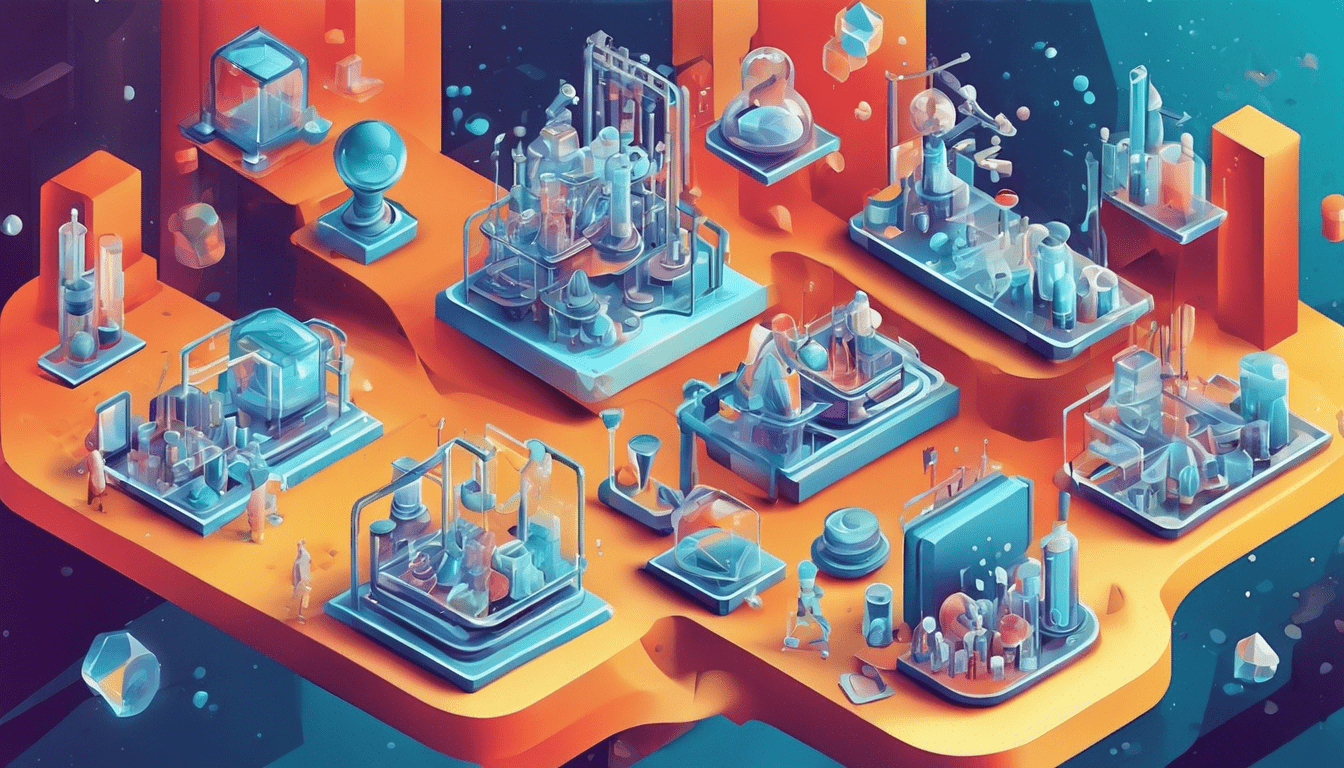
Swift and Bluetooth Integration
Bluetooth technology has become synonymous with wireless connectivity, allowing devices to communicate with each other over short distances. Integrating Bluetooth functionality into your Swift apps can enable a wealth of features, from data sharing to controlling external hardware. In this article, we’ll explore the fundamentals of Bluetooth integration using Swift and the CoreBluetooth framework provided by Apple.
Understanding CoreBluetooth
CoreBluetooth is Apple’s framework that provides classes and protocols for communicating with Bluetooth Low Energy (BLE) devices. BLE is designed for low-power consumption and is perfect for battery-operated devices like wearables and sensors.
To use CoreBluetooth in your Swift project, you must first import the framework:
import CoreBluetooth
Central and Peripheral Roles
In Bluetooth communication, there are two primary roles: Central and Peripheral. A Central device scans for, connects to, and exchanges data with Peripherals. Peripherals advertise data and wait to be connected by Centrals.
Your Swift app can function as either role; the choice depends on its purpose. For example, if your app needs to gather data from sensors, it’ll act as a Central. If it’s broadcasting data, it would function as a Peripheral.
Scanning for Devices
As a Central, before you can connect to a Peripheral, you need to discover it by scanning for devices advertising their services:
let centralManager = CBCentralManager(delegate: self, queue: nil) func centralManagerDidUpdateState(_ central: CBCentralManager) { if central.state == .poweredOn { centralManager.scanForPeripherals(withServices: nil, options: nil) } else { print("Bluetooth is not powered on") } }
Connecting to Peripherals
Once you discover a Peripheral you want to connect to, the next step is establishing a connection:
func centralManager(_ central: CBCentralManager, didDiscover peripheral: CBPeripheral, advertisementData: [String : Any], rssi RSSI: NSNumber) { print("Discovered (String(describing: peripheral.name))") // Stop scanning central.stopScan() // Connect to the discovered peripheral central.connect(peripheral, options: nil) }
Exchanging Data
A successful connection allows the Central to interact with the Peripheral’s services and characteristics, which can be thought of like variables that hold data. To read or write data:
func peripheral(_ peripheral: CBPeripheral, didDiscoverServices error: Error?) { guard error == nil else { print("Error discovering services: (error!.localizedDescription)") return } for service in peripheral.services ?? [] { // Discover characteristics of each service peripheral.discoverCharacteristics(nil, for: service) } } func peripheral(_ peripheral: CBPeripheral, didDiscoverCharacteristicsFor service: CBService, error: Error?) { guard error == nil else { print("Error discovering characteristics: (error!.localizedDescription)") return } if let characteristics = service.characteristics { for characteristic in characteristics { // Read value of characteristic peripheral.readValue(for: characteristic) // Write new value to characteristic (if it is writable) if characteristic.properties.contains(.write) { let newValue: Data = ... peripheral.writeValue(newValue, for: characteristic, type: .withResponse) } } } }
Integrating Bluetooth in your Swift apps opens up possibilities for interacting with various BLE devices. By leveraging CoreBluetooth’s capabilities as explained above, you can control peripherals or gather data from sensors wirelessly. With practice, you can build more complex and robust Bluetooth-enabled applications.
Remember that that’s just the tip of the iceberg; deeper dives into CoreBluetooth will expose advanced features like state preservation and restoration, peer-to-peer connectivity, and more. Happy coding!