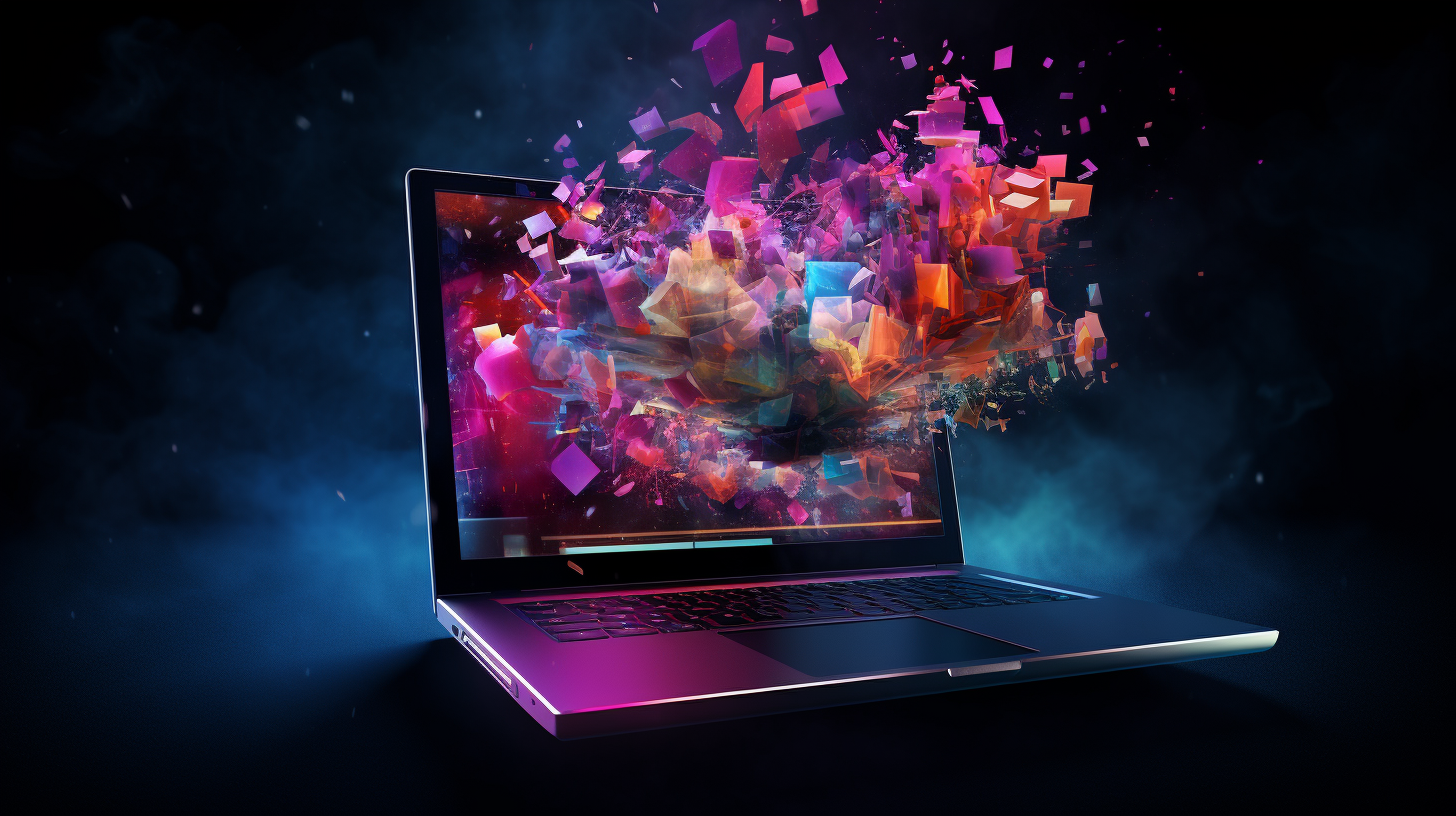
Swift and AVFoundation
AVFoundation is a powerful framework in Swift that allows developers to work with audio and video media in their applications. It provides a set of APIs for playing, recording, editing, and composing media assets. In this article, we will explore the basics of AVFoundation and how to use it in your Swift projects.
AVFoundation is made up of several key classes that enable developers to work with media assets. These include:
- Represents a media asset, such as an audio or video file.
- Enables you to play audio and video assets.
- Manages the capture of audio and video from the device’s camera and microphone.
- Enables you to record audio from the device’s microphone.
Let’s start with an example of how to play an audio file using AVPlayer. First, you need to import the AVFoundation framework in your Swift file:
import AVFoundation
Next, create an instance of AVPlayer and initialize it with the URL of the audio file you want to play:
let audioUrl = URL(fileURLWithPath: Bundle.main.path(forResource: "audioFile", ofType: "mp3")!) let player = AVPlayer(url: audioUrl)
Once you have created an instance of AVPlayer, you can control the playback of the audio file using its methods:
player.play() player.pause()
Now let’s look at an example of how to record audio using AVCaptureSession. First, create an instance of AVCaptureSession and configure it for audio recording:
let captureSession = AVCaptureSession() captureSession.sessionPreset = .high let audioDevice = AVCaptureDevice.default(for: .audio) let audioInput = try! AVCaptureDeviceInput(device: audioDevice!) captureSession.addInput(audioInput)
Next, create an instance of AVAudioRecorder and configure it to record the audio from the capture session:
let audioOutputUrl = URL(fileURLWithPath: NSTemporaryDirectory().appending("audioRecording.m4a")) let audioRecorder = try! AVAudioRecorder(url: audioOutputUrl, settings: [AVFormatIDKey: kAudioFormatMPEG4AAC, AVSampleRateKey: 44100, AVNumberOfChannelsKey: 1]) audioRecorder.prepareToRecord() audioRecorder.record()
To stop the recording, use the stop()
method on the AVAudioRecorder instance:
audioRecorder.stop()
To wrap it up, AVFoundation is a versatile framework that enables you to work with audio and video media in Swift. With its powerful set of APIs, you can easily play, record, edit, and compose media assets in your applications. By following the examples provided in this article, you should now have a good understanding of how to get started with AVFoundation in Swift.