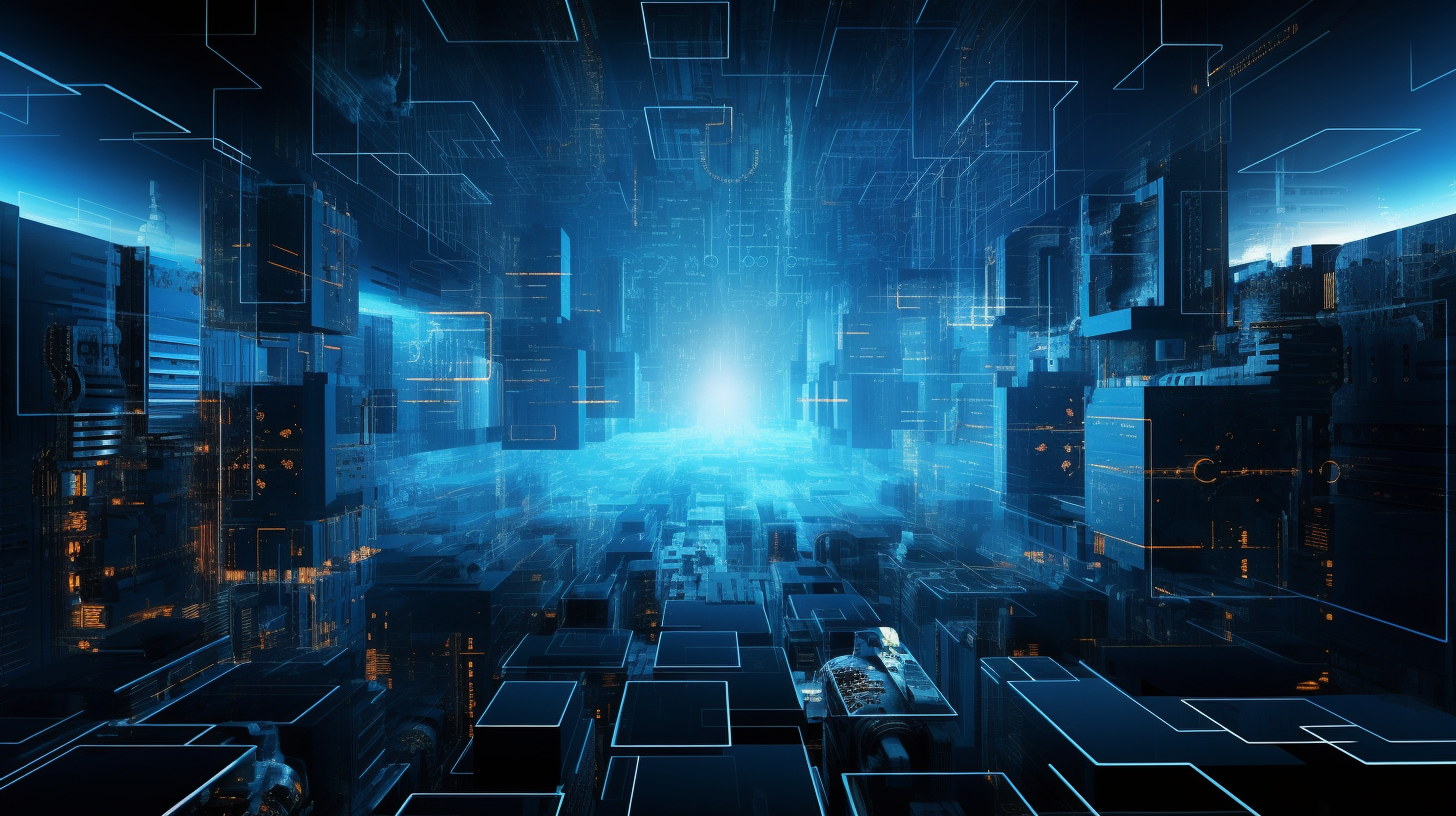
Python for Video Processing and Analysis
If you are a Python developer interested in video processing and analysis, you’ve come to the right place. Python is a powerful language that can be used for a wide range of applications, including video processing and analysis. In this tutorial, we will explore how to use Python and specifically the OpenCV library to process and analyze videos.
What is Video Processing?
Video processing involves manipulating and modifying video data. This can include tasks such as cropping, resizing, rotating, and changing the color of videos. Videos can also be analyzed for various purposes, such as object detection, tracking, and motion estimation.
Introduction to OpenCV
OpenCV is an open-source computer vision library that provides a wide range of tools and functions for video processing and analysis. It can be used with multiple programming languages, including Python.
