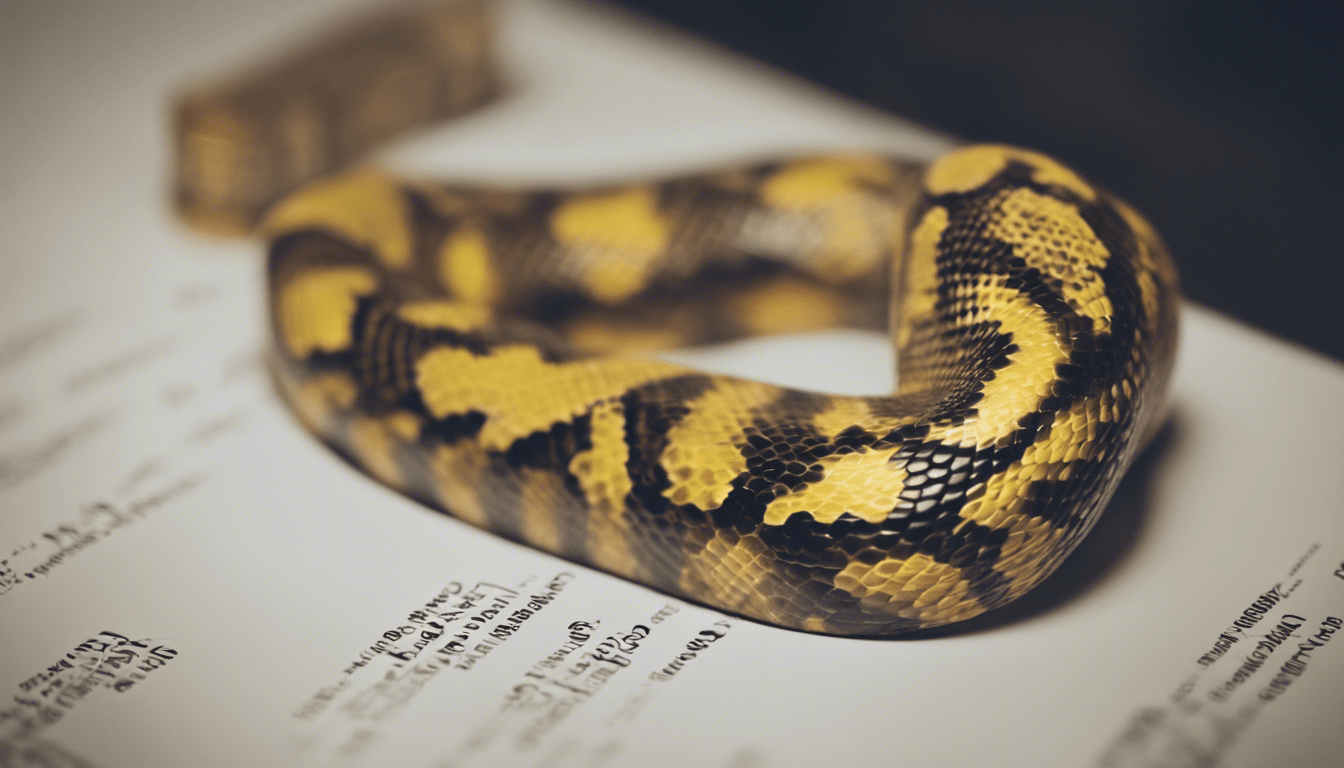
Python and Django: Developing Web Applications
Python has gained immense popularity in various domains, including web development. When it comes to building web applications, Python offers numerous frameworks that simplify the development process. One such framework is Django, which follows the Model-View-Controller (MVC) architectural pattern.
Detailed Explanation of Concepts
1. Python: Python is a high-level programming language that emphasizes code readability and simplicity. Its clean syntax makes it an excellent language choice for beginner developers. Python provides a wide range of libraries and frameworks, making it suitable for different applications, including web development.
# Python Hello World print("Hello, World!")
2. Django: Django is a powerful web framework written in Python. It simplifies the web development process by providing a standardized structure and set of tools. Django follows the Model-View-Controller (MVC) architectural pattern, where the model represents the data, the view displays the user interface, and the controller handles user input and logic.
