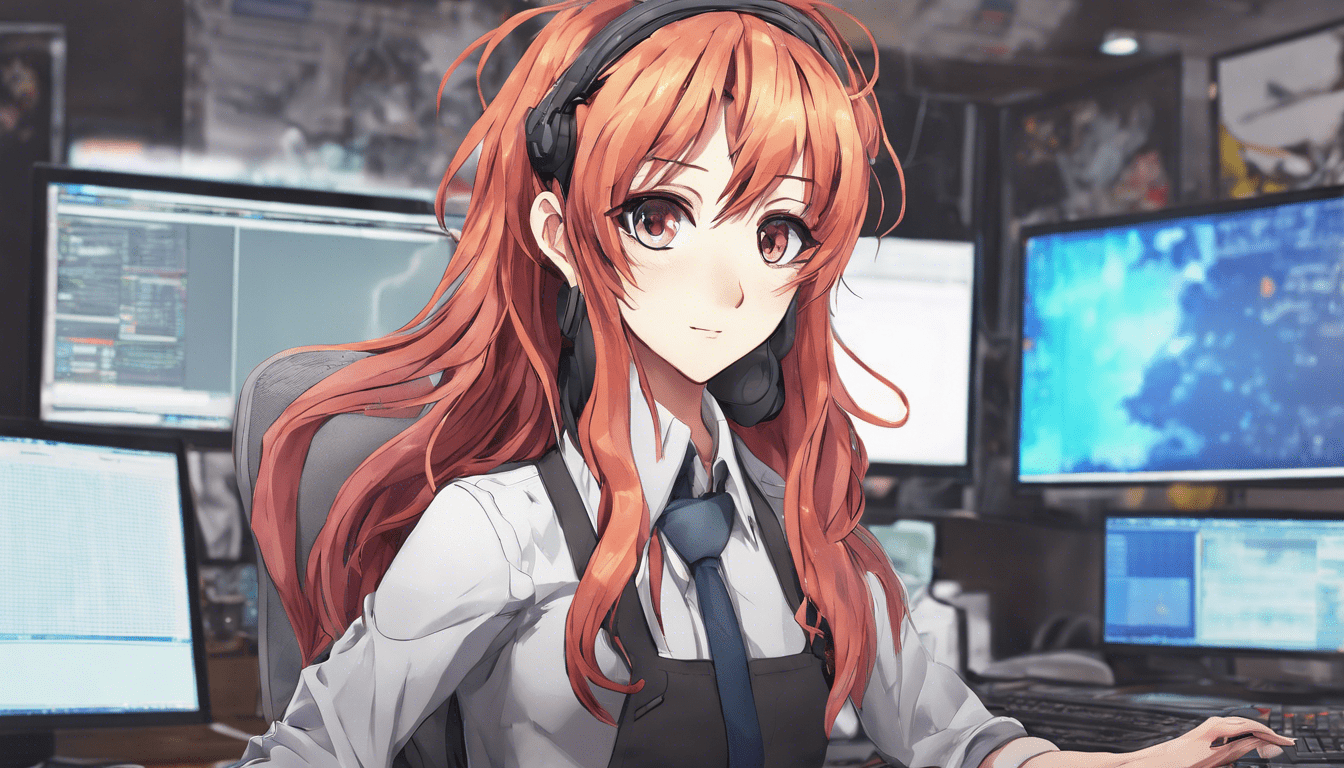
Python and Networking: Sockets and Communication
Python is a versatile programming language that can be used for various purposes, including networking. In this article, we will delve into the key concepts related to Python and networking, specifically focusing on sockets and communication. Understanding these concepts is important for building networked applications and servers using Python.
1. Sockets
Sockets are the endpoints of a network connection that enable communication between two entities on a network. In Python, the socket
module provides a low-level interface for network communication.
To create a socket, you need to specify the address family (such as IPv4 or IPv6) and the socket type (such as TCP or UDP). Here’s an example of creating a TCP/IP socket:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
You can then use this socket to establish connections, send and receive data.
2. IP Addresses and Ports
In networking, an IP address is a unique numerical identifier assigned to each device connected to a network. It enables communication between devices over the Internet. An IP address consists of four sets of numbers separated by periods (e.g., 192.168.0.1).
Ports, on the other hand, are used to identify different services running on a device. They allow multiple applications to run on the same device without interfering with each other. Ports are identified by numbers ranging from 0 to 65535.
3. Communication Using Sockets
Once you have created a socket, you can use it to establish connections, send data, and receive data.
To establish a connection using a TCP/IP socket, you need to specify the IP address and port of the target device. Here’s an example:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) # Connect the socket to the target address server_address = ('localhost', 1234) sock.connect(server_address)
To send data over a TCP/IP socket, you can use the send()
method:
message = "Hello, server!" sock.send(message.encode())
To receive data from a TCP/IP socket, you can use the recv()
method:
data = sock.recv(1024) print(data.decode())
Step-by-Step Guide: Implementing Sockets and Communication
Step 1: Create a TCP/IP Socket
Import the socket
module and create a TCP/IP socket:
import socket # Create a TCP/IP socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Step 2: Establish a Connection
Specify the IP address and port of the target device, then connect the socket:
# Connect the socket to the target address server_address = ('localhost', 1234) sock.connect(server_address)
Step 3: Send and Receive Data
Send data over the socket using the send()
method, and receive data using the recv()
method:
# Send data message = "Hello, server!" sock.send(message.encode()) # Receive data data = sock.recv(1024) print(data.decode())
Common Pitfalls and Troubleshooting Tips
When working with sockets and networking in Python, there are a few common mistakes and issues that beginners may encounter:
- Double-check the IP address and port of the target device to ensure they’re correct.
- Make sure that the firewall settings allow the connection through the specified port.
- Ensure that you encode your messages before sending them and decode received messages properly based on the encoding used.
By being aware of these pitfalls and following best practices, you can avoid many common issues related to socket programming in Python.
Further Learning Resources
If you want to dive deeper into networking with Python, here are some recommended resources:
- “Python Network Programming” by Dr. M. O. Faruque Sarker and Sam Washington, “Foundations of Python Network Programming” by Brandon Rhodes and John Goerzen
- “Python Networking for Network Engineers (Python Cisco)” on Udemy, “Python Network Programming – Part 1: Build 7 Python Apps” on Pluralsight
- “Python Networking Tutorial” on Guru99, “Python Socket Programming Tutorial” on Real Python
By exploring these resources, you can deepen your understanding of networking in Python and further enhance your skills.
Understanding sockets and communication is important for building networked applications and servers using Python. By mastering these concepts, you can create powerful networked programs that facilitate data exchange between devices. Remember to troubleshoot common issues and continuously learn and practice to strengthen your networking skills in Python. Happy coding!
Great overview of sockets and networking in Python! One thing I’d like to add is the importance of handling exceptions when working with sockets. Network communication can be unpredictable, and your code should be prepared to handle situations like timeouts, connection errors, or unexpected data. Using try-except blocks can help ensure that your application can gracefully handle these issues and provide a more robust networking solution.