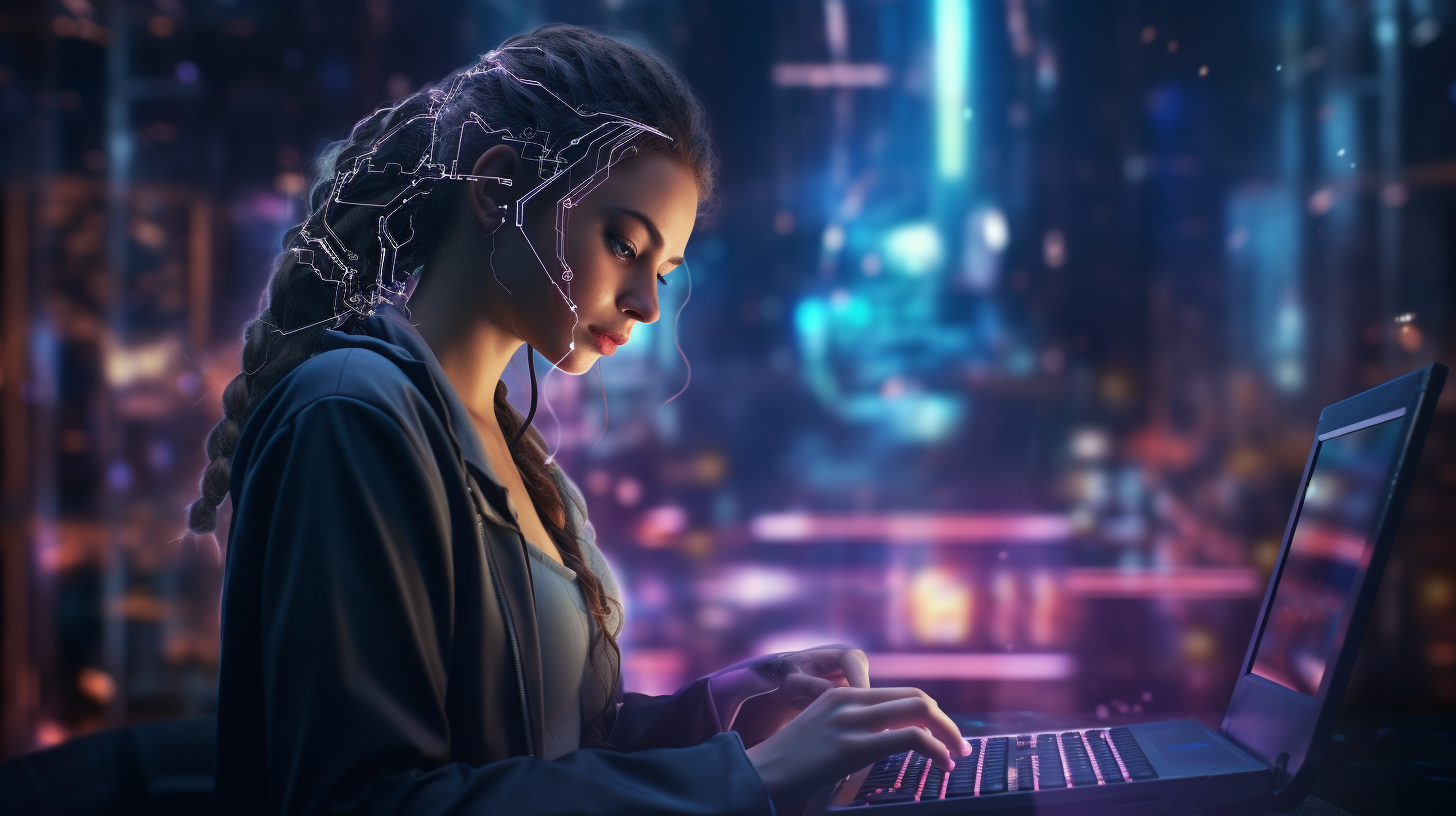
SQL and Functions for Complex Data Analysis
SQL is a powerful language for managing and analyzing data in relational databases. Functions in SQL are predefined operations that can be used to manipulate data, perform calculations, and carry out complex data analysis. In this article, we will discuss some of the most commonly used functions in SQL and how they can be used for complex data analysis.
Aggregate Functions
Aggregate functions are used to perform a calculation on a set of values and return a single value. Some of the most common aggregate functions include:
- Returns the number of rows that match a specified criteria.
- Returns the sum of a numeric column.
- Returns the average value of a numeric column.
- Returns the minimum value of a column.
- Returns the maximum value of a column.
Here is an example of how to use aggregate functions in SQL:
SELECT COUNT(*) AS TotalOrders, SUM(OrderAmount) AS TotalRevenue, AVG(OrderAmount) AS AverageOrderAmount, MIN(OrderAmount) AS SmallestOrderAmount, MAX(OrderAmount) AS LargestOrderAmount FROM Orders WHERE OrderDate >= '2021-01-01' AND OrderDate <= '2021-12-31';
This query will return the total number of orders, total revenue, average order amount, smallest order amount, and largest order amount for orders placed in the year 2021.
String Functions
String functions are used to manipulate string data. Some of the most common string functions include:
- Concatenates two or more strings together.
- Converts a string to uppercase.
- Converts a string to lowercase.
- Removes leading and trailing spaces from a string.
- Extracts a substring from a string.
Here is an example of how to use string functions in SQL:
SELECT CONCAT(FirstName, ' ', LastName) AS FullName, UPPER(FirstName) AS FirstNameUpper, LOWER(LastName) AS LastNameLower, TRIM(Title) AS TrimmedTitle, SUBSTRING(Email, 1, CHARINDEX('@', Email)-1) AS EmailUsername FROM Customers;
This query will return the full name, first name in uppercase, last name in lowercase, trimmed title, and email username for each customer in the Customers table.
Date and Time Functions
Date and time functions are used to manipulate date and time values. Some of the most common date and time functions include:
- Returns the current date and time.
- Returns the current date.
- Returns the current time.
- Adds a specified interval to a date.
- Subtracts a specified interval from a date.
Here is an example of how to use date and time functions in SQL:
SELECT NOW() AS CurrentDateTime, CURDATE() AS CurrentDate, CURTIME() AS CurrentTime, DATE_ADD(OrderDate, INTERVAL 7 DAY) AS OrderDatePlus7Days, DATE_SUB(OrderDate, INTERVAL 1 MONTH) AS OrderDateMinus1Month FROM Orders WHERE OrderStatus = 'Shipped';
This query will return the current date and time, the current date, the current time, the order date plus seven days, and the order date minus one month for all shipped orders in the Orders table.
Functions can greatly enhance the capabilities of SQL queries and make complex data analysis much easier. By understanding and utilizing aggregate, string, and date and time functions, analysts can perform sophisticated calculations and manipulations on their data with ease.