To get started, you’ll need to install the OpenCV library. You can do this using pip by running the following command:
pip install opencv-python
Reading and Playing Videos
Let’s start by learning how to read and play videos using OpenCV.
import cv2 # Open the video file video = cv2.VideoCapture('path_to_video_file') # Check if the video file is opened successfully if not video.isOpened(): raise Exception('Error opening video file') # Read the video frame by frame while video.isOpened(): ret, frame = video.read() # Check if there is a valid frame if ret: # Display the frame in a window named 'Video' cv2.imshow('Video', frame) # Wait for the 'q' key to be pressed to stop playing the video if cv2.waitKey(1) & 0xFF == ord('q'): break else: # Break the loop if there are no more frames break # Release the video file and close the window video.release() cv2.destroyAllWindows()
In the code above, we first open the video file using the cv2.VideoCapture
function. We then check if the video file was opened successfully using the isOpened
method. We read the video frame by frame using the read
method. If there is a valid frame, we display it in a window named ‘Video’ using the imshow
function. The video playback stops when the ‘q’ key is pressed. Finally, we release the video file and close the window.
Modifying Videos
Now let’s learn how to modify videos using OpenCV. We will cover a few common video processing tasks.
Cropping Videos
Cropping involves selecting a region of interest (ROI) in a video and extracting it as a separate video or image sequence.
import cv2 # Open the video file video = cv2.VideoCapture('path_to_video_file') # Check if the video file is opened successfully if not video.isOpened(): raise Exception('Error opening video file') # Define the dimensions of the cropped video x, y, width, height = 100, 100, 300, 300 # Create a VideoWriter object to save the cropped video output = cv2.VideoWriter('path_to_output_video_file', cv2.VideoWriter_fourcc(*'mp4v'), 30.0, (width, height)) # Read the video frame by frame while video.isOpened(): ret, frame = video.read() # Check if there is a valid frame if ret: # Crop the frame using the defined dimensions cropped_frame = frame[y:y+height, x:x+width] # Display the cropped frame in a window named 'Video' cv2.imshow('Video', cropped_frame) # Save the cropped frame to the output video file output.write(cropped_frame) # Wait for the 'q' key to be pressed to stop cropping the video if cv2.waitKey(1) & 0xFF == ord('q'): break else: # Break the loop if there are no more frames break # Release the video file, the output video file, and close the window video.release() output.release() cv2.destroyAllWindows()
In the code above, we first open the video file and check if it was opened successfully. We define the dimensions of the cropped video by specifying the coordinates of the top-left corner and the width and height. We create a VideoWriter
object to save the cropped video. We read the video frame by frame, crop each frame using the defined dimensions, and display the cropped frame in a window named ‘Video’. We also save the cropped frame to the output video file using the write
method of the VideoWriter
object. The cropping process stops when the ‘q’ key is pressed. Finally, we release the video files and close the window.
Applying Filters to Videos
OpenCV provides various filters that can be applied to videos, such as Gaussian blur, Sobel edge detection, and color transformations.
import cv2 # Open the video file video = cv2.VideoCapture('path_to_video_file') # Check if the video file is opened successfully if not video.isOpened(): raise Exception('Error opening video file') # Read the video frame by frame while video.isOpened(): ret, frame = video.read() # Check if there is a valid frame if ret: # Apply Gaussian blur to the frame blurred_frame = cv2.GaussianBlur(frame, (5, 5), 0) # Apply Sobel edge detection to the frame gray_frame = cv2.cvtColor(blurred_frame, cv2.COLOR_BGR2GRAY) edges_frame = cv2.Sobel(gray_frame, cv2.CV_64F, 1, 1, ksize=3) # Display the filtered frame in a window named 'Video' cv2.imshow('Video', edges_frame) # Wait for the 'q' key to be pressed to stop applying filters to the video if cv2.waitKey(1) & 0xFF == ord('q'): break else: # Break the loop if there are no more frames break # Release the video file and close the window video.release() cv2.destroyAllWindows()
In the code above, we first open the video file and check if it was opened successfully. We read the video frame by frame and apply a Gaussian blur filter to each frame using the GaussianBlur
function. We then convert the blurred frame to grayscale using the cvtColor
function and apply Sobel edge detection using the Sobel
function. We display the filtered frame in a window named ‘Video’. The filtering process stops when the ‘q’ key is pressed. Finally, we release the video file and close the window.
Video Analysis
OpenCV provides powerful tools for video analysis, such as object detection and tracking, motion estimation, and optical flow.