3. Model-View-Controller (MVC): MVC is an architectural pattern widely used in web development. The model represents the data and business logic, the view handles the user interface, and the controller manages user input and updates the model and view accordingly. MVC promotes separation of concerns, making applications easier to develop, test, and maintain.
Step-by-Step Guide
Let’s dive into building a simple web application using Python and Django. Follow these steps to get started:
- Install Django: Begin by installing Django on your machine. Open the command prompt or terminal and run the following command:
pip install django
- Create a Django Project: Using the command prompt or terminal, navigate to the desired directory and execute the following command:
django-admin startproject myproject
This will create a new Django project named “myproject”.
- Create a Django App: Change directory to “myproject” and execute the following command to create a new Django app:
python manage.py startapp myapp
This will create a new Django app named “myapp” within the “myproject” project.
- Define Models: Open the “models.py” file inside the “myapp” directory. Define your data models using Python classes. For example, to create a simple blog model, you could use:
from django.db import models class Blog(models.Model): title = models.CharField(max_length=100) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True)
- Create Database Tables: Once you have defined your models, run the following command to create the corresponding database tables:
python manage.py makemigrations python manage.py migrate
- Create Views: In the “views.py” file inside the “myapp” directory, define your views. Views handle user requests and return appropriate responses. For example, to create a view for displaying a blog, you could use:
from django.shortcuts import render from .models import Blog def blog_detail(request, blog_id): blog = Blog.objects.get(id=blog_id) return render(request, 'blog_detail.html', {'blog': blog})
- Create URLs: Open the “urls.py” file in the “myproject” directory and define the URL patterns for your web application. For example, to map the URL “/blog/1” to the “blog_detail” view, you could use:
from django.urls import path from myapp.views import blog_detail urlpatterns = [ path('blog/', blog_detail, name='blog_detail'), ]
- Run the Development Server: Finally, navigate to the “myproject” directory in the command prompt or terminal and run the following command to start the Django development server:
python manage.py runserver
Your web application should now be accessible at “http://localhost:8000/blog/1”.
Common Pitfalls and Troubleshooting Tips
As a newbie, you may encounter some challenges while developing web applications with Python and Django. Here are a few common pitfalls and troubleshooting tips to help you out:
- Double-check your import statements to ensure you’re importing the necessary modules and functions correctly.
- Pay attention to proper indentation as Python relies on it for correct execution. Inconsistent indentation can lead to syntax errors.
- Validate user input to prevent malicious attacks like SQL injection. Django provides built-in mechanisms for data validation and sanitization.
- If you encounter database-related issues, make sure your database settings in the Django configuration file are correctly configured.
Further Learning Resources
To continue your journey in web development with Python and Django, here are some recommended resources for further learning:
- Visit the official Django documentation at https://docs.djangoproject.com/ to explore in-depth topics and advanced concepts.
- This book provides a beginner-friendly introduction to Django and covers essential topics in building web applications.
- Real Python (https://realpython.com/) offers a wide range of tutorials, articles, and courses on Python and Django, catering to all skill levels.
- A high number of online tutorials, such as the Django Project tutorials (https://tutorial.djangoproject.com/), offer step-by-step guides on building different types of Django web applications.
Python and Django provide a powerful combination for developing web applications. Understanding these concepts is important for beginners who aspire to become skilled web developers. By following the step-by-step guide and exploring further resources, you can expand your knowledge and create sophisticated web applications with ease. Remember to practice regularly and keep up with the latest updates in the Python and Django ecosystem for continuous growth in your programming skills.
Great overview of Python and Django for web development! One additional point worth mentioning is the importance of understanding the various databases that can be integrated with Django. While the article touches on creating database tables, beginners should be aware that Django supports several databases such as SQLite, PostgreSQL, MySQL, and Oracle. Choosing the right database for your project’s needs and knowing how to properly configure it within Django very important for the application’s performance and scalability. It would be helpful for beginners to explore the pros and cons of each database option and learn about Django’s ORM (Object-Relational Mapping) for interacting with the chosen database.