Object Detection and Tracking
Object detection and tracking involve identifying and tracking objects within a video.
import cv2 # Load the object detection model net = cv2.dnn.readNetFromCaffe('path_to_prototxt_file', 'path_to_caffemodel_file') # Open the video file video = cv2.VideoCapture('path_to_video_file') # Check if the video file is opened successfully if not video.isOpened(): raise Exception('Error opening video file') # Read the video frame by frame while video.isOpened(): ret, frame = video.read() # Check if there is a valid frame if ret: # Perform object detection blob = cv2.dnn.blobFromImage(frame, 1.0, (300, 300), (104.0, 177.0, 123.0)) net.setInput(blob) detections = net.forward() # Loop over the detections and draw bounding boxes around the objects for i in range(detections.shape[2]): confidence = detections[0, 0, i, 2] if confidence > 0.5: box = detections[0, 0, i, 3:7] * np.array([width, height, width, height]) (x1, y1, x2, y2) = box.astype("int") cv2.rectangle(frame, (x1, y1), (x2, y2), (0, 255, 0), 2) # Display the frame with bounding boxes in a window named 'Video' cv2.imshow('Video', frame) # Wait for the 'q' key to be pressed to stop object detection and tracking if cv2.waitKey(1) & 0xFF == ord('q'): break else: # Break the loop if there are no more frames break # Release the video file and close the window video.release() cv2.destroyAllWindows()
In the code above, we first load the object detection model using the readNetFromCaffe
function. We then open the video file and check if it was opened successfully. We read the video frame by frame and perform object detection using the blobFromImage
function and the forward
method of the network. We loop over the detections and draw bounding boxes around the objects using the rectangle
function. We display the frame with the bounding boxes in a window named ‘Video’. The object detection and tracking process stops when the ‘q’ key is pressed. Finally, we release the video file and close the window.
Motion Estimation and Optical Flow
Motion estimation and optical flow involve detecting and tracking the movement of objects within a video.
import cv2 # Open the video file video = cv2.VideoCapture('path_to_video_file') # Check if the video file is opened successfully if not video.isOpened(): raise Exception('Error opening video file') # Read the first frame of the video ret, prev_frame = video.read() # Convert the first frame to grayscale prev_gray = cv2.cvtColor(prev_frame, cv2.COLOR_BGR2GRAY) # Create a mask for visualization purposes flow_mask = np.zeros_like(prev_frame) # Read the video frame by frame while video.isOpened(): ret, frame = video.read() # Check if there is a valid frame if ret: # Convert the current frame to grayscale curr_gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # Calculate the dense optical flow between the previous and current frames flow = cv2.calcOpticalFlowFarneback(prev_gray, curr_gray, None, 0.5, 3, 15, 3, 5, 1.2, 0) # Convert the optical flow to polar coordinates (magnitude and angle) magnitude, angle = cv2.cartToPolar(flow[..., 0], flow[..., 1]) # Set the hue of the flow mask based on the angle of the optical flow flow_mask[..., 0] = angle * 180 / np.pi / 2 # Set the value of the flow mask based on the magnitude of the optical flow flow_mask[..., 2] = cv2.normalize(magnitude, None, 0, 255, cv2.NORM_MINMAX) # Convert the flow mask to BGR for visualization purposes flow_bgr = cv2.cvtColor(flow_mask, cv2.COLOR_HSV2BGR) # Display the frame with the optical flow visualization in a window named 'Video' cv2.imshow('Video', flow_bgr) # Wait for the 'q' key to be pressed to stop motion estimation and optical flow if cv2.waitKey(1) & 0xFF == ord('q'): break # Update the previous frame and grayscale image prev_frame = frame prev_gray = curr_gray else: # Break the loop if there are no more frames break # Release the video file and close the window video.release() cv2.destroyAllWindows()
In the code above, we first open the video file and check if it was opened successfully. We read the first frame of the video and convert it to grayscale. We create a mask for visualization purposes and initialize it with zeros. We then read the video frame by frame and convert each frame to grayscale. We calculate the dense optical flow between the previous and current frames using the calcOpticalFlowFarneback
function. We convert the optical flow to polar coordinates (magnitude and angle) using the cartToPolar
function. We set the hue of the flow mask based on the angle of the optical flow and the value based on the magnitude. We convert the flow mask to BGR for visualization purposes using the cvtColor
function. We display the frame with the optical flow visualization in a window named ‘Video’. The motion estimation and optical flow process stops when the ‘q’ key is pressed. Finally, we release the video file and close the window.
In this tutorial, we have explored how to use Python and the OpenCV library to process and analyze videos. We have learned how to read and play videos, modify videos by cropping and applying filters, and analyze videos by performing object detection and tracking, motion estimation, and optical flow. Video processing and analysis are important tasks in computer vision and Python with OpenCV provides a powerful and flexible platform for performing these tasks.
Have fun exploring the world of video processing and analysis with Python!
Great tutorial on using Python and OpenCV for video processing and analysis. I would also like to add that for those who are interested in working with real-time video streams, OpenCV provides the VideoCapture function that can be used to capture video from a camera or a video file in real-time. Additionally, for more advanced applications, one can explore the use of machine learning and deep learning techniques for more accurate object detection and tracking. These can be integrated with OpenCV to create powerful computer vision applications.